Question
I'm having bugs on this code, I need help fixing it. This lab is about the shortest path based on flight times. The .dat file
I'm having bugs on this code, I need help fixing it. "This lab is about the shortest path based on flight times. The .dat file has city pairs which represent a potential flight option. The city pair is followed on the same line with an int that represents the flight time in minutes. So, an entry such as AUS HOU 60 would mean that there is a flight between Austin and Houston with a flight time of 60 minutes. Create a recursive graph-based solution in Java to find the lowest total time flight path from one city to another. Test it by finding the shortest flight time path from Austin to Seattle." Thank you.
.dat file:
LA SEATTLE 275 DEN SEATTLE 430 AUSTIN DAL 150 AUSTIN HOU 100 DAL KC 130 DAL DEN 300 KC LA 400 DEN LA 200
code:
import java.io.File; import java.io.FileNotFoundException; import java.util.Map; import java.util.HashMap; import java.util.Scanner; public class AirlineRoutes{ static int V = 7; static int INF = Integer.MAX_VALUE; public static int minimumCostSimplePath(int u, int destination,boolean visited[],int graph[][]){ if (u == destination){ return 0; } visited[u] = true; int ans = INF; for(int i = 0; i < V; i++){ if(graph[u][i] != INF && !visited[i]){ int curr = minimumCostSimplePath(i,destination, visited, graph); if(curr < INF){ ans = Math.min(ans, graph[u][i] + curr); } } } visited[u] = false; return ans; } public static void main(String[] args) throws FileNotFoundException { int graph[][] = new int[V][V]; for(int i = 0; i < V; i++){ for(int j = 0; j < V; j++){ graph[i][j] = INF; } } boolean visited[] = new boolean[V]; HashMap map=new HashMap(); HashMap cityNameMap=new HashMap(); HashMap distanceMap=new HashMap(); File file = new File("/Users/username/Downloads/flightTimes.dat"); Scanner read = new Scanner(file); read.useDelimiter(" "); int count = 0; while(read.hasNext()){ String[] data = read.next().split(" "); distanceMap.put(data[0]+"-"+data[1] , Integer.parseInt(data[2])); if(!cityNameMap.containsKey(data[0])){ cityNameMap.put(data[0] , count); count++; } if(!cityNameMap.containsKey(data[1])){ cityNameMap.put(data[1] , count); count++; } } for (Map.Entry entry : distanceMap.entrySet()) { String k = entry.getKey(); String source = k.split("-")[0]; String destination = k.split("-")[1]; Integer v = entry.getValue(); graph[cityNameMap.get(source)][cityNameMap.get(destination)] =v; } String s = "AUSTIN", t = "SEATTLE"; visited[cityNameMap.get(s)] = true; System.out.print("Shortest flight time between "+ s +" and "+ t +": "); System.out.println(minimumCostSimplePath(cityNameMap.get(s), cityNameMap.get(t), visited, graph)); } }
Step by Step Solution
3.52 Rating (145 Votes )
There are 3 Steps involved in it
Step: 1
To address the issues in your code Ill first address some critical points Mapping cities to integers Youre using integers to represent cities in the g...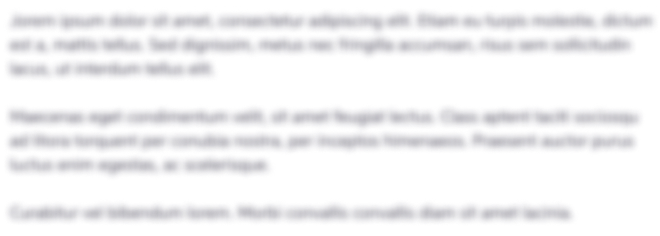
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started