Question
#ifndef HW3_BIGUINT_H #define HW3_BIGUINT_H #include #include using namespace std; class BigUInt { private: // Pointer to array. Each element of array contains a digit. //
#ifndef HW3_BIGUINT_H
#define HW3_BIGUINT_H
#include
#include
using namespace std;
class BigUInt {
private:
// Pointer to array. Each element of array contains a digit.
// Array length is equal to the number of digits of the BigUInt.
// data[0] contains the least significant digit.
// For example, if the BigUInt represents the number 385,
// then data[0] is 5, data[1] is 8, data[2] is 3, and length is 3.
unsigned char* data;
// The length of the array data.
int length;
public:
BigUInt();
BigUInt(unsigned int n);
~BigUInt();
void Print();
void TimesTenPow(unsigned int p);
BigUInt& operator+=(const BigUInt& rhs);
friend ostream& operator<<(ostream& os, const BigUInt& b);
// (a) (2 points) Write copy constructor such that this->data is
// pointing to different location as b.data.
BigUInt(const BigUInt& b);
// (b) (2 points) Write assignment operator such that this->data is
// pointing to different location as b.data.
BigUInt& operator=(const BigUInt& b);
// (c) (3 points) Write the += operator such that new value is equal
// to old value + i.
BigUInt& operator+=(unsigned int i);
// (d) (2 points) Implement SetDigit. This function sets the digit at
// position i. It is set to d. Check that the input i is valid.
// Check also that the input d is valid. If either input is invalid,
// this function returns without doing anything.
void SetDigit(int i, int d);
// (e) (3 points) Return a string representing this unsigned integer.
// For example, if the integer is 4892, then return a string "4892".
string ToString();
friend bool operator>(const BigUInt& lhs, const BigUInt& rhs);
};
ostream& operator<<(ostream& os, const BigUInt& b);
// (f) (4 points) Non-member friend function. Returns true of the value of
// lhs is greater than rhs, returns false otherwise.
bool operator>(const BigUInt& lhs, const BigUInt& rhs);
// (g) (4 points) Write a non-member function + that takes a BigUInt and an
// unsigned int, and returns that BigUInt that represents their sum.
BigUInt operator+(const BigUInt& b, unsigned int i);
#endif
//main
#include
#include "hw3.biguint.h"
using namespace std;
void func(BigUInt b) {
b.SetDigit(3, 5);
b.SetDigit(4, 7);
cout << b << endl;
}
int main() {
BigUInt b1(88408721);
func(b1);
cout << b1 << endl;
string s = b1.ToString();
cout << s << endl;
BigUInt b2(872);
b2 += 134908571;
cout << b2 << endl;
BigUInt b3(645050);
BigUInt b4(10050385);
b4 = b3;
b4.SetDigit(0, 9);
cout << b3 << " " << b4 << endl;
BigUInt b5(3459950);
BigUInt b6(8385);
if (b5 > b6) cout << "5 greater" << endl;
if (b6 > b5) cout << "6 greater" << endl;
BigUInt b7(3453450);
BigUInt b8(3458385);
if (b7 > b8) cout << "7 greater" << endl;
if (b8 > b7) cout << "8 greater" << endl;
BigUInt b10(3459950);
BigUInt b11(3459950);
if (b10 > b11) cout << "10 greater" << endl;
if (b11 > b10) cout << "11 greater" << endl;
BigUInt b12(99832395);
cout << b12 << endl;
b12.TimesTenPow(7);
cout << b12 << endl;
BigUInt b13(10);
b13 = b12 + 170814972;
cout << b13 << endl;
return 0
}
/*
Result:
88475721
88408721
88408721
134909443
645050 645059
5 greater
8 greater
99832395
998323950000000
998324120814972
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
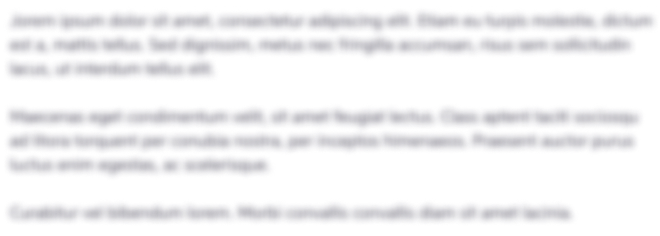
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started