Question
Implement a merge sort algorithm Implement a recursive algorithm You are not allowed to use any of the standard Java collection types for this assignment.
Implement a merge sort algorithm
Implement a recursive algorithm
You are not allowed to use any of the standard Java collection types for this assignment. Do not import any Java standard library features from java.util.
Problem Description and Given Info
For this assignment you are given the following Java source code files:
IList.java (This file is complete make no changes to this file)
MyArrayList.java (This file is complete make no changes to this file)
MySorts.java (You must complete this file)
Main.java (You may use this file to write code to test your quicksort code)
You must complete the public class named MySorts.java with methods as defined below.
Note that your *merge sort algorithm must be implemented to operate on IList objects, as defined in the given files iList.java and MyArrayList.java.
Structure of the MySorts Fields
Your merge sort algorithm should require no static (or instance) fields in your MySorts class.
Structure of the MySorts merge sort Methods
Your MySorts class must implement the following methods:
a public static method named mergesort that takes an object of type IList
a private static method named mergesortHelper that takes an object of type IList
a private static method named getLeftHalf that takes an object of type IList
a private static method named getRightHalf that takes an object of type IList
a private static method named merge that takes two IList
You may implement any additional methods that you may need.
Additional Information
MySorts merge sort algorithm
the mergesort method will take a reference to an IList
the mergesortHelper method is a recursive method that will implement the actual merge sort algorithm. The argument is a reference to the list of values that is to be sorted. this method will call the getLeftHalf and getRightHalf methods to divide a larger list, and call the merge method to merge two sorted sub-lists into one sorted list.
the getLeftHalf method will take as its argument, a reference to the list of values that is to be sorted. This method will return an IList
given an IList named list, of these values {1,2,3,4}, getLeftHalf(list) would return an IList
given an IList named list, of these values {3,6,2,5,4,1,7}, getLeftHalf(list) would return an IList
Note that for lists with an odd number of values (like {3,6,2,5,4,1,7}), the getLeftHalf method should return the smaller half of the list (i.e. {3,6,2} instead of {3,6,2,5}).
the getRightHalf method will take as its argument, a reference to the list of values that is to be sorted. This method will return an IList
given an IList named list, of these values {1,2,3,4}, getRightHalf(list) would return an IList
given an IList named list, of these values {3,6,2,5,4,1,7}, getRightHalf(list) would return an IList
Note that for lists with an odd number of values (like {3,6,2,5,4,1,7}), the getRightHalf method should return the larger half of the list (i.e. {5,4,1,7} instead of {4,1,7}).
the merge method will take two sorted lists as arguments. This method must create a new IList
given an IList named list1, of these values {2,4,6}, and an IList named list2, of these values {1,3,5}, merge(list1, list2) would return an IList
given an IList named list1, of these values {1,4,5,8}, and an IList named list2, of these values {2,3,4,7,9}, merge(list1, list2) would return an IList
public class MySorts { public static void mergesort(IList
private static IList IList leftHalf = mergesortHelper(leftHalf); rightHalf = mergesortHelper(rightHalf); return merge(leftHalf, rightHalf); } private static IList private static IList private static IList while (i while (i while (j return merged; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
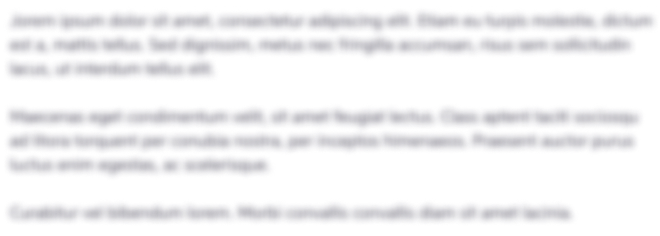
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started