Question
Implement a class named LongInteger. The class contains: A private data field of type long A constructor that takes parameter of type long and creates
Implement a class named LongInteger. The class contains:
- A private data field of type long
- A constructor that takes parameter of type long and creates LongInteger object for the specified long value.
- toLong() method that returns the value of the field.
- The instance methods isEven(), isOdd(), and isPrime() that return true if the value in the object is even, odd, or prime respectively.
- The static methods isEven(long), isOdd(long), and isPrime(long) that return true if the specified value is even, odd, or prime respectively.
- The instance method equals(long) and instance method equals(LongInteger) that return true if the value in this object is equal to the specified value.
- A static method parseLong(String) that converts a string into an long value, positive or negative. Method throws IllegalArgumentException when
- A string contains non-digit characters (other than '-' as the very first character of the sting).
- A string has only '-' and no digits.
- A string represents a number that is too large to be stored as long and produces overflow
- A string represents a number that is too small to be stored as long and produces underflow
Requirements:
- Create string parsing method parseLong(String). Do not use any existing methods that convert string into a long or a floating point number of any sort.
- Make sure to comment explaining your way of method implementation
CODE
public class LongInteger {
public static void main(String[] args) {
// ... other code ...
String longString = "1234567890123456789";
long longValue = LongInteger.parseLong(longString);
System.out.println("Parsed long value: " + longValue);
// ... other code ...
}
private long value;
// Constructor
public LongInteger(long value) {
this.value = value;
}
// Get the value of the LongInteger object
public long toLong() {
return this.value;
}
// Check if the LongInteger object is even
public boolean isEven() {
return this.value % 2 == 0;
}
// Check if the LongInteger object is odd
public boolean isOdd() {
return this.value % 2 != 0;
}
// Check if the LongInteger object is prime
public boolean isPrime() {
if (this.value <= 1) {
return false;
}
for (int i = 2; i <= Math.sqrt(this.value); i++) {
if (this.value % i == 0) {
return false;
}
}
return true;
}
// Check if a long value is even
public static boolean isEven(long value) {
return value % 2 == 0;
}
// Check if a long value is odd
public static boolean isOdd(long value) {
return value % 2 != 0;
}
// Check if a long value is prime
public static boolean isPrime(long value) {
if (value <= 1) {
return false;
}
for (int i = 2; i <= Math.sqrt(value); i++) {
if (value % i == 0) {
return false;
}
}
return true;
}
// Check if the LongInteger object is equal to a long value
public boolean equals(long value) {
return this.value == value;
}
// Check if the LongInteger object is equal to another LongInteger object
public boolean equals(LongInteger other) {
return this.value == other.toLong();
}
// Parse a string into a long value
public static long parseLong(String s) {
// check for empty string
if (s.length() == 0) {
throw new IllegalArgumentException("Empty string");
}
// check for negative sign
boolean negative = false;
int startIndex = 0;
if (s.charAt(0) == '-') {
negative = true;
startIndex = 1;
}
// check for non-digit characters
for (int i = startIndex; i < s.length(); i++) {
char c = s.charAt(i);
if (c < '0' || c > '9') {
throw new IllegalArgumentException("Non-digit character: " + c);
}
}
// parse digits
long result = 0;
for (int i = startIndex; i < s.length(); i++) {
char c = s.charAt(i);
int digit = c - '0';
result = result * 10 + digit;
// check for overflow/underflow
if ((!negative && result < 0) || (negative && result > 0)) {
throw new IllegalArgumentException("Number out of range: " + s);
}
}
// return result with sign
return negative ? -result : result;
}
TEST CODE
import java.io.PrintWriter;
public class TestLongInteger {
public static boolean tests(PrintWriter outputStream)
{
outputStream.println("\r\n----LongInteger Class TEST SETS -------------------------------------------------------\r\n");
boolean t1 = test01LongIntegerClass(outputStream);
boolean t2 = test02LongIntegerClass(outputStream);
boolean t3 = test03LongIntegerClass(outputStream);
return t1&&t2&&t3;
}
public static boolean test01LongIntegerClass(PrintWriter outputStream)
{
int count = 0;
int expectedCount = 3;
int a = 55;
int b = 102;
int prime = 97;
//Test #1
LongInteger num1 = new LongInteger(a);
if(num1.toLong()==a)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: constructor", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: constructor", "FAILED");
//Test #2
LongInteger num2 = new LongInteger(b);
if(!num1.isEven()&& num1.isOdd()&& num2.isEven() && !num2.isOdd())
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: isEven() and isOdd()", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: isEven() and isOdd()", "FAILED");
//Test #3
LongInteger num3 = new LongInteger(prime);
if(num3.isPrime() && !num2.isPrime() && !num1.isPrime())
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: isPrime()", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 01: isPrime()", "FAILED");
if (count==expectedCount) return true;
else return false;
}
public static boolean test02LongIntegerClass(PrintWriter outputStream)
{
int count = 0;
int expectedCount = 3;
int a = 103;
int b = 500;
int prime = 919;
//Test #1
if(!LongInteger.isEven(a)&& LongInteger.isOdd(a)&& LongInteger.isEven(b) && !LongInteger.isOdd(b))
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: static isEven() and static isOdd()", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: static isEven() and static isOdd()", "FAILED");
//Test #2
if(LongInteger.isPrime(prime) && LongInteger.isPrime(a) && !LongInteger.isPrime(b))
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: static isPrime()", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: static isPrime()", "FAILED");
//Test #3
LongInteger num1 = new LongInteger(a);
LongInteger num2 = new LongInteger(a);
LongInteger num3 = new LongInteger(b);
if(num1.equals(a) && !num1.equals(b) && num1.equals(num2) && !num3.equals(num1))
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: both equals methods", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 02: both equals methods", "FAILED");
if (count==expectedCount) return true;
else return false;
}
public static boolean test03LongIntegerClass(PrintWriter outputStream)
{
int count = 0;
int expectedCount = 4;
//Test #1
if(LongInteger.parseLong("9223372036854775807") == 9223372036854775807L && LongInteger.parseLong("12") == 12 && LongInteger.parseLong("0") == 0)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() with valid positive values", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() with valid positive values", "FAILED");
//Test #2
if(LongInteger.parseLong("-54321") == -54321 && LongInteger.parseLong("-9223372036854775808") == -9223372036854775808L && LongInteger.parseLong("-3") == -3)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() with valid negative values", "PASSED");
count++;
}
else outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() with valid negative values", "FAILED");
//Test #3
try // overflow detection test
{
long k = LongInteger.parseLong("9223372036854775808");
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() overflow", "FAILED");
}
catch(IllegalArgumentException e)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() overflow", "PASSED");
count++;
}
//Test #4
try // underflow detection test
{
long k = LongInteger.parseLong("-9223372036854775809");
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() underflow", "FAILED");
}
catch(IllegalArgumentException e)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() underflow", "PASSED");
count++;
}
//Test #5
try // non-digit characters detection test
{
long k = LongInteger.parseLong("456a7");
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() invalid digits", "FAILED");
}
catch(IllegalArgumentException e)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() invalid digits", "PASSED");
count++;
}
//Test #6
try // non-digit characters detection test (special case)
{
long k = LongInteger.parseLong("-");
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() invalid digits (just '-')", "FAILED");
}
catch(IllegalArgumentException e)
{
outputStream.printf("%-80s%-10s\n", "LongInteger TEST SET 03: parseLong() invalid digits (just '-')", "PASSED");
count++;
}
outputStream.println("\nREMINDER! You are not allowed to use any standard parsing method like Long.parseLong() when coding up LongInteger.parseLong(). ");
if (count==expectedCount) return true;
else return false;
}
}
TEST ALL PORTION
import java.io.*;
public class TestAll {
public static void main(String[] args)
{
int count = 0;
int expectedCount = 3;
try
{
System.out.println("Starting...");
File file = new File("_gradingLog.txt");
if(file.exists())
{
file.delete();
}
FileWriter fw = new FileWriter(file,true);
PrintWriter out = new PrintWriter(fw,true);
if(TestPoint.tests(out)) count++;
if(TestRightTriangle.tests(out)) count++;
if(TestLongInteger.tests(out)) count++;
out.close();
out = new PrintWriter("tmp.txt");
if (count==expectedCount) out.print("PASSED");
else {
out.print("FAILED!!!");
}
out.close();
System.out.println("Testing complete. See results in file \"_gradingLog.txt\"");
}
catch (IOException e)
{
System.out.println("File IO troubles"+ e.getMessage());
}
}
}
ERRORS IM GETTING
Starting...
Exception in thread "main" java.lang.IllegalArgumentException: Number out of range: -54321
at LongInteger.parseLong(LongInteger.java:115)
at TestLongInteger.test03LongIntegerClass(TestLongInteger.java:111)
at TestLongInteger.tests(TestLongInteger.java:9)
at TestAll.main(TestAll.java:22)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
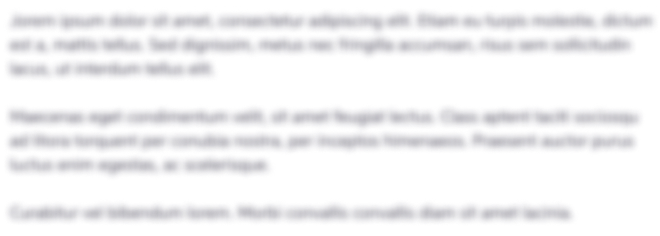
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started