Question
Im really struggling with my java class, i'm hoping someone can help do this assignment and add a lot of context so i can use
Im really struggling with my java class, i'm hoping someone can help do this assignment and add a lot of context so i can use this code to study, understand, and catch up with my class again, this includes explaining the UML diagram. Please be as descriptive as possible, and use intro level coding terminology.
This is the source code given:
DataLoader.java:
Employee.java:
HourlyEmployee.java:
PayrollReport.java:
SalaryEmployee.java:
Staff.java
Temporary.java:
Thats all the source code, once again please be as discriptive as possible, i am well versed in C so you dont have to explain everything, just most things. Thank you to who ever does this, and expect a thumbs up for all the hard work.
You will explore these concepts by completing a Java program that simulates a basic weekly payroll reporting system for the Cinco Corporation. Every employee has an employee ID, a name (first and last), and a title. Further, there are two types of employees: - Salaried employees (type: "Salary" ) - Salaried employees have a base annual salary, which is subject to a 20% income tax rate (state, federal and FICA combined). In addition, each salaried employee receives a $100 post-tax benefits allowance. - Hourly employees - Hourly employees have a per-hour pay rate along with a weekly total of the number of hours they worked. Hourly employees do not receive any benefit allowance. Further, there are two types of hourly employees. - Staff employees (type: "Staff") are directly employed by Cinco and are subject to a 15% income tax rate. - Temporary employees (type: "Temporary" ) are not directly employed by Cinco, but instead are contracted through a third-party temp agency who is responsible for collecting taxes (thus no taxes are taken from their gross pay). Employee data is stored in a flat data file and the basic parsing has been provided. However, you will need to design and implement Java classes to support and model this payroll system. By the end of this lab your class designs should resemble something like the following UML Diagram. A UML (Unified Modeling Language) Diagram is a graphical representation of classes and their relationships. Observe that: - Inheritance is indicated by the arrows - Abstract classes and methods have a small "A" in the icon - Public methods have a green icon and private member variables have red icons - Method parameters and return types are labeled You have been provided a partially completed program that parses a CSV data file (in DataLoader. java ) and creates Employee instances. However, the Employee class is empty. You will need to implement this class and any relevant subclass(es) to complete the program. - Identify the different dasses necessary to model each of the different types of employees in the problem statement. - Identify the relationship between these classes. - Identify the state (variables) that are common to these classes and the state that distinguishes them. Where do each of these pieces of data belong? - Identify the behavior (methods) that are common to each of these classes-what are methods that should be in the superclass? How should subclasses provide specialized behavior? - Some state/behavior may be common to several classes-is there an opportunity to define an intermediate class? - If you need more guidance, consult the UML diagram for one possible design. - To check your work, a text file containing the expected output has been provided in the project (see output/expectedoutput.txt ) Eclipse Tip: Many of the common programming tasks when dealing with objects can be automated by your IDE. For example: once you have designed the state (variables) of your class, you can automatically generate the boilerplate getters, setters, and constructors: when focused on your class, click "Source" then "Generate Getters and Setters" or "Generate Constructors using Fields." Java Note: In a subclass, you must invoke a constructor in the superclass and it must be done first. This rule is so that dasses conform to the is-a relationship. Invoking a super class's constructor is achieved by using the super keyword. If there are multiple constructors, you may invoke any one of them. Note that if a constructor does not explicitly invoke a superclass constructor, the Java compiler automatically inserts a call to the no-argument constructor of the superdass. If the superclass does not have a no-argument constructor, you will get a compile-time error. The Java object class has a no-arg constructor, so if your class does not explicitly extend a class, it implicitly 3.2 Abstractions Now that you have a well designed, functional implementation we will improve on the design by identifying potential abstractions that can be made. Start by making the Employee class abstract. If you had a good design, then nothing should break; the model did not have any generic employee. All employees were of a specific type. If something did break in your code, rethink your design and make the appropriate changes. Identify one or more methods in the Employee class that could be made abstract. Are there any methods in the superclass where it would not be appropriate to have a "default" definition? Make these methods abstract and again make any appropriate changes to your design as necessary. 3.3 Adding an Interface Cinco Corporation also has suppliers that they purchase supplies and parts from to build their products. Suppliers have a company name and an amount due, and so need to be paid, but they are not employees. However, the payroll department would like to have a common interface across all entities/objects in their system that are, in some way, payable. 1. Create an interface (in Eclipse: right click the package then "new" then "Interface") called Identify a single method in this interface that returns the (net) amount payable 2. Make the dass implement this new interface and make the appropriate changes if necessary 3. Create a new class, to represent suppliers and also make it 4. Testing, Submitting \& Grading - Test your programs using the provided JUnit test suite(s). Fix any errors and completely debug your programs. - Submit the following source files through webhandinStep by Step Solution
There are 3 Steps involved in it
Step: 1
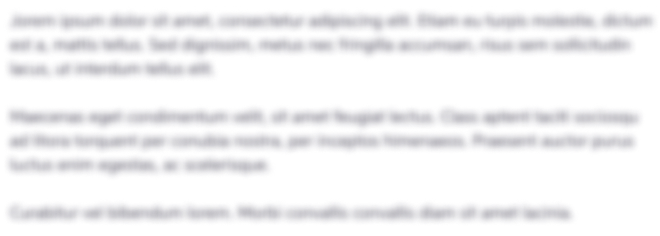
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started