Question
Im getting undefined reference errors for my main when running make. Help? main.o: In function `main': main.cpp:(.text+0x2a): undefined reference to `Stack ::Stack()' main.cpp:(.text+0x3e): undefined reference
Im getting undefined reference errors for my main when running make. Help?
main.o: In function `main':
main.cpp:(.text+0x2a): undefined reference to `Stack::Stack()'
main.cpp:(.text+0x3e): undefined reference to `Stack::push(int)'
main.cpp:(.text+0x52): undefined reference to `Stack::push(int)'
main.cpp:(.text+0x66): undefined reference to `Stack::push(int)'
main.cpp:(.text+0x7a): undefined reference to `Stack::push(int)'
main.cpp:(.text+0x8e): undefined reference to `Stack::push(int)'
main.cpp:(.text+0xb3): undefined reference to `Stack::top()'
main.cpp:(.text+0xfb): undefined reference to `std::ostream& operator<<(std::ostream&, Stack &)'
main.cpp:(.text+0x10a): undefined reference to `Stack::pop()'
main.cpp:(.text+0x133): undefined reference to `std::ostream& operator<<(std::ostream&, Stack &)'
main.cpp:(.text+0x142): undefined reference to `Stack::Stack()'
main.cpp:(.text+0x319): undefined reference to `Stack::push(Student)'
main.cpp:(.text+0x35e): undefined reference to `Stack::push(Student)'
main.cpp:(.text+0x3a3): undefined reference to `Stack::push(Student)'
main.cpp:(.text+0x3cb): undefined reference to `Stack::top()'
main.cpp:(.text+0x424): undefined reference to `Stack::pop()'
main.cpp:(.text+0x433): undefined reference to `Stack::isEmpty()'
main.cpp:(.text+0x453): undefined reference to `Stack::top()'
main.cpp:(.text+0x48a): undefined reference to `Stack::pop()'
main.cpp:(.text+0x4e1): undefined reference to `Stack::~Stack()'
main.cpp:(.text+0x4f0): undefined reference to `Stack::~Stack()'
main.cpp:(.text+0x66d): undefined reference to `Stack::~Stack()'
main.cpp:(.text+0x681): undefined reference to `Stack::~Stack()'
///////////Student.h file
#pragma once
#ifndef STUDENT_H
#define STUDENT_H
#include
#include
#include
using namespace std;
class Student {
private:
float gpa;
string ssn;
public:
Student();
void setGPA(float v);
void setSSN(string s);
void print();
class OutOfGPARange { }; // empty inner class definition under public
class InvalidSSN { }; // empty inner class definition under public
};
#endif
///////////////Student.cpp file
#include
#include
#include "Student.h"
Student::Student()
{
gpa = 0;
ssn = "";
}
void Student::setGPA(float g) {
//assert(g >= 0.0 && g <= 4.0);
//create an instance of the OutOfGPARange class, called exception
if (g < 0.0 || g > 4.0) throw OutOfGPARange();
gpa = g;
}
void Student::setSSN(string s) {
const int SSN_LENGTH = 9;
if (s.length() != SSN_LENGTH)
throw InvalidSSN();
//Checking each character is a digit should be here
//Otherwise, throw InvalidSSN();
ssn = s;
}
void Student::print() {
cout << "gpa: " << gpa << endl;
cout << "ssn: " << ssn << endl;
}
///////////////Stack.h file
#pragma once
#include
#include
#include
using namespace std;
template
class Stack
{
struct Node
{
T data;
Node *next;
};
Node *head;
public:
Stack();
~Stack();
void push(T data);
T top();
void pop();
template
friend ostream& operator<<(ostream &out, Stack
bool isEmpty();
};
///////////////Stack.cpp file
#include "Stack.h"
template
Stack
{
head = NULL;
}
template
Stack
{
Node *cur = head, *tmp;
while (cur != NULL)
{
tmp = cur->next;
delete cur;
cur = tmp;
}
}
template
void Stack
{
Node *cur = head, *newNode;
newNode = new Node;
newNode->data = data;
newNode->next = NULL;
if (head == NULL)
{
head = newNode;
}
else
{
newNode->next = head;
head = newNode;
}
}
template
T Stack
{
return head->data;
}
template
void Stack
{
Node *tmp = head;
head = head->next;
delete tmp;
}
template
ostream& operator<<(ostream &out, Stack
{
typename Stack
while (cur != NULL)
{
out << cur->data << " ";
cur = cur->next;
}
return out;
}
template
bool Stack
{
if (head == NULL)
return true;
return false;
}
////////////////Main.cpp file
#include
#include
#include"Stack.h"
#include"Student.h"
using namespace std;
int main() {
//declare stack of int type
Stack
intStack.push(5);
intStack.push(4);
intStack.push(3);
intStack.push(2);
intStack.push(1);
cout << "Top of intStack is : " << intStack.top() << endl;
cout << "intStack items are : ";
cout << intStack;
intStack.pop();
cout << " intStack items after one pop operation: ";
cout << intStack;
//now declare Stack of type Student
Stack
Student st[5];
st[0].setGPA(3.5);
st[0].setSSN("111111111");
st[1].setGPA(3.5);
st[1].setSSN("222222222");
st[2].setGPA(3.5);
st[2].setSSN("333333333");
//push some students onto stack
StudentStack.push(st[0]);
StudentStack.push(st[1]);
StudentStack.push(st[2]);
Student pt = StudentStack.top();
cout << "Top of the stack is : " << endl;
pt.print();
cout << "Print stack after one pop operation: ";
StudentStack.pop();
while (!StudentStack.isEmpty())
{
pt = StudentStack.top();
StudentStack.pop();
pt.print();
}
}
/////////////////Makefile
#
a.out: Student.o Stack.o main.o
g++ Student.o Stack.o main.o
Student.o: Student.h Student.cpp
g++ -c Student.cpp
Stack.o: Student.h Stack.h Stack.cpp
g++ -c Stack.cpp
main.o: Student.h Stack.h main.cpp
g++ -c main.cpp
clean:
rm a.out *.o
Step by Step Solution
There are 3 Steps involved in it
Step: 1
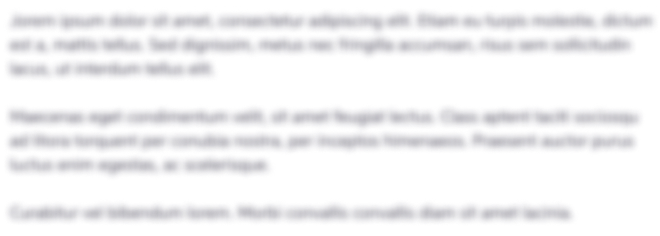
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started