Question
I would like someone to help me with coding a function that takes a file and analyze it as the following Given the name of
I would like someone to help me with coding a function that takes a file and analyze it as the following
Given the name of a file, a list of positive words (all in lowercase), and a list of negative words (all in lowercase) return the positivity score of the contents of this file.
How to calculate positivity score:
For every word that is pos itive in the file (based on the words in the list of positive words) add 1 to the score. For every negative word (based on the list of negative words), subtract 1 from the score. Any neutral words (those that do not appear in either list) do not affect the score in any way.
You should use get_words function in the code and normalize function
I have a problem in making the function analyze_file to iterate through list of words
Files list
- happy_lyrics.txt
(Because I'm happy)
Clap along if you feel like a room without a roof
(Because I'm happy)
Clap along if you feel like happiness is the truth
(Because I'm happy)
Clap along if you know what happiness is to you
(Because I'm happy)
Clap along if you feel like that's what you wanna do
- positive_words.txt
happy
joy
fun
- negative_words.txt
sad
grumpy
annoyed
def normalize(s: str) -> str:
"""
Given a string, return a copy of that string with
all alphabetical characters turned lowercase and
all special characters removed and replaced with a space.
>>> normalize("Hey! How are you? I'm fine. ;D")
'hey how are you i m fine d'
"""
n = ""
for c in s:
if c.isalpha():
n = n + c
else:
n = n + " "
return n
def get_words(filename: str) -> str:
"""
Given the name of a file which contains many words
(one word per line), return a list of all these words.
The file may have comments and a blank space at the beginning
of the file, which should be ignored. All comment lines start with
a semicolon ';'.
"""
words = []
with open(filename, 'r') as file:
for line in file.readlines():
if line[0] == ';' or line.strip() == '':
file.readline()
else:
words.append(line.strip().lower())
return words
def analyze_word(word: str, pos_words: List[str], neg_words: List[str]) -> int:
"""
Given a word, a list of positive words (all in lowercase), and a list of negative words (all in lowercase), return whether or not the word is positive, negative or neutral.
For a positive word, return 1.
For a negative word, return -1.
For a neutral word (one that does not appear in either the
negative words list nor the positive words list), return 0.
>>> ("happy", ['happy', 'love'], ['sad', 'angry'])
1
>>> ("angry", ['happy', 'love', 'joy'], ['sad', 'angry', 'bad'])
-1
>>> ("LOVE", ['happy', 'love', 'joy'], ['sad', 'angry', 'bad'])
1
>>> ("sAd", ['happy', 'love', 'joy'], ['sad'])
-1
>>> ("okay", ['happy', 'love', 'joy'], ['sad', 'angry'])
0
"""
word = word.strip().lower()
if word in pos_words:
return 1
elif word in neg_words:
return -1
else:
return 0
def analyze_file(filename: str, pos_words: List[str], neg_words: List[str]) -> int:
"""
Given the name of a file, a list of positive words (all in lowercase),
and a list of negative words (all in lowercase), return some interesting
data about the file in a tuple, with the first item in the tuple being
the positivity score of the contents of this file, and the rest of the items
being whatever else you end up analyzing. (Helper functions are highly
encouraged here.)
How to calculate positivity score:
For every word that is pos itive in the file (based on the words
in the list of positive words) add 1
to the score. For every negative word (based on the list
of negative words), subtract 1 from the score. Any neutral
words (those that do not appear in either list) do
not affect the score in any way.
"""
Pass
if __name__ == "__main__":
positives = "positive_words.txt"
negatives = "negative_words.txt"
s = input("Enter a filename: ")
pos_words = get_words(positives)
neg_words = get_words(negatives)
data = analyze_file(s, pos_words, neg_words)
if data[0] < 0:
print("Overall feeling: :(")
elif data[0] > 0:
print("Overall feeling: :)")
else:
print("Overall feeling: :|")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
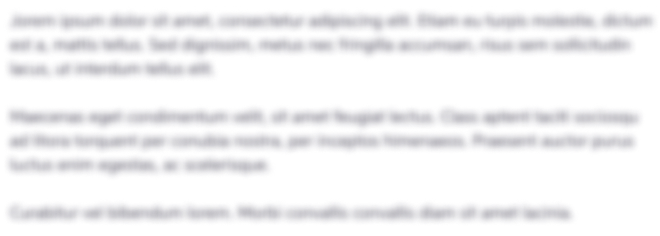
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started