Question
Implement a video manager. You can add videos, create playlists, generate HTML for playlists, etc. In the code distribution we have included a driver (SampleDriver.java)
Implement a video manager. You can add videos, create playlists, generate HTML for playlists, etc. In the code distribution we have included a driver (SampleDriver.java) and expected output (SampleDriverOutput.txt) that illustrates some of the functionality provided by the system.
Fill in the empty methods to add videos, create playlist and generate HTML for playlist in Playlist.Java and also Video.Java
- tubeVideosManager A package where you will find shells for classes you need to implement.
- tests A package where you will find public tests and a shell file for student tests.
- text files/directories Files providing input for public tests, and support directories will be found in the main project folder. Expected outputs for public tests will be found in the expectedResults folder. Results generated by your code when you run your public tests will be placed in the results folder.
Method Descriptions are below
Genre.java
public enum Genre { Comedy, Educational, Documentary, Music, FilmAnimation }
Playlist.java
public class Playlist { private String name; private ArrayList videoTitles;
/** * Initializes playlist with the specified name and creates an empty ArrayList. If the parameter is null or is a blank string (according to String class isBlank() method) the method will throw an IllegalArgumentException (with any message) and perform no processing. * @param name */ public Playlist(String name) { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for name * @return name string */ public String getName() { throw new UnsupportedOperationException("Implement this method"); }
/** * Initializes the current object so changes to the current object will not affect the parameter object. * @param playlist */ public Playlist(Playlist playlist) { throw new UnsupportedOperationException("Implement this method"); }
/** * Provided; please don't modify. toString for class * @return string with object info */ public String toString() { String answer = "Playlist Name: " + name + " ";
answer += "VideoTitles: " + videoTitles;
return answer; }
/** * Adds the title to the Arraylist storing titles. We can add the same video title several times. If the parameter is null or is a blank string (according to String class isBlank() method) the method will throw an IllegalArgumentException (with any message) and perform no processing. * @param videoTitle * @return true if title is added; false otherwise */ public boolean addToPlaylist(String videoTitle) { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for the ArrayList of titles. You must avoid privacy leaks. * @return ArrayList with titles */ public ArrayList getPlaylistVideosTitles() { throw new UnsupportedOperationException("Implement this method"); }
/** * Removes all instances of the title parameter from the ArrayList of titles. If the parameter is null or is a blank string (according to String class isBlank() method) the method will throw an IllegalArgumentException (with any message) and perform no processing. * @param videoTitle * @return true if the ArrayList (videoTitles) was changed as a result of * calling this method and false otherwise. */ public boolean removeFromPlaylistAll(String videoTitle) { throw new UnsupportedOperationException("Implement this method"); }
/** * Randomizes the list of titles using a random parameter and Collections.shuffle. If the parameter is null, call Collections.shuffle with just the ArrayList. * @param random */ public void shuffleVideoTitles(Random random) { throw new UnsupportedOperationException("Implement this method"); } }
Video.java
public class Video implements Comparable
{ private String title, url; private int durationInMinutes; private Genre videoGenre; private ArrayList comments;
/** * Initializes a video object. If any parameter is null or if a string parameter is a blank (according to String class isBlank() method), the method will throw an IllegalArgumentException (with any message) and perform no processing. Also the same exception will be thrown if the duration is zero or negative. * @param title * @param url * @param durationInMinutes * @param videoGenre */ public Video(String title, String url, int durationInMinutes, Genre videoGenre) { throw new UnsupportedOperationException("Implement this method"); }
/** * Initializes the Video object so changes to the parameter do not affect the current object. Your implementation must be efficient (avoid any unnecessary * copies). * @param video */ public Video(Video video) { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for title * @return title string */ public String getTitle() { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for url * @return url string */ public String getUrl() { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for duration * @return duration */ public int getDurationInMinutes() { throw new UnsupportedOperationException("Implement this method"); }
/** * Get method for video genre * @return string with genre */ public Genre getGenre() { throw new UnsupportedOperationException("Implement this method"); }
/** * Provided; please don't modify. toString for class * @return string with object info */ public String toString() { String answer = "Title: " + "\"" + title + "\" ";
answer += "Url: " + url + " "; answer += "Duration (minutes): " + durationInMinutes + " "; answer += "Genre: " + videoGenre + " ";
return answer; }
/** * Adds specified comments to the video. If the parameter is null or is a blank string (according to String class isBlank() method) the method will throw an IllegalArgumentException (with any message) and perform no processing. * @param comments * @return true if comments added; false otherwise */ public boolean addComments(String comments) { throw new UnsupportedOperationException("Implement this method"); }
/** * Returns copy so changes to the copy does not affect the original. Your implementation must be efficient (avoid any unnecessary copies). * @return ArrayList of strings */ public ArrayList getComments() { throw new UnsupportedOperationException("Implement this method"); }
/** * Videos will be compared using title. If we were to sort an ArrayList of * Videos, they will appear in lexicographical (alphabetical) order (e.g, "A","B", "C"). * @return negative, 0, or positive value */ public int compareTo(Video video) { throw new UnsupportedOperationException("Implement this method"); }
/** * Two Video objects are considered equal if they have the same title. Implement * the method using the instanceof operator rather than using getClass(). * @return true if objects are considered equal; false otherwise */ @Override public boolean equals(Object obj) { throw new UnsupportedOperationException("Implement this method"); } }
SampleDriver.java
public class SampleDriver {
public static void main(String[] args) { String output = "";
/* Creating video and adding comments */ output += "============== One ============== "; String title = "How to Draw in Java Tutorial"; String url = "https://www.youtube.com/embed/ifVf9ejuFWI"; int durationInMinutes = 17; Genre genre = Genre.Educational; Video video = new Video(title, url, durationInMinutes, genre); video.addComments("Nice video"); video.addComments("Recommended"); output += video; output += "Comments: " + video.getComments() + " ";
output += "============== Two ============== "; /* Creating playlist and adding video */ Playlist playlist = new Playlist("Favorites"); playlist.addToPlaylist(title); playlist.addToPlaylist(title); // We can add title multiple times playlist.addToPlaylist("Hello"); output += playlist + " "; playlist.removeFromPlaylistAll(title); output += "After remove" + " "; output += playlist + " ";
output += "============== Three ============== "; TubeVideosManager tubeVideosManager = new TubeVideosManager();
/* Adding first video */ tubeVideosManager.addVideoToDB(title, url, durationInMinutes, genre);
/* Adding second video */ title = "Git & GitHub Crash Course for Beginners"; url = "https://www.youtube.com/embed/SWYqp7iY_Tc"; durationInMinutes = 33; genre = Genre.Educational; tubeVideosManager.addVideoToDB(title, url, durationInMinutes, genre);
/* Getting videos */ ArrayList
videos = tubeVideosManager.getAllVideosInDB(); output += videos + " ";
output += "============== Cuatro ============== "; /* Loading Videos */ tubeVideosManager = new TubeVideosManager(); boolean printFeedback = false; tubeVideosManager.loadVideosToDBFromFile("videosInfoSet2.txt", printFeedback); videos = tubeVideosManager.getAllVideosInDB(); output += videos + " "; String playlistName = "ToWatch"; tubeVideosManager.addPlaylist(playlistName); title = "One Day More"; tubeVideosManager.addVideoToPlaylist(title, playlistName); playlist = tubeVideosManager.getPlaylist(playlistName); output += "Displaying Playlist " + playlist + " "; output += tubeVideosManager.getStats() + " ";
System.out.println(output); } }
TubeVideosManager.java Thank you for your help if you are confuse you can comment to let me know i will thumbs up. Here is my email taphlawolf at g mail .com i can send any file you need to you. some of the files i cant provide because of limits line space. Can You comment a email address for me to send the SampledriverOutput.txt file. I also would like to ask future question as well if i need help
Step by Step Solution
There are 3 Steps involved in it
Step: 1
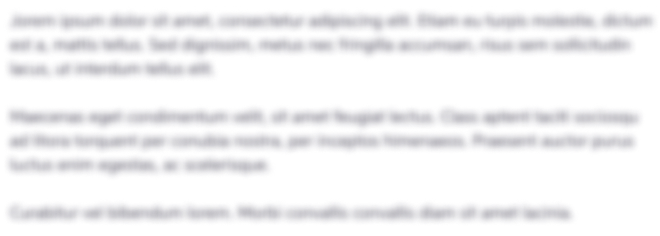
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started