Question
if we have FavoritePage class: public class FavoritePage { private String name; private String url; private int visitCount; public String getName() { return name; }
if we have FavoritePage class:
public class FavoritePage {
private String name;
private String url;
private int visitCount;
public String getName() {
return name;
}
public String getUrl() {
return url;
}
public int getVisitCount() {
return visitCount;
}
public void setName(String name) {
this.name = name;
}
public void setUrl(String url) {
this.url = url;
}
public void setVisitCount(int visitCount) {
this.visitCount = visitCount;
}
public FavoritePage(String name, String url, int visitCount) {
super();
this.name = name;
this.url = url;
this.visitCount = visitCount;
}
}
and singleton Database class that hosts a collection of FavoritePage instances and provides the following methods: - static void load( File f ) throws IOException, ClassNotFoundException load the database (into the singleton variable) from the specified file - void save( File f ) throws IOException save this database to the specified file - int getPageCount() - FavoritePage getPage( int ) return the site at the specified index - List getPages() return the list of sites, wrapped to be unmodifiable (see Collections . unmodifiableList()).
//DataBaseClass
class DataBaseClass{
private static DataBaseClass obj=new DataBaseClass();//Early, instance will be created at load time
private DataBaseClass(){}
private static List
private static File databaseFile;
public static DataBaseClass getObject(){
return obj;
}
//load the database (into the singleton variable) from the specified file
static void load( File f ) throws IOException, ClassNotFoundException{
databaseFile = f;
BufferedReader br = new BufferedReader(new FileReader(f));
String str;
while ((str = br.readLine()) != null) {
int i=0;
String name = null,url = null,count = null;
//assume database file is have page name , url and count in space seperated file
StringTokenizer st = new StringTokenizer(str, " ");
while(st.hasMoreTokens()) {
if(i== 0)
name = st.nextToken(); //make first part as page name
if(i == 1)
url = st.nextToken(); // make second part as url of page
if(i==2)
count = st.nextToken();
}
int visitCount = Integer.parseInt(count);
FavoritePage favPage = new FavoritePage(name, url, visitCount);
pages.add(favPage);
//System.out.println(str);
}
}
//save this database to the specified file
void save( File f ) throws IOException{
f = databaseFile;
}
//return the site at the specified index
int getPageCount() {
return pages.size();
}
//return the list of sites, wrapped to be unmodifiable
FavoritePage getPage(int index) {
return pages.get(index);
}
List getPages() {
return pages;
}
}
and we have two activities: site list and site detail like that:
site_list:
xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" xmlns:tools="http://schemas.android.com/tools" android:gravity="center" android:orientation="vertical"> <Button android:id="@+id/button1" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button1" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="16dp"/> <Button android:id="@+id/button2" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button2" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="58dp" /> <Button android:id="@+id/button3" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button3" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="100dp" /> <Button android:id="@+id/button4" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button4" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="142dp" /> <Button android:id="@+id/button5" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button5" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="184dp" /> <Button android:id="@+id/button6" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button6" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="226dp" /> <Button android:id="@+id/button7" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button7" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="268dp" /> <Button android:id="@+id/button8" android:layout_width="207dp" android:layout_height="42dp" android:text="@string/button8" tools:layout_editor_absoluteX="89dp" tools:layout_editor_absoluteY="310dp" /> LinearLayout>
site_detail:
xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingRight="16dp" android:paddingLeft="16dp" android:paddingBottom="16dp" android:paddingTop="16dp" android:orientation="vertical"> <TextView android:id="@+id/text_visit" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" android:text="@string/text_visit" /> <TextView android:id="@+id/text_url" android:layout_width="match_parent" android:layout_height="9dp" android:layout_weight="1" android:text="@string/text_url" /> <WebView android:id="@+id/web_view" android:layout_width="366dp" android:layout_height="413dp">WebView> LinearLayout>
How can do that in Android Studio:
Pressing a button on the site list should increment the visit count, save the updated database, and show the detail activity which should display the URL, visit count and a WebView showing site. // You can use fragments
Step by Step Solution
There are 3 Steps involved in it
Step: 1
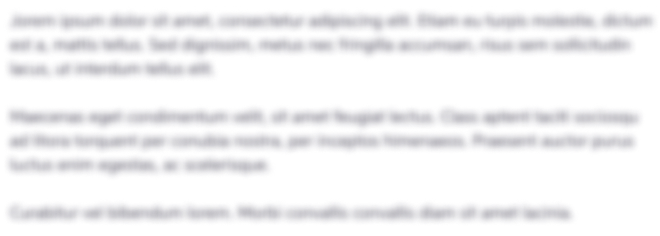
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started