Question
Implement the following 2 non-static classes. Each class must be in its own C# file. If the following two classes are not each within their
Implement the following 2 non-static classes. Each class must be in its own C# file. If the following two classes are not each within their own C# file, you will lose points.
1) Account class
Implement private fields with public setters and getters in the Account class - use appropriate data types as discussed in class
AccountNumber - should allow only for 20 digits, including leading zeros, but NO LETTERS - example value: "00045678493857683928". Your setter should set empty if validation fails. - "Numbers" allowing for leading zeros are really just strings. If less than 20 digits or more than 20 digits set to empty string
AccountType - "Savings" or "Checking" - no other types or string should be accepted in this setter. If I pass "MoneyMarket" the setter should default this property to empty. Casing can be ignored - for example, "SAVINGS" and "savings" can be accepted. I will accept strings but can also be enum.
AccountBalance - How much money is in the account. Balance should never be less than zero. If your setter allows for setting a value less than zero you will lose points. You should check the value first, and if it is less than zero, just set the balance to zero.
Implement the following public methods (behaviors) in the Account class
Default constructor - no arguments but the properties are initialized to non-null values.
Parameterized constructor - arguments for all properties can be passed (AccountNumber, AccountType, AccountBalance)
ToString - return a formatted string with labels for each of the following: account number, account type, and balance. AccountBalance should be formatted with a $ and decimals. This should be in a single string on 1 line.
- ex: "Account number: 11111111111111111111 Account type: Checking Account Balance: $500.00"
Equals - accept an object as a parameter, cast it to an Account type, and compare the following
- Whether the Account number, Account type, and Account balance match. If they match return true, else return false
- Ignore mixed casing on Account type - Use ToUpper or ToLower to ignore casing
"CHECKING" should match "checking", etc.
2) Bank class
Implement private fields with public setters and getters in the Bank class - use appropriate data types as discussed in class.
Name - example value: "FirstUSA Bank"
Address - example value: "123 Main St. Cleveland, OH. 44177."
PhoneNumber - example value: "216-999-9999". A phone number value should NOT be settable if the format of the string is anything other than ###-###-####. You will need to check the format before allowing a setting of the value. For example, a value of "123" or "2169999999" should not be settable - it MUST be formatted as "###-###-####"
- Set value to empty if the validation rule fails - you may want to consider implementing the validation as a private helper method, like private bool IsPhoneNumberValid(string phoneNumber) {}
Accounts (use an array with a max size of 10) -
- NOTE: The assignment calls for a max size of 10. If you choose to use any other collection type, you must introduce a constraint to only hold 10, and it must also store the Accounts in sequential order (i.e. order in which they were added), just like a traditional array declared with brackets (i.e. []).
Implement the following public methods (behaviors) in the Bank class
Default constructor - no arguments but the properties (private field values) are initialized to non-null values.
NOTE: The Accounts array itself should not be null after initialization, but the individual instances of Account can be null when the property is initialized.
Parameterized constructor - all property arguments can be passed (name, address, phone number, and account list) - NOTE: The phone number validation is still needed here.
ToString - return a formatted string with labels for each of the following: the Name, address, phone number, and number of non-null accounts
For example: "Bank Name: FirstUSA Bank PhoneNumber: 444-555-6666 Address: 123 Main St. Cleveland, OH 44060 Number Of Accounts: 3" - If you only have 3 non-null accounts, you have 3 accounts. Even though your array holds 10, if you only added 3 accounts, you should only show 3 in your ToString()
Equals - returns true or false - accept an object, cast it to a Bank type, and compare the following
Whether the bank names match, regardless of mixed casing (hint, try comparing using ToUpper or ToLower)
- EX: "FirstUSA Bank" should be equal when compared to "firstusa BANK"
- Whether the addresses match, regardless of mixed casing
- Whether the accounts match in sequential order - NOTE: the accounts will be implementing their own Equals method which you will need to use inside the Bank's Equals method. Do not sort Accounts arrays before comparing.
- Recall that equal compares an object parameter to the class instance itself
- Pseudocode:
Compare non-account info (name/phone/address)
If they don't match return false
If those match, compare each bank's accounts:
for (int i = 0; i < Accounts.Length; i++)
{
if (!Accounts[i].Equals(accountParam[i])) return false; // ! is not operator
}
Otherwise return true, everything matches.
AddAccount - Accept 1 account as a parameter. Make sure parameter is not null - if null do not add the account. Add a non-null instance of account to your Accounts property, to the last available (null) index of the Accounts array. An account should not be added if (a) any account matches using Account .Equals method (see below) OR your account array is already maxed out at 10 entries.
The AddAccount method should be a bool return type. Return a true if the account was added and a false if it was not. You will need to write a loop and check each index to see if the value in the accounts array index is null or not null. For example (if Accounts[0] == null then set Accounts[0] = non-null account instance parameter)
RemoveAccount - Accept 1 account as a parameter. Ensure parameter is not null before proceeding. Find the index of the account that matches.
Use .Equals method on account and a for loop (or some other loop type of your choice). If the Account instance parameter matches, set the index value to null. The RemoveAccount should be a bool type. Return true if an account was removed, otherwise return false . If parameter was null, or no account matched and removal did not occur, return false.
Implement the following in Program.cs
Rename Program.cs to BankApplication.cs - retain the Main() method as your entry point
This should remain a static class.
In Main method:
Instantiate a new Bank instance.
Then, using a loop, Create 10 instances of account and add them to the bank using AddAccount.
With each loop iteration, alter the last digit of the account number so that each account number is unique.
HINT: Here is one way to do it but there are others
for (int i = 0; i < 10; i++)
{
string accountNumber = "0000000000000000000" + i.ToString(); //19 zeroes and a last digit
Account a = new Account();
a.AccountNumber = accountNumber;
//set other properties then add to the bank
bank.AddAccount(a);
}
OTHER HINT: I would implement the above as a method because you will have to do this twice
Remove 2 of those accounts using RemoveAccount. You should be able to do so by simply calling RemoveAccount and passing a non-null instance of the bank's Account array as a parameter.
Print ToString method of Bank to the console using Console.WriteLine(). After printing out Bank ToString() method, iterate through the accounts in Bank using a loop, and print ToString() of each account in the bank using Console.WriteLine().
Create a second instance of Bank. Modify enough of its public properties to ensure it won't match the first bank using Bank .Equals method. Print conditional string result of Equals method on the two banks using Console.WriteLine(). It should be false.
Pseudocode example: If (bank1.Equals(bank2) Console.WriteLine("The banks match") else Console.WriteLine("The banks don't match");
Create a third bank instance using its parameterized constructor. In other words, pass parameters to its constructor Create a new, unique account using its default constructor and add it to the third bank.
Call .RemoveAccount on first bank instance using the new, unique account above - should return false
Print result of ToString() on the third bank using Console.WriteLine().
Finally, ensure graceful exit of your program by requiring enter key to be typed to exit (Console.ReadLine())
Step by Step Solution
There are 3 Steps involved in it
Step: 1
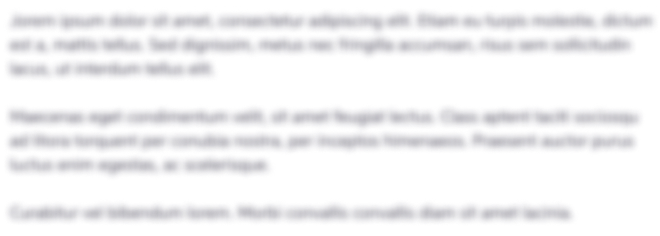
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started