Question
I'm a beginner in JAVA. Part A is done: public class GenericQueue { // A linked list (LL) node to store a queue entry private
I'm a beginner in JAVA.
Part A is done:
public class GenericQueue
// A linked list (LL) node to store a queue entry
private class QNode
{
T key;
QNode
// constructor to create a new linked list node
public QNode(T key) {
this.key = key;
this.next = null;
}
}
private QNode
private int N;
public GenericQueue() {
this.front = this.rear = null;
N = 0;
}
// Method to add an key to the queue.
public void addToQueue( T key)
{
// Create a new LL node
QNode
// If queue is empty, then new node is front and rear both
if (this.rear == null)
{
this.front = this.rear = temp;
return;
}
// Add the new node at the end of queue and change rear
this.rear.next = temp;
this.rear = temp;
N++;
}
// Method to remove an key from queue.
public T deleteFromQueue( )
{
// If queue is empty, return NULL.
if (this.front == null)
return null;
// Store previous front and move front one node ahead
QNode
this.front = this.front.next;
// If front becomes NULL, then change rear also as NULL
if (this.front == null)
this.rear = null;
N--;
return temp.key;
}
public boolean isEmpty() {
return N == 0;
}
public int size() {
return N;
}
public T lookUp() {
// If queue is empty, return NULL.
if (this.front == null)
return null;
return front.key;
}
public void clearQueue() {
this.front = this.rear = null;
N = 0;
}
public String toString() {
String res = "";
QNode
while(t != null) {
res = res + t.key+" ";
t = t.next;
}
return res;
}
}
Part B:
A Caesar cipher is a simple approach to encoding messages by shifting each letter in a message along the alphabet ( left or right ) by a constant amount k. For example, if k is 3 the letter A becomes D, b becomes e and so on. Similarly, if k is -2, E becomes C and f becomes d. In both cases you may need to wrap around to the beginning or end of the alphabet; for example, if k is -2 then A becomes Y.
An improvement to this encoding technique is to use a repeating key, i.e. each letter is shifted by a different amount using a list of key values. If the message has more letters than key values, you start over again at the beginning of the list. Here is an example:
encoding key list: -4 -1 -6 7 -4 5 -2 -3
Original message: | K | n | o | w | l | e | d | g | e |
| i | s |
| p | o | w | e | r |
Encoding key list: | -4 | -1 | -6 | 7 | -4 | 5 | -2 | -3 | -4 |
| -1 | -6 |
| 7 | -4 | 5 | -2 | -3 |
Encoded message: | G | m | i | d | h | j | b | d | a |
| h | m |
| w | k | b | c | o |
If you wanted to decode the message instead of encoding it, you would have to use the negative of each key value. For example:
Encoded message: | G | m | i | d | h | j | b | d | a |
| h | m |
| w | k | b | c | o |
-Encoding key list: | 4 | 1 | 6 | -7 | 4 | -5 | 2 | 3 | 4 |
| 1 | 6 |
| -7 | 4 | -5 | 2 | 3 |
Decoded message: | K | n | o | w | l | e | d | g | e |
| i | s |
| p | o | w | e | r |
Characters that are not letters remain as they are, for example blanks, commas, periods, apostrophes are left untouched.
Write the class Cipher. All data members and methods will be static. The static data members are the following:
a private static final String object (a constant) defining the encoding key, for example -4 -1 -6 7 -4 5 -2 -3
a static queue object of type Integer used to store the encoding key list. Each time a key value is used in the encoding or decoding process, it is deleted from the queue and then added right back in the queue (which will place it at the end of the queue).
The class will have the following two public static methods:
public static String encode(String): method that encodes the given message using the encoding key list. It returns the encoded message.
public static String decode(String): method that decodes the given message according to the encoding key (reversing the sign of each key value). It returns the decoded message.
IMPORTANT NOTES:
Define any number of private static methods that will allow you to perform the encoding and decoding efficiently.
Do not repeat whole sections of code for encoding and decoding (the only difference is the sign of the key value)
Uppercase letters are encoded and decoded to uppercase letters and lowercase letters are encoded and decoded to lowercase letters. Do not repeat whole sections of code to perform the encoding or decoding of uppercase letters and lowercase letters.
When you begin any encoding or decoding, the queue must contain the original encoding key.
Characters that are not letters remain as they are, for example blanks, commas, periods, apostrophes are left untouched.
Do not use ASCII values directly in your code; for example use int(a) instead of 97.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
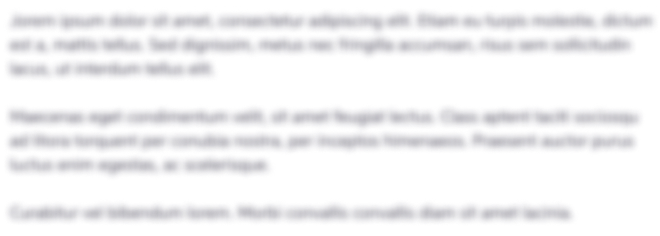
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started