Question
Implement the cpp program faclity.cpp that uses the provided class facility.h and can be tested with the provided program testFacility.cpp Instrutions of facility.cpp are provided
Implement the cpp program faclity.cpp that uses the provided class facility.h and can be tested with the provided program testFacility.cpp
Instrutions of facility.cpp are provided below--
Facility(string s)
The constructor takes a single string argument. The argument s contains a full line read from theFacilities.txt file. The constructor should initialize the data members of Facility by selecting the appropriate substrings from the argument. The latitude and longitude fields should be converted to double values using the convert_latitude and convert_longitude member functions. The sign of the latitude_ and longitude_data members should be determined by checking whether the latitude and longitude fields end with N or S, and E or W respectively.
string site_number(void) const
This function returns the facility's site number.
string type(void) const
This function returns the facility's type.
string code(void) const
This function returns the facility's code.
string name(void) const
This function returns the facility's name.
double latitude(void) const
This function returns the latitude of the facility in degrees decimal. Latitudes in the southern hemisphere are negative numbers.
double longitude(void) const
This function returns the longitude of the facility in degrees decimal. Longitudes in the western hemisphere are negative numbers.
double distance(double lat, double lon) const
This function returns the distance in nautical miles between the facility and the position defined by (lat,lon) in degrees decimal. The implementation of this function uses the gcdistance function provided in filesgcdistance.h and gcdistance.cpp .
double convert_latitude(string s) const
This function converts the string s representing a latitude in seconds decimal to a double value in degrees decimal. One degree is 3600 seconds. The sign of the result is positive if the string s ends with N and negative if it ends with S . For example, the latitude represented by the string 135427.7000N should be converted to the value 37.6188
double convert_longitude(string s) const
This function converts the string s representing a longitude in seconds decimal to a double
value in degrees decimal. One degree is 3600 seconds. The sign of the result is positive if the string s ends with E and negative if it ends with W . For example, the longitude represented by the string 440551.5000Wshould be converted to the value -122.3754 .
//
// Facility.h
//
#ifndef FACILITY_H
#define FACILITY_H
#include
class Facility
{
public:
Facility(std::string s);
std::string site_number(void) const;
std::string type(void) const;
std::string code(void) const;
std::string name(void) const;
double latitude(void) const;
double longitude(void) const;
double distance(double lat, double lon) const;
private:
const std::string site_number_;
const std::string type_;
const std::string code_;
const std::string name_;
const double latitude_, longitude_;
double convert_latitude(std::string s) const;
double convert_longitude(std::string s) const;
};
#endif
//
// testFacility.cpp
//
#include "Facility.h"
#include
#include
using namespace std;
int main()
{
string line;
getline(cin,line);
Facility f(line);
cout << f.site_number() << " " << f.type() << " " << f.code() << " "
<< f.name() << " ";
cout << setw(12) << setprecision(4) << fixed << f.latitude() << " "
<< setw(12) << setprecision(4) << fixed << f.longitude() << " ";
cout << f.distance(40,-100) << endl;
}
example of input--
02187.*A AIRPORT SFO 01/05/2017AWPSFO CACALIFORNIA SAN MATEO CASAN FRANCISCO SAN FRANCISCO INTL PUPUCITY & COUNTY OF SAN FRANCISCO PO BOX 8097 SAN FRANCISCO, CA 94128 650-821-5000JOHN L. MARTIN PO BOX 8097 SAN FRANCISCO, CA 94128 650-821-500037-37-07.7000N 135427.7000N122-22-31.5000W440551.5000WE 13.1S14E2015 SAN FRANCISCO 08SE 5207ZOA ZCOOAKLAND NOAK OAKLAND 1-800-WX-BRIEF SFO Y04/1940O I E S 05/1973 NGPRY NO OBJECTION NYNYF F04292016 100LLA MAJORMAJORHIGH/LOW SS-SR Y122.950 N CG Y 001000009001 004 354151 05955600794701368600242212/12/20153RD PARTY SURVEY10/22/20143RD PARTY SURVEY10/22/2014 HGR,TIE AFRT,AVNCS,CARGO,CHTR,INSTR Y-LKSFO
example of output--
02187.*A AIRPORT SFO SAN FRANCISCO INTL 37.6188 -122.3754 1053.7350
Step by Step Solution
There are 3 Steps involved in it
Step: 1
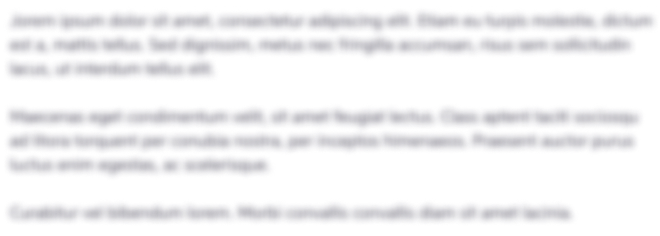
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started