Question
I'm not able to get out a tree output from this program. Please can someone help me to resolve the issue? Here is the code:
"I'm not able to get out a tree output from this program. Please can someone help me to resolve the issue?" Here is the code:
import javax.swing.*;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.io.IOException;
public class BinarySearchTreeMain {
private JRadioButton IsBalancedRadioButton;
ButtonGroup buttonGroup = new ButtonGroup();
private JTextField expressionEntryField, expressionResultField;
private JRadioButton InorderRadioButton;
private Component IsFullButton;
public static void main(String[] args) throws IOException {
BinarySearchTreeMain binarySearch = new BinarySearchTreeMain();
binarySearch.instantiateGui();
}
private void instantiateGui() {
JFrame window = new JFrame("Binary Tree Categorizer");
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = (JPanel) window.getContentPane();
window.setLayout(null);
JLabel promptLabel = new JLabel("Enter Tree");
promptLabel.setBounds(25, 15, 100, 20);
panel.add(promptLabel);
JLabel resultLabel = new JLabel("Output");
resultLabel.setBounds(25, 70, 100, 20);
panel.add(resultLabel);
expressionEntryField = new JTextField("", 30);
expressionEntryField.setBounds(125, 15, 350, 20);
panel.add(expressionEntryField);
expressionResultField = new JTextField("", 30);
expressionResultField.setBounds(125, 70, 350, 20);
panel.add(expressionResultField);
JButton MakeTreeButton = new JButton("MakeTree?");
MakeTreeButton.setBounds(175, 125, 125, 25);
MakeTreeButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, MakeTreeButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(MakeTreeButton);
JButton IsBalancedButton = new JButton("IsBalanced?");
IsBalancedButton.setBounds(175, 175, 125, 25);
IsBalancedButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, IsBalancedButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(IsBalancedButton);
JButton IsFullButton = new JButton("IsFull?");
IsFullButton.setBounds(175, 225, 125, 25);
IsFullButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, IsFullButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(IsFullButton);
JButton IsProperButton = new JButton("IsProper?");
IsProperButton.setBounds(175, 275, 125, 25);
IsProperButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, IsProperButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(IsProperButton);
JButton HeightButton = new JButton("Height?");
HeightButton.setBounds(175, 325, 125, 25);
HeightButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, HeightButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(HeightButton);
JButton NodesButton = new JButton("Nodes");
NodesButton.setBounds(175, 375, 125, 25);
NodesButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, NodesButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(NodesButton);
JButton InOrderButton = new JButton("InOrder?");
InOrderButton.setBounds(175, 425, 125, 25);
InOrderButton.addActionListener((ActionEvent e) -> {
try {
String entry = expressionEntryField.getText();
validateUserInput(entry);
validateUserSelections();
sortButtonAction(entry, InOrderButton);
} catch (Exception e1) {
System.out.println("Uncaught Exception: " + e1);
}
});
panel.add(InOrderButton);
window.getRootPane().setDefaultButton(MakeTreeButton);
window.setSize(500, 600);
window.setVisible(true);
}
private void validateUserSelections() {
}
private void sortButtonAction(String entry, AbstractButton MakeTreeRadioButton) throws Exception {
BinarySearchTree binarySearchTree;
String values[] = entry.split(" ");
// TODO: Optimize
if (MakeTreeRadioButton.isSelected()) {
binarySearchTree = new BinarySearchTree();
for (int i = 1; i < values.length; i++) {
if (values[i].split("/").length > 2) {
throw new Exception("Error. Malformed Fraction");
}
if (!values[i].contains("/")) {
Integer.parseInt(values[i]);
values[i] = values[i] + "/1";
}
binarySearchTree.bstInsert("int", (values[i]));
}
} else {
binarySearchTree = new BinarySearchTree(Integer.parseInt(values[0]));
for (int i = 1; i < values.length; i++) {
binarySearchTree.insert(Integer.parseInt(values[i]));
}
}
if (IsBalancedRadioButton.isSelected()) {
expressionResultField.setText(binarySearchTree.ascendingOrder(binarySearchTree.getRoot()));
} else {
expressionResultField.setText(binarySearchTree.ascendingOrder(binarySearchTree.getRoot()));
}
}
private void validateUserInput(String entry) throws Exception {
if (entry.equals("")) {
throw new Exception("Please Enter an Expression.");
}
for (int i = 0; i < entry.length(); i++) {
char c = entry.charAt(i);
if (!Character.isDigit(c) && c != '/' && !Character.isWhitespace(c)) {
throw new Exception("Error: Non numeric input detected.");
}
if (c == '/' && IsBalancedRadioButton.isSelected()) {
throw new Exception("Error: List of Integers contains a fraction.");
}
}
}
}
public class BinarySearchTree {
int key;
BinarySearchTree left, right;
public BinarySearchTree(int item) {
key = item;
left = right = null;
}
public BinarySearchTree() {
}
public void bstInsert(String string, String string2) {
}
public void insert(int parseInt) {
}
public Object getRoot() {
return null;
}
public String ascendingOrder(Object root) {
return null;
}
}
// Java program to introduce Binary Tree
class BinaryTree {
// Root of the Binary Tree
BinarySearchTree root;
// Constructors
BinaryTree(int key) {
root = new BinarySearchTree(key);
}
BinaryTree() {
root = null;
}
// tree.root = new Node(1);
// tree.root.left = new Node(2);
// tree.root.right = new Node(3);
// tree.root.left.left = new Node(4);
void printPostorder(BinarySearchTree node) {
if (node == null)
return;
// first recur on left subtree
printPostorder(node.left);
// then recur on right subtree
printPostorder(node.right);
// now deal with node
System.out.print(node.key + " ");
}
// Given a binary tree print this node in inorder
void printInorder(BinarySearchTree node) {
if (node == null)
return;
// first recur on left child
printInorder(node.left);
// the print the data of node
System.out.print(node.key + " ");
// now recur on right child
printInorder(node.right);
}
// Given a binary tree, print its nodes in preorder
void printPreorder(BinarySearchTree node) {
if (node == null)
return;
// first print data of node
System.out.print(node.key + " ");
// then recur on left subtree
printPreorder(node.left);
// now recur on right subtree
printPreorder(node.right);
}
public static void instantiateGui() {
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
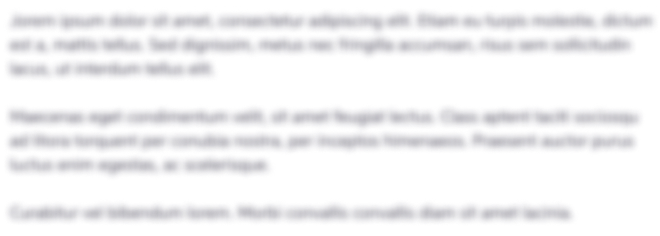
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started