Question
Implement a HashTable for integers using open hashing to represent sets of integers. You must implement the hash table yourself and are not allowed to
Implement a HashTable for integers using open hashing to represent sets of integers.
You must implement the hash table yourself and are not allowed to use the C++ standard
template library, i.e., you will need to implement a class for the set. Your set must provide
the basic set operations as discussed in class (add, delete, search, show, quit). Each
element of the set will be stored in a node object, i.e. you will need a separate class that
handles the nodes.
Upon starting your program, it will display the following prompt: set> (followed by a
space):
set>
The user can then add, delete, search, or show the set. If the element is already in the
set and you attempt to add that element to the set, then you need to display an
informational warning message. Similarly, if the element is not already in the set and you
attempt to delete that element from the set, then you need to display an informational
warning message.
Your hash table will have B=7 buckets and your hash function is h(x) = x^2 mod B.
FUNCTION DETAILS:
add will insert a number into the set and is followed by the prompt. If the element is
already in the set, provide an informational message WARNING: duplicate input: #
set> add 7
set> add 7
WARNING: duplicate input: 7
set> add 3
set>
delete will delete an element, if it is in the set. If the element does not exist, provide an
informational message WARNING: target value not found: #
set> delete 3
set> delete 8
WARNING: target value not found: 8
set>
search will return true or false to indicate if the element has been found
set> search 7
true
set> search 4
false
set>
show will list all the elements of the hash table in the following form:
set> show
()-()-()-()-()-()-()
set>
The parenthesis will contain the elements of the individual buckets, separated by
commas. The above example is the empty set
Assume the hash table contains the following elements (order of insertion matters, since
elements are appended at the end of a list for each bucket)
set> add 1
set> add 2
set> add 3
set> add 4
set> add 5
set> add 6
set> add 7
set> show
(7)-(1,6)-(3,4)-()-(2,5)-()-()
set>
quit will exit the program
set> quit


Step by Step Solution
There are 3 Steps involved in it
Step: 1
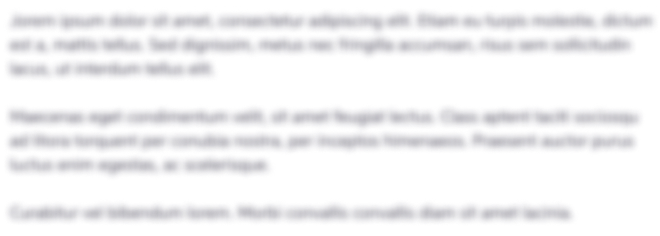
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started