Question
I'm facing an issue when I run my puzzle searchword program in GO the rows do not match test 4 go run ./cmd/wordsearch i puzzle2.txt
I'm facing an issue when I run my puzzle searchword program in GO the rows do not match test 4 “go run ./cmd/wordsearch i puzzle2.txt”
Here by my codes :
// Package main is a program to search for words in the puzzle
package main
import (
"bufio"
"errors"
"fmt"
"os"
)
func main() {
if len(os.Args) != 3 {
handleError(errors.New("Usage: wordsearch
return
}
word := os.Args[1]
puzzleFile := os.Args[2]
puzzle, err := readPuzzle(puzzleFile)
if err != nil {
handleError(err)
return
}
matches := findMatches(word, puzzle)
for _, match := range matches {
fmt.Println(match)
}
}
func handleError(err error) {
if err != nil {
if _, err := fmt.Fprintln(os.Stderr, err); err != nil {
// Handle any error that occurs while writing to os.Stderr
fmt.Printf("Error writing to os.Stderr: %v\n", err)
}
}
}
func readPuzzle(filePath string) ([][]rune, error) {
file, err := os.Open(filePath)
if err != nil {
return nil, err //nolint
}
defer file.Close()
var puzzle [][]rune
scanner := bufio.NewScanner(file)
for scanner.Scan() {
line := scanner.Text()
row := []rune(line)
puzzle = append(puzzle, row)
}
if scanner.Err() != nil {
return nil, scanner.Err()
}
return puzzle, nil
}
func findMatches(word string, puzzle [][]rune) []string {
var matches []string
directions := []struct {
dr, dc int
arrow rune
}{
{0, 1, '→'},
{0, -1, '←'},
{1, 0, '↓'},
{-1, 0, '↑'},
{1, 1, '↘'},
{-1, 1, '↗'},
{1, -1, '↙'},
{-1, -1, '↖'},
}
rows := len(puzzle)
cols := len(puzzle[0])
for r := 0; r < rows; r++ {
for c := 0; c < cols; c++ {
for _, dir := range directions {
if hasWord([]rune(word), puzzle, r, c, dir.dr, dir.dc) {
matches = append(matches, fmt.Sprintf("(%d, %d) %c", r+1, c+1, dir.arrow))
}
}
}
}
return matches
}
func hasWord(word []rune, puzzle [][]rune, r, c, dr, dc int) bool {
for i := 0; i < len(word); i++ {
// fmt.Println(r, len(puzzle), c, len(puzzle[0]), puzzle[r][c], rune
if r < 0 || r >= len(puzzle) || c < 0 || c >= len(puzzle[0]) || puzzle[r][c] != word[i] {
return false
}
r += dr
c += dc
}
return true
}
-----------------
And here he correct simple the program should generate :
$ cat puzzle1.txt
abcdefg
hiŮklmn
pqœstuv
$ cat puzzle2.txt
cxxxefg
bxfxhi0
cxxxc10
$ go run ./cmd/wordsearch de puzzle1.txt
(1, 4) →
$ go run ./cmd/wordsearch œke puzzle1.txt
(3, 3) ↗
$ go run ./cmd/wordsearch cx puzzle2.txt
(1, 1) →
(1, 1) ↘
(3, 1) →
(3, 1) ↗
(3, 5) ←
(3, 5) ↖
$ go run ./cmd/wordsearch i puzzle2.txt
(2, 6) ←
(2, 6) ↑
(2, 6) →
(2, 6) ↓
(2, 6) ↖
(2, 6) ↗
(2, 6) ↘
(2, 6) ↙
-------------------------
And here are the requirement structures for this program:
- Write a Go program and put your file(s) in
cmd/wordsearch/
.
2. The program's first argument is the word to search for in the puzzle. The second argument is either the file path to the puzzle or "-" which means the program will read the puzzle from standard input. The puzzle is a UTF-8 encoded text file with one line per row in the puzzle. The puzzle is rectangular so all rows must have the same number of columns. If they do not then write "inconsistent line length" to standard error and exit with exit code 3.
3. Output the matches one per line in the following format. (R, C) D
where R
is the row (1-based), C
is the column (1-based), and D
is the unicode direction arrow from the set →←↓↑↘↗↙↖
. The matches must be output in lexicographic order on the vector RCD
.
4. Be sure to close of file descriptor. You will loose a point for each file descriptor not closed.
5. Do not use any library other than the Go standard library.
6. The source code must compile with the most recent version of the Go compiler.
7. The program must not panic under any circumstances.
8. Make sure your code is "gofmt'd". See gofmt
or better use goimports
or better yet configure IDE to do this formatting on file save.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
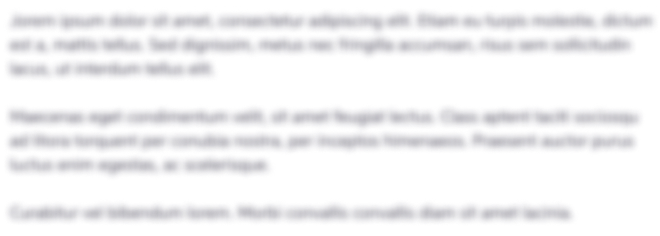
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started