Question
Implement the function: void reverseQueue(QueType queue) // QueType.h #ifndef QUETYPE_H #define QUETYPE_H #include ItemType.h class FullQueue { }; class EmptyQue { }; typedef char ItemType;
Implement the function: void reverseQueue(QueType queue)
// QueType.h
#ifndef QUETYPE_H #define QUETYPE_H #include "ItemType.h"
class FullQueue {
};
class EmptyQue {
};
typedef char ItemType;
class QueType { public: QueType(int max); QueType(); ~QueType(); void MakeEmpty(); bool IsEmpty() const; bool IsFull() const; void Enqueue(ItemType newItem); void Dequeue(ItemType &newItem); int getLength(QueType queue); ItemType Front() const; private: int front; int rear; ItemType *items; int maxQue; int length; };
#endif // QUETYPE_H
// QueType.cpp
#include "QueType.h"
QueType::QueType(int max) { maxQue = max + 1; front = maxQue - 1; rear = maxQue - 1; items = new ItemType[maxQue]; }
QueType::QueType() { maxQue = 9; front = maxQue - 1; rear = maxQue - 1; items = new ItemType[maxQue]; }
QueType::~QueType() { delete [] items; }
void QueType::MakeEmpty() { front = maxQue - 1; rear = maxQue - 1; }
bool QueType::IsEmpty() const { return (rear == front); }
bool QueType::IsFull() const { return ( (rear + 1) % maxQue == front); }
void QueType::Enqueue(ItemType newItem) { if(IsFull()) throw FullQueue(); else { rear = (rear + 1) % maxQue; items[rear] = newItem; //length++; } }
void QueType::Dequeue(ItemType &item) { if(IsEmpty()) throw EmptyQue(); else { front = (front + 1) % maxQue; items = items[front]; } }
ItemType QueType::Front() const { if(IsEmpty()) throw EmptyQue(); else { return items[front]; } }
// ItemType.h
#ifndef ITEMTYPE_H #define ITEMTYPE_H
class ItemType { public: ItemType(); void Initialize(int number); private: int value; };
#endif // ITEMTYPE_H
// ItemType.cpp
#include "ItemType.h"
ItemType::ItemType() { value = 0; }
void ItemType::Initialize(int number) { value = number; }
// StackType.h
#include "ItemType.h"
class FullStack {
};
class EmptyStack {
};
class StackType { public: StackType(int max); StackType(); bool isFull() const; bool isEmpty() const; void Push(ItemType item); void Pop(); ItemType Top() const; ~StackType(); private: int top; int maxStack; ItemType *items; };
// StackType.cpp
#include "StackType.h"
StackType::StackType(int max) { maxStack = max; top = -1; items = new ItemType[maxStack]; }
StackType::StackType() { maxStack = 10; top = -1; items = new ItemType[maxStack]; }
bool StackType::isFull() const { return (top == maxStack - 1); }
StackType::~StackType() { delete [] items; }
void StackType::Pop() { if(isEmpty()) throw EmptyStack();
top--; }
ItemType StackType::Top() const { if(isEmpty()) throw EmptyStack();
return items[top]; }
void StackType::Push(ItemType newItem) { if(isFull()) throw FullStack();
top++; items[top] = newItem; }
bool StackType::isEmpty() const { return (top == -1); }
// driver file
#include "QueType.h" #include "StackType.h" #include
void Print(QueType &); void reverseQue(QueType &);
int main() { QueType q; ItemType i;
// Put 1 into the queue i.Initialize(1); q.Enqueue(i);
// Put 2 into the queue i.Initialize(2); q.Enqueue(i);
// Put 3 into the queue i.Initialize(3); q.Enqueue(i);
// Put 4 into the queue i.Initialize(4); q.Enqueue(i);
// Put 5 into the queue i.Initialize(5); q.Enqueue(i);
// Put 6 into the queue i.Initialize(6); q.Enqueue(i);
// Put 7 into the queue i.Initialize(7); q.Enqueue(i);
// Put 8 into the queue i.Initialize(8); q.Enqueue(i);
// Put 9 into the queue i.Initialize(9); q.Enqueue(i);
cout << "Printing the data in the queue. ";
Print(queue);
cout << "Printing the data in the queue in reverse. ";
reverseQueue(queue);
Print(queue); }
void Print(QueType &queue) { while(!queue.IsEmpty()) { cout << queue.Front().value << " "; queue.Dequeue() } }
void reverseQue(QueType &queue) { stack tempStack; while(!queue.IsEmpty()) { tempStack.Push(queue.Front()); queue.Dequeue(); } while(!tempStack.isEmpty()) { queue.Enqueue(tempStack.Top()); tempStack.Pop(); } }
How to finish this to get an output below?
Printing data in the queue.
1 2 3 4 5 6 7 8 9
Printing data in the queue in reverse.
9 8 7 6 5 4 3 2 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
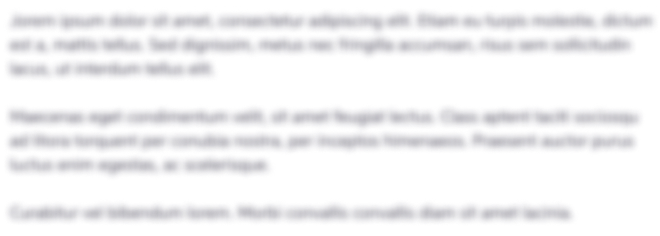
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started