Question
Implement a program that: reads a number of personal records (for example, using PERSON struct from the earlier lab) from the standard input, creates a
Implement a program that:
- reads a number of personal records (for example, using PERSON struct from the earlier lab) from the standard input,
- creates a database of personal records,
- allows for adding new entries to the database,
- allows for deleting entries from the database,
- includes functions to acquire a record of personal data, and
- includes functions to display (print) a single selected record from the database, and
- also allows for printing all records in the database.
NOTES:
- if name is longer than 40 characters it should be truncated,
- duplicates are allowed,
- when removing entry, remove the first found.
The database application must be implemented using the linked list functions provided in list.c and the accompanying list.h.There are several functions that need to be completed in the list package.
All functions related to the operations on the database must be in the file person.c. There is the companion file person.h for keeping the data structures and function declarations.
A test driver is implemented in the main.c.
You must not modify any of the code. Instead, add the code that implements the missing functionality as indicated by the TODO comments.
#include "list.h" void add(LIST **head, LIST **tail, void *data) { if (*tail == NULL) { *head = *tail = (LIST *) malloc(sizeof(LIST)); (*head)->data = data; (*head)->next = NULL; } else { (*tail)->next = (LIST *) malloc(sizeof(LIST)); *tail = (*tail)->next; (*tail)->data = data; (*tail)->next = NULL; } } void clearIteratively(LIST **head, LIST **tail) { if (*head == NULL) return; LIST *currNode = *head; LIST *nextNode = NULL; do { nextNode = currNode->next; if (currNode->data != NULL) free(currNode->data); free(currNode); currNode = nextNode; } while (currNode != NULL); *head = NULL; *tail = NULL; } void clearRecursively(LIST **currNode, LIST **tail) { if (*currNode == NULL) return; LIST *nextNode; // TODO Complete this function clearRecursively(&nextNode, tail); *currNode = NULL; *tail = NULL; } void delete(LIST **head, LIST **tail, void *data) { if (*head == NULL) return; if (data == NULL) return; if ((*head)->data == data) { LIST *newHead = (*head)->next; free((*head)->data); free(*head); *head = newHead; if (*head == NULL) *tail = NULL; return; } LIST *prevNode, *currNode; prevNode = (*head); currNode = (*head)->next; while (currNode != NULL) { // TODO Complete this function } }
#ifndef LIST_H_ #define LIST_H_ #include#include typedef struct list { void *data; struct list *next; } LIST; void add(LIST **head, LIST **tail, void *data); void clearIteratively(LIST **head, LIST **tail); void clearRecursively(LIST **head, LIST **tail); void delete(LIST **head, LIST **tail, void *data); #endif /* LIST_H_ */
#include "person.h" LIST *head = NULL, *tail = NULL; void inputPersonalData(PERSON *person) { // TODO Implement the function } void addPersonalDataToDatabase(PERSON *person) { // TODO Implement the function } void displayDatabase() { // TODO Implement the function } void displayPerson(PERSON *person) { // TODO Implement the function } PERSON *findPersonInDatabase(char *name) { // TODO Implement the function return NULL; // if not found } void removePersonFromDatabase(char *name) { // TODO Implement the function } void clearDatabase() { // TODO Implement the function }
#ifndef PERSON_H_ #define PERSON_H_ #include#include #include #include "list.h" typedef char NAME[41]; typedef struct date { int month; int day; int year; } DATE; typedef struct person { NAME name; int age; float height; DATE bday; } PERSON; void inputPersonalData(PERSON *person); void addPersonalDataToDatabase(PERSON *person); void displayDatabase(); void clearDatabase(); void displayPerson(PERSON *person); PERSON *findPersonInDatabase(char *name); void removePersonFromDatabase(char *name); #endif /* PERSON_H_ */
#include#include #include #include "person.h" #define DEF_NUM 1 int main(void) { PERSON *person; int num; puts("Enter the initial number of records:"); if (scanf("%d", &num) < 1) num = DEF_NUM; while (num-- > 0) { person = (PERSON *) malloc(sizeof(PERSON)); inputPersonalData(person); addPersonalDataToDatabase(person); } displayDatabase(); puts(" --> Searching database for Maya"); PERSON *maya = findPersonInDatabase("Maya"); if (maya == NULL) puts("Maya not found"); else { displayPerson(maya); puts(" --> Removing Maya from database"); removePersonFromDatabase("Maya"); } displayDatabase(); // added to test finding and removing the last element puts(" --> Searching database for Frank"); PERSON *frank = findPersonInDatabase("Frank"); if (frank == NULL) puts("Frank not found"); else { displayPerson(frank); puts(" --> Removing Frank from database"); removePersonFromDatabase("Frank"); } displayDatabase(); puts(" --> Removing Miro from database"); removePersonFromDatabase("Miro"); displayDatabase(); puts(" --> Adding new record to database"); person = (PERSON *) malloc(sizeof(PERSON)); inputPersonalData(person); addPersonalDataToDatabase(person); displayDatabase(); puts(" --> Clearing database"); clearDatabase(); displayDatabase(); puts(" --> Adding new record to database"); person = (PERSON *) malloc(sizeof(PERSON)); inputPersonalData(person); addPersonalDataToDatabase(person); displayDatabase(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
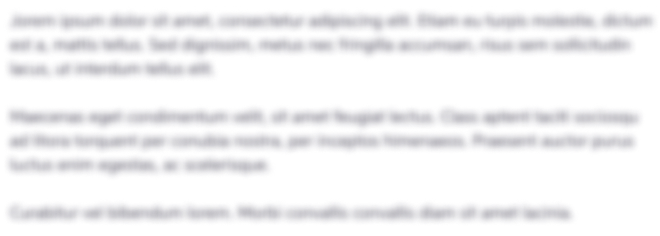
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started