Question
I would really appreciate some help with this programming assignment, I have seen relatively the same problem answered but our instructor added the stipulation that
I would really appreciate some help with this programming assignment, I have seen relatively the same problem answered but our instructor added the stipulation that we are given the main function and it cannot be changed, and so i am extremely confused, thank you for your time! (answer in C++ programming language please)
Tasks
The United States Postal Service encourages companies that send large volumes of mail to use a bar code to encode the zip code that the package should be routed to. Your job is to write software that will drive a barcode generator that your company designed and built. In order to get full credit for this assignment you must write your own functions, you should not be writing any code in the main function, it is given to you in the starter files. The encoding scheme for a five-digit zip code is shown in figure 1. There are full-height frame bars on each side. The five encoded digits are followed by a check digit, which is computed as follows: Add up all digits, and choose the check digit to make the sum a multiple of 10.
The sixth digit of the code is the check digit, which allows us to determine if we have entered a valid zip code. .If you are generating the check digit (correction character), which is not a requirement of this project, you would follow the table below.
For example, the zip code 95014 has a sum of 19, so the check digit is 1 to make a sum equal to 20. Each digit of the zip code, and the check digit, is encoded according to table 1 where 0 denotes a half bar and 1 a full bar. The digit can be computed from the bar code using the column weights 7,4,2,1,0. For example, 01100 is 0 x 7 + 1 x 4 + 1 x 2 + 0 x 1 + 0 x 0 = 6. The only exception is 0, which would yield 11 according to the weight formula. Again, this is required for creating a NEW barcode. You are only supposed to verify existing barcodes.
.
Sample Output
Write a program that asks the user for a bar code and prints the correct zip code. For example the bar code below should output 95014.
||:|:::|:|:||::::::||:|::|:::|||
95014
||:|:::|:|:||::::::||:::||:|::||
95011
||:|:::|:|:||::::::||:::||:|::||\\\\
The postal bar code is invalid.
Hints
Here is the completed main function
int main(int argc, char **argv)
{
//Do not modify the main function
string inputBarCode;
cin >> inputBarCode;
int validZip = convert(inputBarCode);
if (validZip >= 0){
cout
} else{
cout
}
return 0;
}
- In the table above a 1 is equal to a | and a 0 is equal to a colon (:)
- Leverage the string class in the standard library.
- You can use the substring method to split the barcode that the user inputs into your program string.substr
- Split up the problem into different functions to make your code eaiser to understand (Abstraction). For example create a function named string_to_num that converts a string (||:::) to a number (see the example below).
/**
Returns the zip code value for a given bar code string.
@param code the bar code string
@return value of the bar code, -1 if invalid code
*/
int string_to_num(string code)
{
if (code == "||:::") {
return 0;
}
//more code here to get the rest of the digits
}
- In your convert function call your string_to_num function that you created to process each part of the barcode. Note: be sure to check for known invalid codes (whats below and code length != 32, character != | or :).
/**
Converts a barcode sequence into its numeric form.
@param barcode the barcode string
@return numeric form of the barcode, -1 if invalid
*/
int convert(string barcode)
{
const int DIGIT_LENGTH = 5;
//Check if the barcode starts with a |
if (barcode.substr(0, 1) != "|") {
return -1;
}
//Check if we have a string that is long enough to make a barcode
if (barcode.substr(barcode.length() - 1, 1) != "|") {
return -1;
}
//Grab the first digit
int d1 = string_to_num(barcode.substr(1, DIGIT_LENGTH));
//process the rest of the digits
//Calculate the number
return /*the barcode as a number*/;
}
- You are allowed to create more functions if needed. These are just hints!
- Below are a some zip codes that you can test with the correct output
||:|:::|:|:||::::::||||::::|:|:| == 95010
||:|:::|:|:||::::::||:::||:|::|| == 95011
||:|:::|:|:||::::::||::|:|::||:| == 95012
::|:::||:|:::|||:::|:|::||| == The postal bar code is invalid.
- You will need to add #include to the top of your file so you can access the string class
- You will need to calculate the check digit using a function we discussed in class.
- Say our barcode is 95014)
So in our code once we have read in the barcode (using the string_to_num function)
we should be able to verify that our barcode is correct with the following formula.
If the remainder of (9 + 5 + 0 + 1 + 4 + 1) / 10 is 0 (zero) then we have a valid zip code.
- Remember, you are not required to generate a check digit. You are just required to verify that the given barcode and check digit (what is typed into your program by the user) is correct.
- The term Correction Character and Check digit mean the same thing.
What not to do
Do not modify any header file given to you.
What you will be graded on
You will be graded on the following criteria:
- Your program compiles and runs. If your program does not compile, 60% of the grade is in jeopardy. However, depending on the severity of the issue, you can receive some credit.
- Your code logic is correct. Be sure to test your conditionals and all of your endpoints. Code that is not reachable (Dead Code) should not be present.
- You code is well structured and your use of abstraction is good.
- Your use of variables and constants. Well named variables should be used. Constants should be used when warranted. Proper case for constants and camel case for variables should be used.
- Your use of commenting and white space. Each file that is turned in needs a file header. All functions need function headers. Comments should be used to aid in readability (for you, weeks later and for others). White space aids in readability. If you are using an IDE then indentation is handled for you automatically. Sections of code should be broken up using blank lines; i.e., to separate declarations and code, etc..
What to turn in
All the files should be well documented, to include file headers and headers for each function, as well as appropriate inline comments.
Turn in main.cpp. Any other submission will not be graded.
5-Digt ZIP Code (A Fleld) Correcton Charackr Fram Bar 4 DZIP Code 2 3 Bar 4 (weight 1) 0 0 0 Digit Bar 1 (weight 7) Bar 2 (weight 4) 0 Bar 3 (weight 2) Bar 5 (weight 0) 2 0 0 0 0Step by Step Solution
There are 3 Steps involved in it
Step: 1
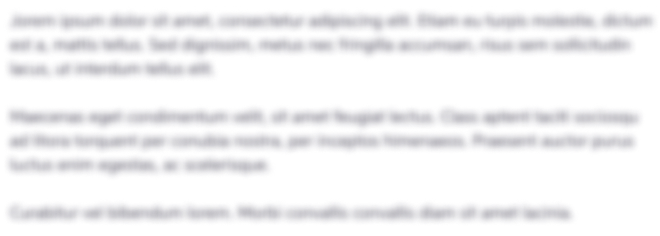
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started