Question
I would like the working file itself for this java code and an explanation of how to put this code in a java complier to
I would like the working file itself for this java code and an explanation of how to put this code in a java complier to get it working. Please and thank you.
ATM_GUI_InterfaceBuilder.java
import javax.swing.*; import java.awt.event.*;
@SuppressWarnings("serial") public class ATM_GUI_InterfaceBuilder extends JFrame {
private static int accountNumber; private static Account savingsAccount = new Savings(accountNumber); private static Account checkingAccount = new Checking(accountNumber);
private static JButton button1, button2, button3, button4; private static JTextField inputFieldValue; private static JRadioButton checking, savings; private static JPanel atmPanel;
ActionEvent transaction;
public static void main(String[] args) { new ATM_GUI_InterfaceBuilder();
}
public ATM_GUI_InterfaceBuilder() {
atmPanel = createNewPanel(); createButtonsAndFields(); buttonActonOnClick(atmPanel); createJRadioButtonGroup_SetDefaultSelection(atmPanel); createTextField(atmPanel); displayPanel(atmPanel);
}
private JPanel createNewPanel() {
JPanel newPanel = new JPanel(); this.setSize(400, 100); this.setLocationRelativeTo(null); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setTitle("ATM Machine");
return newPanel; }
private void createTextField(JPanel thePanel) {
thePanel.add(inputFieldValue); }
private void createJRadioButtonGroup_SetDefaultSelection(JPanel thePanel) {
ButtonGroup groupAccountType = new ButtonGroup(); groupAccountType.add(checking); groupAccountType.add(savings);
thePanel.add(checking); thePanel.add(savings);
checking.setSelected(true); }
private void createButtonsAndFields() {
checking = new JRadioButton("Checking"); savings = new JRadioButton("Savings"); inputFieldValue = new JTextField("", 10); button1 = new JButton(ButtonToTransactions.WITHDRAW); button2 = new JButton(ButtonToTransactions.DEPOSIT); button3 = new JButton(ButtonToTransactions.TRANSFER_TO); button4 = new JButton(ButtonToTransactions.BALANCE); }
private void buttonActonOnClick(JPanel thePanel) {
ActionListener withdrawal = new TransactionButton(thePanel, inputFieldValue, savingsAccount, checkingAccount, ButtonToTransactions.WITHDRAW, checking, savings, button1);
button1.addActionListener(withdrawal);
ActionListener deposit = new TransactionButton(thePanel,
inputFieldValue, savingsAccount, checkingAccount, ButtonToTransactions.DEPOSIT, checking, savings, button2); button2.addActionListener(deposit);
ActionListener transferTo = new TransactionButton(thePanel, inputFieldValue, savingsAccount, checkingAccount, ButtonToTransactions.TRANSFER_TO, checking, savings, button3); button3.addActionListener(transferTo);
ActionListener balance = new TransactionButton(thePanel, inputFieldValue, savingsAccount, checkingAccount, ButtonToTransactions.BALANCE, checking, savings, button4); button4.addActionListener(balance); }
private void displayPanel(JPanel thePanel) {
this.add(thePanel); this.setVisible(true); }
/* * Private Classes extends ButtonType Class. Used to execute back-end * commands when a button is pushed */
/* * savingsAccount checkingAccount */
private class TransactionButton extends ButtonTransactionType {
private JRadioButton rButton1; private JRadioButton rButton2; private JTextField textField; private Account account1; private Account account2; private String transactionType;
TransactionButton(JPanel thePanel, JTextField textField, Account account2, Account account1, String transactionType, JRadioButton rButton1, JRadioButton rButton2, JButton button) {
this.account1 = account1; this.account2 = account2; this.rButton1 = rButton1; this.rButton2 = rButton2; this.textField = textField; this.transactionType = transactionType;
thePanel.add(button);
}
@Override public void actionPerformed(ActionEvent transaction) {
if (rButton2.isSelected()) {
excuteTransactionPanel(textField, account2, account1, transactionType);
} else {
if (rButton1.isSelected()) {
excuteTransactionPanel(textField, account1, account2, transactionType);
}
}
}
}
}
Account.java
public interface Account {
public double deposit(Double amount) throws NumberFormatException;
public double withdrawal(double amount) throws InsufficientFunds;
public double getBalance();
public int getAccountNumber();
public double transferTo(double amount, Account account) throws InsufficientFunds;
public double OVERDRAFT_FEE();
public double SERVICE_FEES();
}
Savings.java
public class Savings extends Transactions {
public Savings(int accountNumber) { super(accountNumber);
}
}
Checking.java
public class Checking extends Transactions {
public Checking(int accountNumber) { super(accountNumber);
}
}
ButtonToTransactions.java
import java.awt.event.ActionListener;
import javax.swing.JTextField;
public interface ButtonToTransactions extends ActionListener {
public static final String WITHDRAW = "Withdraw"; public static final String DEPOSIT = "Deposit"; public static final String TRANSFER_TO = "Transfer to"; public static final String BALANCE = "Balance";
public void excuteTransactionPanel(JTextField textField, Account account1, Account account2, String transaction);
}
ButtonTransactionType.java
import java.awt.Component; import java.text.NumberFormat;
import javax.swing.JOptionPane; import javax.swing.JTextField;
public abstract class ButtonTransactionType implements ButtonToTransactions {
private Component ATM_GUI_InterfaceBuilder;
private Double amount; private Double transactionAmount; private int buttonClicked;
private static final int ZERO = 0; private static final int ENOUGH_TO_CHARGE = 4;
private void displayTransactionResult(Account account) {
String dollarFormat = NumberFormat.getCurrencyInstance()
.format(account.getBalance());
JOptionPane.showMessageDialog(ATM_GUI_InterfaceBuilder, dollarFormat, "Balance in Account # " + account.getAccountNumber(), JOptionPane.INFORMATION_MESSAGE);
}
private void checkForOverdraftPanel(Account account) {
account.SERVICE_FEES(); buttonClicked = ZERO;
if (account.getBalance() < ZERO) { account.OVERDRAFT_FEE();
JOptionPane.showConfirmDialog(null, "An Over Draft fee has been assessed on your Account", "Overdraft FEE: $35.00 ", JOptionPane.CLOSED_OPTION); }
}
private void feeAssessmentPanel(Account account1, Account account2) {
if (buttonClicked == ENOUGH_TO_CHARGE) {
JOptionPane.showConfirmDialog(null, "A service fee has been assessed on your Account", "Service FEE: $1.50 ", JOptionPane.CLOSED_OPTION);
if (account1.getBalance() >= account2.getBalance()) {
checkForOverdraftPanel(account1);
} else {
checkForOverdraftPanel(account2);
}
} }
private void accountTransaction(JTextField textField, Account account1, Account account2, String TRANSACTIONS) throws NumberFormatException, InsufficientFunds {
switch (TRANSACTIONS) {
case BALANCE:
account1.getBalance(); break;
case TRANSFER_TO:
transactionAmount = Double.valueOf(textField.getText()); amount = transactionAmount; account1.transferTo(amount, account2); break;
case DEPOSIT:
transactionAmount = Double.valueOf(textField.getText()); amount = transactionAmount; account1.deposit(amount); break;
case WITHDRAW:
transactionAmount = Double.valueOf(textField.getText()); amount = (transactionAmount % 20 == ZERO) ? transactionAmount : null; account1.withdrawal(amount);
if (amount > ZERO) buttonClicked++; feeAssessmentPanel(account1, account2);
break;
default:
break; } }
public void excuteTransactionPanel(JTextField textField, Account account1, Account account2, String transaction) {
try {
accountTransaction(textField, account1, account2, transaction);
} catch (InsufficientFunds e3) {
JOptionPane.showConfirmDialog(null, "You current have Insufficient Funds for this Transaction", "Insufficient Funds", JOptionPane.CLOSED_OPTION);
} catch (NumberFormatException e1) {
JOptionPane.showConfirmDialog(null, "Please enter a number", "Number Error: Account # " + account1.getAccountNumber(), JOptionPane.CLOSED_OPTION);
} catch (RuntimeException e4) {
JOptionPane.showConfirmDialog(null, "Only amount in increments of $20 are allowed", "$20s Only", JOptionPane.CLOSED_OPTION);
} finally {
displayTransactionResult(account1);
}
}
}
InsufficientFunds.java
@SuppressWarnings("serial") public class InsufficientFunds extends Exception {
public InsufficientFunds() { super(); }
}
Transactions.java
import java.util.Random;
abstract class Transactions implements Account {
private double balance; private int accountNumber; private final double SERVICE_FEES = 1.50; private final double OVERDRAFT = 35.00;
private void setBalance(double balance) { this.balance = balance; }
private void setAccountNumber(int accountNumber) { this.accountNumber = accountNumber; }
public Transactions(int accountNumber) {
Random rand = new Random(); accountNumber = rand.nextInt(9999999);
this.setAccountNumber(accountNumber); // this.setBalance(100);
}
public double deposit(Double amount) throws NumberFormatException {
if (amount == null) {
throw new NumberFormatException();
} else { setBalance(getBalance() + amount);
}
return getBalance(); }
public double withdrawal(double amount) throws InsufficientFunds {
if (amount <= getBalance()) {
setBalance(getBalance() - amount);
} else {
throw new InsufficientFunds();
}
return getBalance(); }
public double transferTo(double amount, Account account) throws InsufficientFunds {
if (amount <= account.getBalance()) {
account.withdrawal(amount); setBalance(getBalance() + amount);
} else {
throw new InsufficientFunds(); }
return getBalance(); }
public double OVERDRAFT_FEE() {
setBalance(getBalance() - OVERDRAFT); return getBalance();
}
public double SERVICE_FEES() {
setBalance(getBalance() - SERVICE_FEES); return getBalance();
}
public double getBalance() {
return balance; }
public int getAccountNumber() { return accountNumber; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
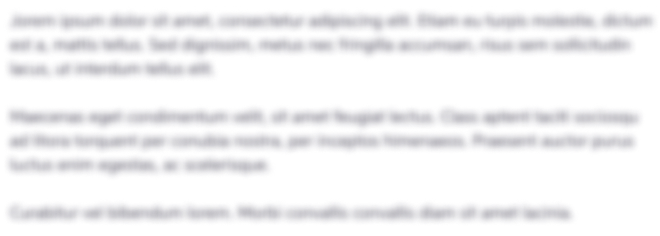
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started