Question
I'm building a Binary search sort with Ruby, but I can't get my code to work. class BinaryTree attr_accessor :payload, :left, :right def initialize(payload, left=nil,
I'm building a Binary search sort with Ruby, but I can't get my code to work.
class BinaryTree
attr_accessor :payload, :left, :right
def initialize(payload, left=nil, right=nil)
@payload = payload
@left = left
@right = right
end
end
def newNode(payload)
temp = BinaryTree.new(payload)
end
def storeSorted(tree, arr, i)
if tree.payload != nil
storeSorted(tree.left, arr, i)
arr << tree.payload
storeSorted(tree.right, arr, i)
end
end
def bstInsert(tree, payload)
return newNode(payload) unless tree.payload.nil?
if payload < tree.payload
tree.left = bstInsert(tree.left, payload)
elsif tree.payload > tree.payload
tree.right = bstInsert(tree.right, payload)
end
return tree
end
def sort(arr)
root = BinaryTree.new(nil, nil, nil)
root = bstInsert(root, arr[0])
arr.each {|i| bstInsert(root, arr[i])}
j = 0
storeSorted(root, arr, j)
end
arr = [7, 4, 9, 1, 6, 14, 10]
sort(arr)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
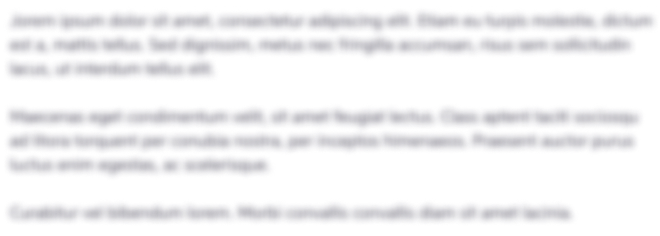
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started