Question
I'm currently working on the assignment below and I'm having issues with the print member function and the two operator functions. The print function I'm
I'm currently working on the assignment below and I'm having issues with the print member function and the two operator functions. The print function I'm having issues in the definition. Two required operator overloaded functions are << and + and Im having issues with the decleration and definition of them. Below are the instructions and below that is my current code in the form of header file, imp file, and driver.
//**********************************************************************
Part 1
Build a class listType based on statically allocated array. Its declaration is provided below (and in shell code provided with this HW):
const int MAX = 100; // max capacity for all listType objects class listType { public: listType(int max = 5); // constructor // Post: maxSize <-- max. if max is not specified or <=0, default value 5 will be used. if max > MAX, MAX will be used // size <-- 0. int getSize() const { return size; } // return # of elements actually stored int getMaxSize() const { return maxSize; } // return capacity bool isEmpty() const { return size == 0; } bool isFull() const { return size == maxSize; } int search(int element) const; // look for an item. return index of first occurrence int at(int index) const; // return element at a specific location void print() const; // print content of list on screen in format of [a, b, c] (like what ArrayList in Java does) bool insert(int element); // append/insert an element at the end bool insert(int index, int element); // insert an element into location index. // Shifts the element currently at that index (if any) and any subsequent elements to the right bool remove(int index); // remove element at the specified location private: int dataArr[MAX]; // static array storing data items int size; // actual # of elements stored. size <= maxSize int maxSize; // The capacity of this listType obj. 0 <= maxSize <= MAX. }; |
This class behaves like ArrayList in Java, except that each object has a capacity limit maxSize. The elements should always be stored at index [0] ~ [size-1] without any holes between.
Note that the definition of four of the member functions are already provided as inline functions.
search() will return the index of the first occurrence of element if found, otherwise return -1.
Use assert() (, chapter 4, p236) to handle invalid index parameter of at(): add this line as the first statement into at(): assert(index >= 0 && index < size);
The two insert()s and the remove() should return true when the operation is done successfully, and return false when the operation fails, such as attempt to insert into a full list, remove from an empty list, or if an invalid index is provided.
Provide appropriate driver code to test this class thoroughly (dont need to test the four given functions) before proceeding to Part 2.
Part 2
Modify your listType program from Part 1.
Overload output operator (<<) as a standalone function (non-member non-friend). It should work with cout and any ofstream object.
remove member function print()
Overload binary + operator as a member to add two listType objects: should return a listType object which contains all elements from the two operands (two listType objects). You dont need to handle duplicates or order the elements in any specific way.
Modify your driver accordingly to test those two operators. Here is the output of my partial driver:
Additional requirements for Part 1 and Part 2
Organize your program into header file (interface), implementation file, and driver file. Use #include guard with the header file.
Pre- and Post- condition comments for each function (except for the four given ones). Pre- condition may be skipped if its just xx is initialized. All parameters except for one should be IN type (either pass by value or const reference) therefore xx is initialized is implied.
Comment your program appropriately. Pay attention to the standard stuff like coding style, indention, heading, and curly braces
//***********************************************************
// listTypeProg.cpp (HW9 shell code)
// CS225
// HW9. a statically allocated array based list type class with overloaded operators
#pragma once
#include
const int MAX = 100; // max capacity for all listType objects
class listType
{
public:
listType(int max = 5); // constructor
// Post: maxSize <-- max. if max is not specified or <=0, default value 5 will be used. if max > MAX, MAX will be used
// size <-- 0.
int getSize() const { return size; } // return # of elements actually stored
int getMaxSize() const { return maxSize; } // return capacity
bool isEmpty() const { return size == 0; }
bool isFull() const { return size == maxSize; }
int search(int element) const; // look for an item. return index of first occurrence
int at(int index) const; // return element at a specific location
void print() const; // print content of list on screen in format of [a, b, c]
bool insert(int element); // append/insert an element at the end
bool insert(int index, int element);
// insert an element into location index
// Shifts the element currently at that index (if any) and any subsequent elements to the right
bool remove(int index); // remove element at the specified location
//listType operator+(const listType l) const; *****operator I'm having issues with in both decleration and definition.
//friend std::ostream& operator<<(std::ostream& output, const listType&); *****operator I'm having issues with in both decleration and definition.
private:
int dataArr[MAX]; // static array storing data items
int size; // actual # of elements stored. size <= maxSize
int maxSize; // The capacity of this listType obj. 0 <= maxSize <= MAX.
};
//*********************************************************************
#include
#include
#include "listType.h"
listType::listType(int max) //constructor
{
this->maxSize = max;
}
int listType::search(int element) const
{
for (int i = 0; i
{
if (dataArr[i] == element)
return i;
}
return -1;
}
int listType::at(int index) const
{
assert(index >= 0 && index < size);
{
return dataArr[index];
}
}
void listType::print() const //having issues with the definition of this member function
{
std::cout << "[ ";
for (int i = 0; i < size; i++)
{
std::cout << listType::at(i) << " ";
}
std::cout << "]";
}
bool listType::insert(int element)
{
if (size < maxSize)
{
dataArr[size++] = element;
return true;
}
else
{
return false;
}
}
bool listType::insert(int index, int element)
{
if (index >= 0 && index <= size)
{
if (size < maxSize)
{
int temp = dataArr[index];
dataArr[index] = element;
for (int i = index; i
{
int temp1 = dataArr[i + 1];
dataArr[i + 1] = temp;
temp = temp1;
}
dataArr[size++] = temp;
return true;
}
else
{
return false;
}
}
else
return false;
}
bool listType::remove(int index)
{
if (index >= 0 && index
{
for (int i = index; i
{
dataArr[i] = dataArr[i + 1];
}
size--;
return true;
}
else
return false;
}
//**************************************************************
#include
#include "listType.h"
int main()
{
listType *list1 = new listType(6);
listType *list2 = new listType(6);
list1->insert(3);
list1->insert(4);
list1->insert(5);
list1->insert(1);
list1->insert(8);
list1->insert(0, 5);
list1->insert(10);
list1->remove(4);
list1->insert(2, 10);
list2->insert(3);
list2->insert(4);
list2->insert(5);
list2->insert(1);
list2->insert(8);
list2->insert(0, 5);
list2->insert(10);
list2->remove(4);
list2->insert(2, 10);
list1->print;
//listType* l = list1 + list2;
//std::cout << l << std::endl;
return 0;
} //end main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
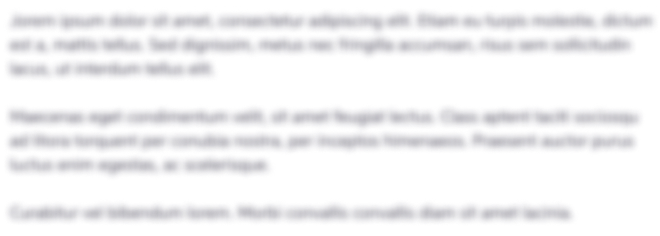
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started