Question
Implement a priority queue data structure. Thanks #include // Include to use NULL, otherwise use nullptr #include #include node.hpp template class PQ{ private: Node *head;
Implement a priority queue data structure. Thanks
#include
template
public: // desc: Inializes a new, empty queue PQ();
// desc: Adds new data to the queue // param: data The data to add to the list // param: priority The priority assigned to this node // returns: void void enqueue(T &data, int priority);
// desc: Removes the front element form the queue // returns: void void dequeue(void);
// desc: Removes the value found at the front of the queue // returns: The data found at the front of the queue T get_front(void);
// desc: Checks if the queue is empty or not // returns: true is the queue is empty, false if not bool isEmpty(void);
// desc: Clears the queue // returns: void void clear(void);
// desc: Returns the number of elements in the queue // returns: The number of elements in the queue int get_count(void); };
template
template
}
template
template
template
template
template
#pragma once #include
template
template
template
template
template
template
template
template
template
1 1 1 3 1 9 2 3 1 33 3 3 1 2 4 3 1 89 5 3 1 999 0 3 2 3 2 3 2 3 4 2 3 4 2 3 4 2 3 4 2 3 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
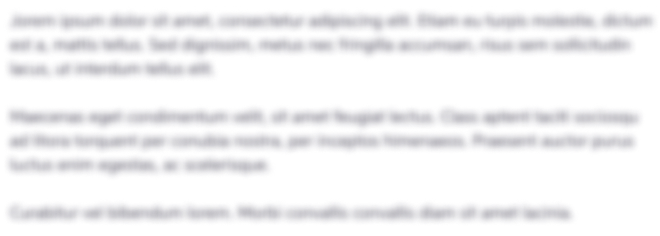
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started