Question
Implement a MIPS assembly language program that defines main, readArray, printArray, and swapElements procedures/functions. The readArray takes an array of integers as its parameter, reads
Implement a MIPS assembly language program that defines main, readArray, printArray, and swapElements procedures/functions. The readArray takes an array of integers as its parameter, reads in integers from a user to fill the array and also print each value as long as it is with the number of elements specified by the parameter "howMany" and "length". The printArray takes an array of integers as its parameter, prints each integer of the array. The swapElements procedure/function takes parameters of arrays of integers, and its length, and asks a user how many integers to read in and calls the readArray procedure/function twice to populate two arrays. The it compares each element of two arrays and it arranges their contents so that the integer of the array1 should be less than or equals to the integer of the array2 for the same index. (If they are out of order, then it swaps them.) Then it prints out the updated content of the two arrays, by calling the printArray procedure/function twice. The main procedure/function calls the swapElements procedure/function.
The following shows how it looks like in a C program:
//The readArray procedure reads integers from user input and store them in the array void readArray(int array[], int length, int howMany, int number) { int num, i = 0; while (i < howMany && i < length) { printf("Enter an integer: "); //read an integer from a user input and store it in num1 scanf("%d", &num); array[i] = num; i++; } printf(" The array %d content: ", number); i = 0; while (i < howMany && i < length) { printf("%d ", array[i]); i++; } return; } //The printArray procedure prints integers of the array void printArray(int array[], int length, int howMany, int number) { int i; printf(" Result array %d Content: ", number); i = 0; while (i < howMany && i < length) { printf("%d ", array[i]); i++; } return; } //The swapElements calls readArray procedure to populate two arrays, //then compares them and swaps elements, then print the result arrays. void swapElements(int array1[], int array2[], int length) { int i, min, max; int howMany, search, found; printf("Specify how many numbers should be stored in the arrays (at most 10): "); scanf("%d", &howMany); readArray(array1, length, howMany, 1); readArray(array2, length, howMany, 2); i = 0; while (i < howMany && i < length) { if (array1[i] < array2[i]) { min = array1[i]; max = array2[i]; } else { min = array2[i]; max = array1[i]; } array1[i] = min; array2[i] = max; i++; } printArray(array1, length, howMany, 1); printArray(array2, length, howMany, 2); return; } // The main calls the swapElements int main() { int arraysize = 10; int numbers1[arraysize], numbers2[arraysize]; swapElements(numbers1, numbers2, arraysize); return 0; }
The following is a sample output (user input is in bold):
Specify how many numbers should be stored in the arrays (at most 10): 8 Enter an integer: 1 Enter an integer: -12 Enter an integer: 53 Enter an integer: -4 Enter an integer: 5 Enter an integer: 32 Enter an integer: 1 Enter an integer: 7 The array 1 content: 1 -12 53 -4 5 32 1 7 Enter an integer: 1 Enter an integer: 7 Enter an integer: 1 Enter an integer: 5 Enter an integer: 1 Enter an integer: -5 Enter an integer: -2 Enter an integer: 3 The array 2 content: 1 7 1 5 1 -5 -2 3 Result array 1 Content: 1 -12 1 -4 1 -5 -2 3 Result array 2 Content: 1 7 53 5 5 32 1 7
Each procedure/function needs to have a header using the following format:
############################################################################ # Procedure/Function readArray # Description: ----- # parameters: $a0 = address of array # return value: $v0 = length # registers to be used: $s3 and $s4 will be used. ############################################################################
Step by Step Solution
There are 3 Steps involved in it
Step: 1
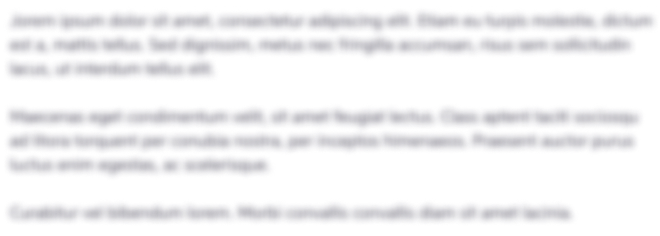
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started