Question
Im super stuck on a problem. I cant seem to figure it out. Please help me figure out the problem. I have a bug in
Im super stuck on a problem. I cant seem to figure it out. Please help me figure out the problem. I have a bug in it that I cant find. can you help me debug my problem, an how I can make my problem better
Problem
7.16 LAB: Leap year - functions (OPTIONAL - EXTRA CREDIT)
A year in the modern Gregorian Calendar consists of 365 days. In reality, the earth takes longer to rotate around the sun. To account for the difference in time, every 4 years, a leap year takes place. A leap year is when a year has 366 days: An extra day, February 29th. The requirements for a given year to be a leap year are:
1) The year must be divisible by 4
2) If the year is a century year (1700, 1800, etc.), the year must be evenly divisible by 400
Some example leap years are 1600, 1712, and 2016.
a program that takes in a year and determines whether that year is a leap year.
Ex: If the input is:
1712
the output is:
1712 is a leap year.
Ex: If the input is:
1913
the output is:
1913 is not a leap year.
Your program must define and call the following function. The function should return true if the input year is a leap year and false otherwise.
bool IsLeapYear(int userYear)
Note: This is a lab from a previous chapter that now requires the use of a function.
The code I have so far
#include
using namespace std;
bool isLeapYear(int userYear)
{
bool isLeapYear;
if (userYear % 400 == 0)
{
isLeapYear = true;
}
else if(userYear%100 != 0 && userYear%4 == 0)
{
isLeapYear = true;
}
else
{
isLeapYear = false;
}
return isLeapYear;
}
int main ()
{
int userYear;
cin >> userYear;
if (isLeapYear(userYear) == true)
{
cout << userYear << " is a leap year." << endl;
}
else
{
cout << userYear << " is not a leap year." << endl;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
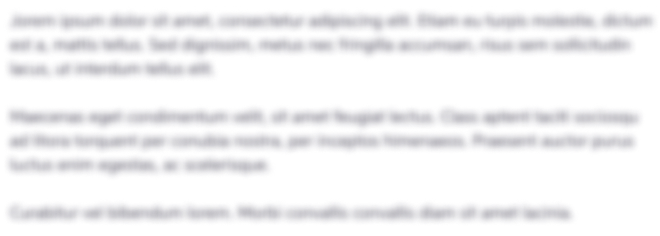
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started