Question
Implement a class Student. For the purpose of this exercise, a student has a name and a total quiz score. Supply an appropriate constructor and
Implement a class Student. For the purpose of this exercise, a student has a name and a total quiz score. Supply an appropriate constructor and functions get_name(), add_quiz(int score), get_total_score(), and get_average_score(). To compute the latter, you also need to store the number of quizzes that the student took. Then, modify the Student class to compute grade point averages. Member functions are needed to add a grade, and get the current GPA. Specify grades as elements of a class Grade. Supply a constructor that constructs a grade from a string, such as "B+". You will also need a function that translates grades into their numeric values (for example, "B+" becomes 3.3).
Write a C++ Program using the skeleton code below:
#include
class Student { public: Student(); void add_grade(const Grade &g); private: double m_quiz_total; int m_quiz_count; };
class Grade { public: Grade(const std::string &grade_string); private: std::string m_grade_string; double grade_value; };
Student::Student() { } void Student::add_grade(const Grade &g) { }
Grade::Grade(const std::string &grade_s) { }
int main() { bool done = false; Student student; // get the student data before processing grades while(! done) { std::string grade_string; // = prompt_grade_string(); student.add_grade(Grade(grade_string)); // prompt user for more grades to add yes or no // if user answers no then set done to true } //student.print_average(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
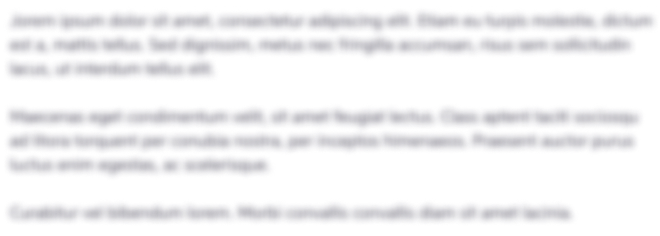
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started