Question
If you could please implement any of the methods w/o using any imports (ie. no LinkedList, or ArrayList etc..) Thank you VERY MUCH! ***********SOURCE CODE**********
If you could please implement any of the methods w/o using any imports (ie. no LinkedList, or ArrayList etc..) Thank you VERY MUCH!
***********SOURCE CODE********** public class Lab3CS3 { /** * A program to test the HashTable class */ public static void main(String[] args){ MyHashTable table = new MyHashTable(5); String key,value; while (true) { System.out.println(" Menu:"); System.out.println(" 0. Populate table"); System.out.println(" 1. Test put(key,value)"); System.out.println(" 2. Test get(key)"); System.out.println(" 3. Test containsKey(key)"); System.out.println(" 4. Test remove(key)"); System.out.println(" 5. Show contents of hash table."); System.out.println(" 6. EXIT"); System.out.print("Enter your command: "); java.util.Scanner sc=new java.util.Scanner(System.in); int item = sc.nextInt(); switch (item) { case 0: table.put("jan","1"); table.put("feb","2"); table.put("mar","3"); table.put("apr","4"); table.put("may","5"); table.put("jun","6"); table.put("jul","7"); table.put("aug","8"); table.put("sep","9"); table.put("oct","10"); table.put("nov","11"); table.put("dec","12"); break; case 1: sc=new java.util.Scanner(System.in); System.out.print("Enter Key : "); key = sc.next(); System.out.print("Enter Value: "); value = sc.next(); table.put(key,value); break; case 2: sc=new java.util.Scanner(System.in); System.out.print("Enter Key : "); key = sc.next(); System.out.print("Value for this key is "+table.get(key)); break; case 3: sc=new java.util.Scanner(System.in); System.out.print("Enter Key: "); key = sc.next(); System.out.println(" containsKey(" + key + ") is "+ table.containsKey(key)); break; case 4: System.out.print("Enter Key to remove: "); sc=new java.util.Scanner(System.in); key = sc.next(); table.remove(key); break; case 5: table.show(); break; case 6: return; default: System.out.println(" Illegal command."); break; } System.out.println(" Hash table size is " + table.size()); } } } /** Implementation from scratch of a HashTable with a fixed capacity array of linked list nodes The keys and values are both strings Given a key string, where does the (key, value) go? Use the function hash(key)= key.hashCode()%table-length */ class MyHashTable{ /** This is an "inner class" of Nodes that will be visible only to the outer class. */ private static class Node{ String key; String value; Node next; public Node(String key, String value){ this.key=key; this.value=value; next=null; } public String toString(){ return "("+key+","+value+")"; } } private Node[] nodeArray; //fixed size Array to hold the head of linked lists public int count=0; //total (key, value) pairs in the entire hash table public MyHashTable() { nodeArray = new Node[4]; //a very small table } /** * Generates the hash table with a specified initial size. * Precondition: initalSize > 0 */ public MyHashTable(int initialSize) { if (initialSize /** To put a new key/value pair in the hash table, first check if the key is already present in the table.. You could optionally also use this to change the value associated with an existing key. If the key is not present in the table, 1) new Node with the key and value as data. 2) find the hash function value of this node.key - it tells you which slot the node will go into. 3) Place the node at the end of the linked list whose head can be found at this slot */ public void put(String key,String value){ //Change this -Your Code here - return; } /** this is our hash function */ public int hash(String key){ return key.hashCode()%nodeArray.length; } /** return the value corresponding to this key, if the key exists */ public String get(String key){ //Change this - YOur Code return ""; } /** display the table on console */ public void show(){ //Change this - Your Code } /** Is the key anywhere in this hash table? */ public boolean containsKey(String key){ //Your Code - change this return false; } /** method to remove a node with supplied key. if key is not found, give a message and do nothing */ public void remove(String key){ //Change this - Your Code return; } public int size(){ return count; } }
Topic: Build your own hash table from scratch using chaining Policy: 1) Each lab is due at the end of each allocated Lab Section. The TA will be available at the allocated classroom to help you for the lab/homework. 2) Exception: if you plan to do the lab in advance, you must submit your lab answer before the start of your allocated lab section. Instruction: In this exercise, you are going to implement a hash table from scratch. You must not use any of the Java collection APIs. In this implementation of hash table, you will create a hash table of key-value pairs where both key and value are String objects. The hash table will be implemented using a fixed capacity array and chaining - if multiple key values have the same hash function value, the key-value pairs are put into a linked list. The basic unit of storage is a Node, consisting of a key, a value, and a reference to the next node, the same as the node used in a raw implementation of a linked list. Download the provided Java file. There is a menu-driven driver program class to test. Your job is to implement the missing methods to put (key, value) pairs into the table as nodes, and perform various other operations on them. Submission: Submit your code through Brightspace
Step by Step Solution
There are 3 Steps involved in it
Step: 1
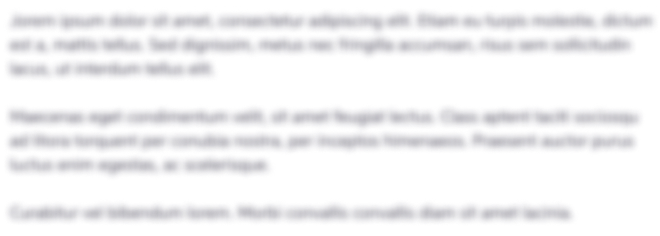
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started