Question
Implement a BigNumber class that stores nonnegative integers with up to 1000 digits. The digits will be stored as array elements in reverse order, e.g.
Implement a BigNumber class that stores nonnegative integers with up to 1000 digits. The digits will be stored as array elements in reverse order, e.g. 234 is stored with 4 stored in location zero, 3 stored in location 1 and 2 stored in location 2 . This approach is required, not optional.
You will implement the BigNumber.cpp file to go with the BigNumber.h below. You'll also want to implement a driver.cpp to test all member functions. For example ...
BigNumber n(234);
cout << n << endl; (etc.)
// insert tests for all the operations
___________________________________________________
Your implementation for multiplication should be efficient enough to compute factorials using a function similar to the one below. You should be able to compute factorial(100) without much time delay.
BigNumber factorial(const BigNumber & num) {
BigNumber temp(1), num2(num);
while (num2 > 1) {
temp *= num2--;
}
return temp;
}
HERE IS MY CODE;
BigNumber.h
#ifndef BIGNUMBER_H
#define BIGNUMBER_H
#include
#include
using namespace std;
class BigNumber
{
friend ostream &operator<<(ostream &, const BigNumber &); // stream insertion operator
friend istream &operator >> (istream &, BigNumber &); // stream extraction operator
private:
int digits[1000]; // Note: digits[i] represents the 10^i's place digit.
int numDigits; // number of digits starting from leading nonzero digit.
// (This means zero has numDigits equal to 0.)
public:
BigNumber(int value = 0); // default constructor and int constructor.
BigNumber(string value); // string constructor.
int getNumDigits() const; // return numDigits
bool isZero() const; // return true iff *this is equal to ZERO
bool operator< (const BigNumber & c) const; // less than
bool operator> (const BigNumber & c) const; // greater than
bool operator>= (const BigNumber & c) const; // greater than or equal
bool operator<= (const BigNumber & c) const; // less than or equal
bool operator== (const BigNumber & c) const; // equal
bool operator!= (const BigNumber & c) const; // not equal
BigNumber & operator++ (); // preincrement
BigNumber operator++ (int dummy); // postincrement
BigNumber operator+ (const BigNumber & c) const; // plus
BigNumber& operator+= (const BigNumber & c); // plus equals
BigNumber operator* (const BigNumber & c) const; // times
BigNumber& operator*= (const BigNumber & c); // times equals
};
#endif
BigNumber.cpp
#include "BigNumber.h"
#include
#include
using namespace std;
ostream &operator<<(ostream & out, const BigNumber & num) // stream insertion operator
{
for (int i = num.numDigits - 1; i >= 0; i--)
out << num.digits[i];
return out;
}
istream &operator >> (istream &, BigNumber &) // stream extraction operator
{
}
BigNumber::BigNumber(int value = 0) // default constructor and int constructor.
{
}
BigNumber::BigNumber(string value) // string constructor.
{
for (int i = 0; i < 1000; i++)
digits[i] = 0;
int stringLength = value.length();
numDigits = 0;
for (int i = stringLength - 1; i >= 0; i--)
{
digits[numDigits++] = value[i] - '0'; // minus zero to get real value and not ascii value.
}
}
int BigNumber::getNumDigits() const // return numDigits
{
return numDigits;
}
bool BigNumber::isZero() const // return true iff *this is equal to ZERO
{
return numDigits == 0;
}
bool BigNumber::operator< (const BigNumber & c) const // less than
{
if (this->numDigits > c.numDigits)
{
return false;
}
if (this->numDigits < c.numDigits)
{
return true;
}
for (int i = numDigits - 1; i >= 0; i--)
{
if (this->digits[i] != c.digits[i])
return c.digits[i] > this->digits[i];
}
return false;
}
bool BigNumber::operator> (const BigNumber & c) const // greater than
{
}
bool BigNumber::operator>= (const BigNumber & c) const // greater than or equal
{
}
bool BigNumber::operator<= (const BigNumber & c) const // less than or equal
{
}
bool BigNumber::operator== (const BigNumber & c) const // equal
{
}
bool BigNumber::operator!= (const BigNumber & c) const // not equal
{
}
BigNumber & BigNumber::operator++ () //preincrement
{
// missing code
return *this;
}
BigNumber BigNumber::operator++ (int dummy) // postincrement
{
BigNumber temp = *this;
// ++*this; // or // these 3 are
operator++(); // or // the same
// this->operator++(); // thing.
return temp;
}
BigNumber BigNumber::operator+(const BigNumber & c) const // plus
{
BigNumber temp = *this; // or BigNumber temp(*this);
temp += c;
return temp;
}
BigNumber & BigNumber::operator+= (const BigNumber & other) // plus equals
{
int loopBound = numDigits;
if (other.numDigits > numDigits)
loopBound = other.getNumDigits;
int carry = 0;
// use a for loop to access each digit of other and
// call addDigit to add it to the corresponding
// digit of the current object.
// Then check whether a last carry needs to be added
// and whether numDigits needs to be updated.
return *this;
}
BigNumber BigNumber::operator* (const BigNumber & c) const // times
{
}
BigNumber & BigNumber::operator*= (const BigNumber & other) // times equal
{
}
Source.cpp
#include "BigNumber.h"
#include
#include
using namespace std;
int main()
{
BigNumber myNumber("499"), yourNumber("598"); //ourNumber("575");
// cout << ">" << myNumber << "<" << endl;
// cout << "Number of digits is " << myNumber.getNumDigits() << endl;
cout << (myNumber < yourNumber) << endl;
cout << (yourNumber < myNumber) << endl;
cout << (yourNumber < yourNumber) << endl;
system("pause");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
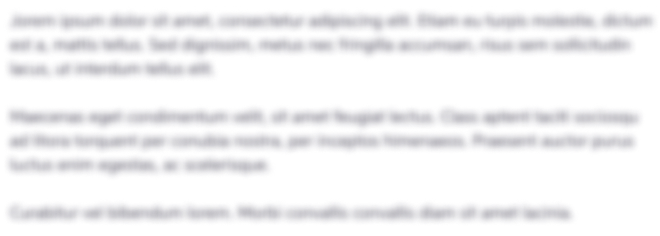
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started