Question
#ifndef _ANIMALS_H_ #define _ANIMALS_H_ #include using namespace std; enum Category { canidae = 0, felidae }; enum Subcategory { dog = 0, cat }; class
#ifndef _ANIMALS_H_ #define _ANIMALS_H_
#include
enum Category { canidae = 0, felidae }; enum Subcategory { dog = 0, cat };
class Animals { private: Category category; Subcategory subcategory; string name, breed; // private local variables int age;
public: Animals(Category animal_category, string animal_breed, Subcategory animal_subcategory, string animal_name, int animal_age); // constructor
// accessor methods Category getCategory(); string getBreed(); Subcategory getSubcategory(); string getName(); int getAge();
// Declare a friend function named changeAge which has 2 parameters and no return value. // The first parameter is a pointer to Animals, and the second is an int. // See the helper function in hw10.cpp for proper use of this function.
virtual void display(){ } };
#endif // _ANIMALS_H_
................................
#include "Canidae.h" #include "Animals.h"
#include
string Canidae::getCanidaeName() { string Name=getName(); return Name; }
int Canidae::getCanidaeAge() { int Age=getAge(); return Age; }
string Canidae::getCanidaeBreed(){ string breed=getBreed(); return breed; } void Canidae::display() { cout << "Category: Canidae" << endl << endl; cout << endl << "Breed: " << getBreed() << endl; }
// Q2a: Define Display for Dog class // Define the method display that you declared within the Dog class in the header file // Information should be printed in the following format: // Category: // Breed: // Subcategory: // Name: // Age: // (See the print_all function in hw10.cpp for proper use of this function. In this function you need to get the required values from Canidae class only.)
..............................................................
#include "Canidae.h" #include "Dog.h"
#include
#include "Felidae.h"
#include
string Felidae::getFelidaeName() { string felidae_name=getName(); return felidae_name; }
int Felidae::getFelidaeAge() { int felidae_age=getAge(); return felidae_age; }
string Felidae::getFelidaeBreed(){ string breed=getBreed(); return breed; } void Felidae::display() { cout << "Category: Felidae" << endl << endl; cout << endl << "Breed: " << getBreed() << endl; }
// Q2b: Define Display for Cat class // Define the method display that you declared within the Cat class in the header file // Information should be printed in the following format: // Category: // Breed: // Subcategory: // Name: // Age: // (See the print_all function in hw10.cpp for proper use of this function. In this function you need to get the required values from Felidae class only.) #include "Felidae.h" #include "Cat.h"
#include
................................
// READ BEFORE YOU START: // You are given a partially completed program that creates a list of animals. // Each animal has the corresponding information: Category, breed, subcategory, name and age. // In the Animals.h file, you will find the definition for this enum 'category'and 'subcategory'. // Animals on the list can be 2 different 'ctegories' : either a canidae or a felidae. // The classes Dog and Cat are subclasses of the Canidae and Felidae class respectively. // Both of these classes will have their own use of the virtual display method. // // To begin, you should trace through the given code and understand how it works. // Please read the instructions above each required function and follow the directions carefully. // If you modify any of the given code, the return types, or the parameters, you risk failing the automated test cases. // // You are to assume that all input is valid: // Valid name: String containing alphabetical letters beginning with a capital letter // Valid breed: String containing alphabetical letters beginning with a capital letter // All input will be a valid length and no more than the allowed amount of memory will be used
#include "Container.h" #include "Animals.h" #include "Canidae.h" #include "Felidae.h" #include "Dog.h" #include "Cat.h"
#include #include #include
using namespace std;
// forward declarations void flush(); void branching(char); void helper(char); void add_animal(Category, string, Subcategory, string, int); Animals* search_animal(Category, string, Subcategory, string, int); void remove_animal(Category, string, Subcategory, string, int); void remove_all(); void print_all();
void save(string); // 10 points void load(string); // 10 points
Container* list = NULL; // global list
int main() { _CrtSetDbgFlag(_CRTDBG_ALLOC_MEM_DF | _CRTDBG_LEAK_CHECK_DF); // Use to check for memory leaks in VS
load("Animals.txt");
char ch = 'i';
do { cout << "Please enter your selection" << endl; cout << "\ta: add a new animal to the list" << endl; cout << "\tc: change the age of an animal" << endl; cout << "\tr: remove an animal from the list" << endl; cout << "\tp: print all animals on the list" << endl; cout << "\tq: quit and save list of animals" << endl; cin >> ch; flush(); branching(ch); } while (ch != 'q');
save("Animals.txt"); remove_all(); list = NULL; return 0; }
void flush() { int c; do c = getchar(); while (c != ' ' && c != EOF); }
void branching(char c) { switch (c) { case 'a': case 'c': case 'r': case 'p': helper(c); break; case 'q': break; default: printf(" Invalid input! "); } }
// The helper function is used to determine how much data is needed and which function to send that data to. // It uses pointers and values that are returned from some functions to produce the correct ouput. // There is no implementation needed here, but you should study this function and know how it works. // It is always helpful to understand how the code works before implementing new features. // Do not change anything in this function or you risk failing the automated test cases. void helper(char c) { string breed, name; int age; Category category; Subcategory subcategory; int category_check = -1, subcategory_check=-1;
if (c == 'p') print_all(); else { cout << "Please enter the animal's breed: " << endl; cin >> breed;flush(); cout << endl << "Please enter the animal's name: " << endl; cin >> name;flush(); cout << endl << "Please enter the animal's age: " << endl; cin >> age;
while (!(category_check == 0 || category_check == 1)) { cout << endl << "Please select one of the following: " << endl; cout << "0. Candide " << endl; cout << "1. Felidae" << endl; cin >> category_check; }
category = (Category)category_check;
while (!(subcategory_check == 0 || subcategory_check == 1)) { cout << endl << "Please select one of the following: " << endl; cout << "0. Dog " << endl; cout << "1. Cat" << endl; cin >> subcategory_check; } subcategory=(Subcategory)subcategory_check; Animals* animal_result = search_animal(category, breed, subcategory, name, age);
if (c == 'a') // add animal { if (animal_result == NULL) { add_animal(category, breed, subcategory, name, age); cout << endl << "Animal added." << endl << endl; } else cout << endl << "Animal already on list." << endl << endl; } else if (c == 'c') // change animal age { if (animal_result == NULL) { cout << endl << "Animal not found." << endl << endl; return; }
cout << endl << "Please enter the new age for this animal: " << endl; cin >> age; flush();
changeAge(animal_result, age);
cout << endl << "Animal's age changed." << endl << endl; } else if (c == 'r') // remove animal { if (animal_result == NULL) { cout << endl << "Animal not found." << endl << endl; return; }
remove_animal(category, breed, subcategory, name, age); cout << endl << "Animal removed from the list." << endl << endl; } } }
// Q3b: Define Friend Function Change Age // Define the function changeAge that is declared within the Animals.h file. // This function sets the age value of the Animals pointer to the value of the int parameter.
// Q4: Add Animal // This function will be used to add a new animal to the tail of the global linked list. // You will need to use the enums category and 'subcategory' variable to determine which constructors to use. // Remember that search is called before this function, therefore, the new animal is not on the list.
// No implementation needed here, however it may be helpful to review this function Animals* search_animal(Category category, string breed, Subcategory subcategory, string name, int age) { Container* container_traverser = list;
while (container_traverser != NULL) { if (container_traverser->animals->getName() == name && container_traverser->animals->getBreed() == breed && container_traverser->animals->getCategory() == category && container_traverser->animals->getSubcategory() == subcategory) return container_traverser->animals;
container_traverser = container_traverser->next; }
return NULL; }
// No implementation needed here, however it may be helpful to review this function void remove_animal(Category category, string breed, Subcategory subcategory, string name, int age) { Container* to_be_removed;
if (list->animals->getName() == name && list->animals->getBreed() == breed && list->animals->getCategory() == category && list->animals->getSubcategory() == subcategory) { to_be_removed = list; list = list->next; delete to_be_removed->animals; delete to_be_removed; return; }
Container* container_traverser = list->next; Container* container_follower = list;
while (container_traverser != NULL) { if (container_traverser->animals->getName() == name && container_traverser->animals->getBreed() == breed && container_traverser->animals->getCategory() == category && container_traverser->animals->getSubcategory() == subcategory) { to_be_removed = container_traverser; container_traverser = container_traverser->next; container_follower->next = container_traverser; delete to_be_removed->animals; delete to_be_removed; return; } container_follower = container_traverser; container_traverser = container_traverser->next; } } // No implementation needed here, however it may be helpful to review this function void remove_all() { while (list != NULL) { Container* temp = list; list = list->next; delete temp->animals; delete temp; } }
// This function uses the virtual display() method of the Canidae, Felidae, Dog and Cat classes to print all Animals in an oragnized format. void print_all() { Container *container_traverser = list;
if (list == NULL) cout << endl << "List is empty!" << endl << endl;
while (container_traverser != NULL) { container_traverser->animals->display(); container_traverser = container_traverser->next; } }
// Q5a: Save (5 points) // Save the linked list of animals to a file using ofstream. // You will need to come up with a way to store the amount of Containers in linked list. // Hint: You may want to cast the enum 'type' to an int before writing it to the file.
// Q5b: Load (5 points) // Load the linked list of animals from a file using ifstream. // You will need to create the linked list in the same order that is was saved to a file. // You will need to create a new node for the linked list, then add it to the tail of the list. // Hint: If you casted the enum 'type' to an int, you will need to cast it back to a 'Type'. // You will use the 'type' variable read from the file to determine which constructor to use.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
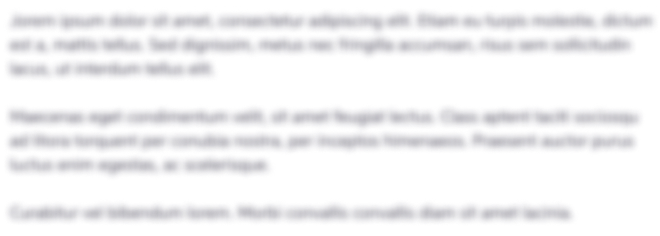
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started