I'm trying to finish up this one programming project I have to finish up before 12. The point of the project is that it's a
I'm trying to finish up this one programming project I have to finish up before 12. The point of the project is that it's a continuation of a previous one with some requirements added on. The point is that after the results of the masked table is created, you have to write a separate method to output the contents of the masked table to a file called masked.txt. The file will be opened as new clean output meaning it will not be opened as an appended file. The table should look like a table if the file is opened with a text editor with columns formatted and lined up properly. The file will then be close. Then, as a separate method in your program write code to open and input the table from the file masked.txt and output each line of input to the screen. Finally, all my file input and output have to have the necessary trap for exceptions (i.e file does not exist and so forth). What I'm having the most trouble with is the last three methods and figuring out how to call the into my main method without having them be connected to my other outputs.
Here is what I have so far:
import java.util.Scanner; import java.util.Random; /** This program reads a file with numbers, and writes the numbers to another * file, lined up in a column and followed by their total. */ import java.io.File; import java.io.FileNotFoundException; import java.io.FileWriter; import java.io.IOException; import java.io.PrintWriter; public class project2 { //Declaring a public string public static void main(String[]args) { //Declaring my scanner variable Scanner input = new Scanner(System.in); //Asking user to initialize my variables System.out.print("Please enter the number of columns you want the table to contain: "); int col = input.nextInt(); System.out.print("Now enter the number of rows you want the table to contain: "); int row = input.nextInt(); //Creating validation of user input System.out.println("You chose to have " + row + " rows & " + col + " columns. Here is what your table looks like."); int[][] original = ArrayInitializer(row, col); int[][] mask = MatrixConverter(original); //Creating space for the two tables System.out.println(); // Displaying matrixes System.out.println("Original matrix:"); displayOriginal(original); //Creating space for the two tables System.out.println(); // Displaying matrixes System.out.println("Binary matrix:"); displayMatrix(mask); } // Initializing my arrays & declaring the output in separate methods public static int[][] ArrayInitializer(int rowz, int colz) { int[][]original = new int[rowz][colz]; //Declaring my random variable Random random = new Random(); //Creating nested for loop for first # table for(int i = 0; i < rowz; i++) { for(int j = 0; j < colz; j++) { //Setting limit for randomly generated #s from 49 to -49 original[i][j] = random.nextInt(99)-49; } } return original; }
//Displaying the Original output in a separate method static void displayOriginal(int[][] originalarray) { int row = originalarray.length; int col = originalarray[0].length; for(int i = 0; i < row; i++) { for(int j = 0; j < col; j++) { System.out.print(originalarray[i][j] + " "); } //Display the first # table System.out.println(); } } // Creating method to convert Matrix output in a separate method public static int[][] MatrixConverter(int[][] original) { //Creating nested for loop for masked # table int row = original.length; int col = original[0].length; int[][] mask = new int[row][col]; for(int i = 0; i < row; i++) { for(int j = 0; j < col; j++) { //Converting positive and negative integers to 1s and 0s if(original[i][j] >= 0) { mask[i][j] = 1; } else { mask[i][j] = 0; } } } return mask; } //Displaying matrix output in a separate method static void displayMatrix(int[][] mask) { int row = mask.length; int col = mask[0].length; for(int i = 0; i < row; i++) { for(int j = 0; j < col; j++) { System.out.print(mask[i][j] + " "); } //Display the masked # table System.out.println(); } return; } //Declaring a new main method for the output of the displayMatrix in a text format public static void main1(String[] args) throws Exception { //Displaying the displayMatrix in a text file fileReader(); filewriter(); } //Declaring the fileReader in a new method public static void fileReader() throws FileNotFoundException { try { // Create a Scanner object for keyboard input. Scanner keyboard = new Scanner(System.in);
// Get the filename. System.out.print("Enter the filepath: "); String filename = keyboard.nextLine();
// Open the file. File file = new File(filename); Scanner inFile = new Scanner(file);
// Read lines from the file until no more are left. while (inFile.hasNext()) { // Read the next name. int Element = inFile.nextInt(); // Display the last name read. System.out.println(Element); }
// Close the file. inFile.close(); keyboard.close(); } catch (IOException e) { e.printStackTrace(); } } public static void filewriter() throws IOException { try { File dir = new File("Masked.txt"); dir.mkdirs(); File file = new File(dir, "filename.txt"); FileWriter archivo = new FileWriter(file); archivo.write(String.format("%20s %20s", "column 1", "column 2 ")); archivo.write(String.format("%20s %20s", "data 1", "data 2")); archivo.flush(); archivo.close(); } catch (IOException e) { e.printStackTrace(); } } } If anyone can help out within the next couple of hours that would be great.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
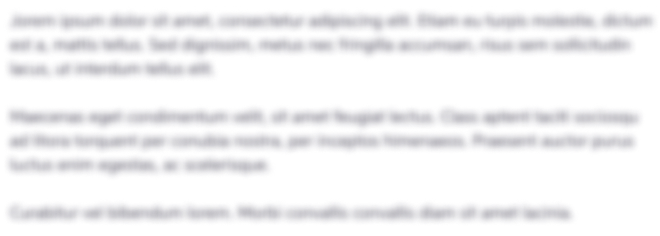
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started