Question
Implement the following functions inside the LinkedBag: -add a value at the end of the list -eliminate a value from the list without losing the
Implement the following functions inside the LinkedBag:
-add a value at the end of the list
-eliminate a value from the list without losing the original order
-print the contents of the array
-in the main program, show how the copy constructor works
//////////////////////////LinkedBag.cpp////////////////////////////////////
#include "LinkedBag.h"
#include "Node.h"
#include
template
LinkedBag
{
} // end default constructor
template
LinkedBag
{
itemCount = aBag.itemCount;
Node
if (origChainPtr == nullptr)
headPtr = nullptr; // Original bag is empty
else
{
// Copy first node
headPtr = new Node
headPtr->setItem(origChainPtr->getItem());
// Copy remaining nodes
Node
origChainPtr = origChainPtr->getNext(); // Advance original-chain pointer
while (origChainPtr != nullptr)
{
// Get next item from original chain
ItemType nextItem = origChainPtr->getItem();
// Create a new node containing the next item
Node
// Link new node to end of new chain
newChainPtr->setNext(newNodePtr);
// Advance pointer to new last node
newChainPtr = newChainPtr->getNext();
// Advance original-chain pointer
origChainPtr = origChainPtr->getNext();
} // end while
newChainPtr->setNext(nullptr); // Flag end of chain
} // end if
} // end copy constructor
template
LinkedBag
{
clear();
} // end destructor
template
bool LinkedBag
{
return itemCount == 0;
} // end isEmpty
template
int LinkedBag
{
return itemCount;
} // end getCurrentSize
template
bool LinkedBag
{
// Add to beginning of chain: new node references rest of chain;
// (headPtr is null if chain is empty)
Node
nextNodePtr->setItem(newEntry);
nextNodePtr->setNext(headPtr); // New node points to chain
// Node
headPtr = nextNodePtr; // New node is now first node
itemCount++;
return true;
} // end add
template
vector
{
vector
Node
int counter = 0;
while ((curPtr != nullptr) && (counter < itemCount))
{
bagContents.push_back(curPtr->getItem());
curPtr = curPtr->getNext();
counter++;
} // end while
return bagContents;
} // end toVector
template
bool LinkedBag
{
Node
bool canRemoveItem = !isEmpty() && (entryNodePtr != nullptr);
if (canRemoveItem)
{
// Copy data from first node to located node
entryNodePtr->setItem(headPtr->getItem());
// Delete first node
Node
headPtr = headPtr->getNext();
// Return node to the system
nodeToDeletePtr->setNext(nullptr);
delete nodeToDeletePtr;
nodeToDeletePtr = nullptr;
itemCount--;
} // end if
return canRemoveItem;
} // end remove
template
void LinkedBag
{
Node
while (headPtr != nullptr)
{
headPtr = headPtr->getNext();
// Return node to the system
nodeToDeletePtr->setNext(nullptr);
delete nodeToDeletePtr;
nodeToDeletePtr = headPtr;
} // end while
// headPtr is nullptr; nodeToDeletePtr is nullptr
itemCount = 0;
} // end clear
template
int LinkedBag
{
int frequency = 0;
int counter = 0;
Node
while ((curPtr != nullptr) && (counter < itemCount))
{
if (anEntry == curPtr->getItem())
{
frequency++;
} // end if
counter++;
curPtr = curPtr->getNext();
} // end while
return frequency;
} // end getFrequencyOf
template
bool LinkedBag
{
return (getPointerTo(anEntry) != nullptr);
} // end contains
// private
// Returns either a pointer to the node containing a given entry
// or the null pointer if the entry is not in the bag.
template
Node
{
bool found = false;
Node
while (!found && (curPtr != nullptr))
{
if (anEntry == curPtr->getItem())
found = true;
else
curPtr = curPtr->getNext();
} // end while
return curPtr;
} // end getPointerTo
//////////////////////LinkedBag.h///////////////////////////////////////
#ifndef _LINKED_BAG #define _LINKED_BAG
#include "BagInterface.h" #include "Node.h"
template
#include "LinkedBag.cpp" #endif
////////////////////////////////////////Node.h///////////////////////////////////////
#ifndef _NODE #define _NODE
template
#include "Node.cpp" #endif
//////////////////////////Node.cpp////////////////////////////////////////
#include "Node.h" #include
template
template
template
template
template
template
template
/////////////////////////////////////BagInterface.h/////////////////////////////////////
#ifndef _BAG_INTERFACE #define _BAG_INTERFACE
#include
template
Step by Step Solution
There are 3 Steps involved in it
Step: 1
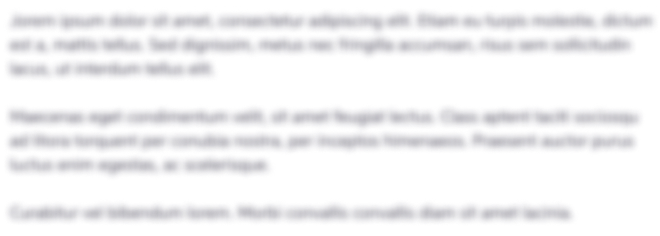
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started