Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#ifndef BST _ CHECKER _ H #define BST _ CHECKER _ H #include Node.h class BSTChecker { public: static Node * CheckBSTValidity ( Node
#ifndef BSTCHECKERH
#define BSTCHECKERH
#include "Node.h
class BSTChecker
public:
static Node CheckBSTValidityNode root
return CheckBSTValidityRecursiveroot nullptr, nullptr;
private:
static Node CheckBSTValidityRecursiveNode node, Node minNode, Node maxNode
if node nullptr
return nullptr;
Check if the node's key violates the BST property
if minNode nullptr && nodekey minNodekeymaxNode nullptr && nodekey maxNodekey
return node;
Check if the left child is linking to an ancestor
if nodeleft nullptr && nodeleft minNode nodeleft maxNode
return nodeleft;
Check if the right child is linking to an ancestor
if noderight nullptr && noderight minNode noderight maxNode
return noderight;
Recursively check the left subtree
Node leftViolation CheckBSTValidityRecursivenodeleft, minNode, node;
if leftViolation nullptr
return leftViolation;
Recursively check the right subtree
Node rightViolation CheckBSTValidityRecursivenoderight, node, maxNode;
if rightViolation nullptr
return rightViolation;
If no violations are found, return nullptr
return nullptr;
static bool IsDescendantNode ancestor, Node node
if ancestor node
return false;
if ancestor node
return true;
return IsDescendantancestorleft, node IsDescendantancestorright, node;
;
#endif
Cant seem to pass the last two tests being
:Unit test
Invalid tree with left child linking to parent
Test feedback
CheckBSTValidity returned a node that is not the BST ruleviolating node.
:Unit test
Invalid tree with left child linking to ancestor
Test feedback
CheckBSTValidity returned a node that is not the BST ruleviolating node.
Here are the read only main and node.h files
#include
#include
#include "Node.h
#include "BSTChecker.h
using namespace std;
int main
Node badNode;
Node userRoot;
string inputString;
inputString ;
userRoot Node::ParseinputString;
if userRoot
badNode BSTChecker::CheckBSTValidityuserRoot;
cout "Output should be: endl;
if badNode
cout tostringbadNodekey endl;
else
cout No violation" endl;
else
cout "Failed to parse input tree" endl;
Node::DeleteTreeuserRoot;
inputString ;
userRoot Node::ParseinputString;
if userRoot
badNode BSTChecker::CheckBSTValidityuserRoot;
cout "Output should be: No violation" endl;
if badNode
cout tostringbadNodekey endl;
else
cout No violation" endl;
else
cout "Failed to parse input tree" endl;
Node::DeleteTreeuserRoot;
inputString None, None None None;
userRoot Node::ParseinputString;
if userRoot
badNode BSTChecker::CheckBSTValidityuserRoot;
cout "Output should be: endl;
if badNode
cout tostringbadNodekey endl;
else
cout No violation" endl;
else
cout "Failed to parse input tree" endl;
Node::DeleteTreeuserRoot;
Node root new Node;
rootInsertAll;
Node userResult BSTChecker::CheckBSTValidityroot;
Check the user's result
if nullptr userResult
cout "BSTChecker::CheckBSTValidity correctly returned nullptr" endl;
else
cout "BSTChecker::CheckBSTValidity returned something other than nullptr ;
cout "for a valid BST Expected return value is nullptr." endl;
Node::DeleteTreeroot;
Node node new Node new Node new Node;
badNode new Node nullptr, new Node;
root new Node node badNode;
Call the user's CheckBSTValidity function
userResult BSTChecker::CheckBSTValidityroot;
Check the user's result
bool pass false;
if badNode userResult
cout "CheckBSTValidity correctly returned the ruleviolating node." endl;
pass true;
node.h file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
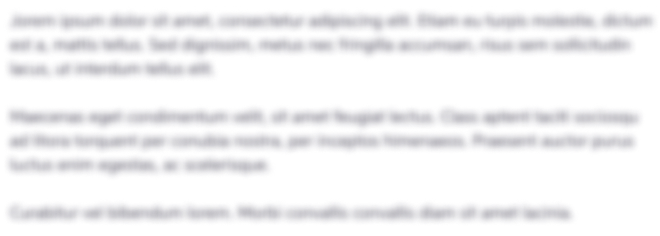
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started