Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#ifndef _ _ ICS 4 6 _ LL _ STACK _ HPP #define _ _ ICS 4 6 _ LL _ STACK _ HPP #include
#ifndef ICSLLSTACKHPP
#define ICSLLSTACKHPP
#include
#include
#include
namespace shindler::ics::project
class StackEmptyException : public std::runtimeerror
public:
explicit StackEmptyExceptionconst std::string& err
: std::runtimeerrorerr
;
struct Node
int data;
Node next;
;
This is the LinkedListStack class you will be implementing for this project.
Remember to see the writeup for more details!
template
class LinkedListStack
private:
fill in private member data here
Node head;
Node tail;
int linkedSize;
public:
constructor
LinkedListStack;
copy constructor remember deep copy!
LinkedListStackconst LinkedListStack& stackb;
assignment operator remember deep copy!
LinkedListStack& operatorconst LinkedListStack& stackb;
You do not need to implement these
LinkedListStackLinkedListStack&& delete;
LinkedListStack& operatorLinkedListStack&& delete;
destructor
~LinkedListStack;
nodiscard sizet size const noexcept;
nodiscard bool empty const noexcept;
We have two top functions. The first gets called if your
LinkedListStack is not a const, while the latter gets called on a const
LinkedListStack the latter might occur via const reference parameter,
for instance Be sure to test both! It is important when testing to
ensure that EVERY funtion gets called and tested!
nodiscard T& top;
nodiscard const T& top const;
void pushconst T& elem noexcept;
void pop;
;
template
LinkedListStack::LinkedListStack : headnullptr tailnullptr linkedSize
TODO: Fill in your constructor implementation here.
done using default member initialization
template
LinkedListStack::LinkedListStackconst LinkedListStack& stackb : headnullptr tailnullptr linkedSize
TODO: Fill in your copy constructor implementation here.
Node temp stackb.head;
if stackbhead nullptr
head nullptr;
else
whiletempnext nullptr
pushtempdata;
temp tempnext;
template
LinkedListStack& LinkedListStack::operator
const LinkedListStack& stackb
TODO: Fill in your assignment operator implementation here.
clear the existing content of the stack
ifthis &stackb
whileempty
pop;
copy content from stackb
Node temp stackb.head;
whiletempnext nullptr
pushtempdata;
temp tempnext;
return this; Stub so this function compiles without implementation.
template
LinkedListStack::~LinkedListStack
TODO: Fill in your destructor implementation here.
whileempty
pop;
template
sizet LinkedListStack::size const noexcept
TODO: Fill in your size implementation here.
return linkedSize;
template
bool LinkedListStack::empty const noexcept
TODO: Fill in your isEmpty implementation here.
bool check false;
ifhead nullptr
check true;
return check;
if linkedSize
check true;
return check;
return check;
template
T& LinkedListStack::top
TODO: Fill in your top implementation here.
The following is a stub just to get the template project to compile.
You should delete it for your implementation.
ifhead
return headdata;
template
const T& LinkedListStack::top const
TODO: Fill in your const top implementation here.
The following is a stub just to get the template project to compile.
You should delete it for your implementation.
if head
return headdata;
template
void LinkedListStack::pushconst T& elem noexcept
TODO: Fill in your push implementation here.
create a new list node
Node newNode new Node;
assign data with the new node
newNodenext nullptr;
newNodedata elem;
add the node to the top of the linked list
update head to new node
head newNode;
linkedSize;
template
void LinkedListStack::pop
TODO: Fill in your pop implementation here.
if head
assign local variable with head node data
Node temp head;
head headnext;
remove head node
delete temp;
decrement size variable
linkedSize;
namespace shindler::ics::proje
Step by Step Solution
There are 3 Steps involved in it
Step: 1
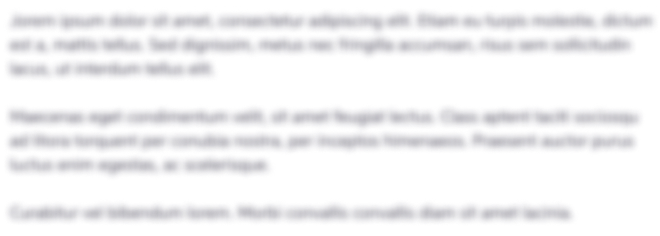
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started