Question
I'm trying to instead of inputting a numeric value, I instead input the letter grade with corresponding -/+ signs and output the numeric value. Example:
I'm trying to instead of inputting a numeric value, I instead input the letter grade with corresponding -/+ signs and output the numeric value.
Example: input: C-, output: 1.7
import java.util.Scanner;
public class Grade { public static void main(String[] args) { Scanner in = new Scanner(System.in); double mark = in.nextDouble(); in.close();
String grade = null;
if (mark < 0 || mark > 4) { System.out.println("Mark is outside the boundaries!"); return; } else if (mark >= 3.85) { grade = "A"; } else if (mark >= 3.7) { grade = "A-"; } else if (mark >= 3.0) { grade = "B+"; } else if (mark >= 2.85) { grade = "B"; } else if (mark >= 2.7) { grade = "B-"; } else if (mark >= 2.0) { grade = "C+"; } else if (mark >= 1.85) { grade = "C"; } else if (mark >= 1.7) { grade = "C-"; } else if (mark >= 1.0) { grade = "D+"; } else if (mark > 0.85) { grade = "D"; } else if (mark > 0.7) { grade = "D-"; } else { grade = "F"; }
System.out.printf("Grade of student is %s", grade);
in.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
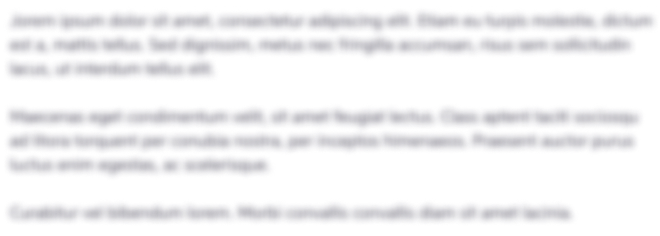
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started