Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Implement the HeapSort algorithm based on the heap in the skeleton of the class MaxHeap. The heap must be created using bottom - up construction
Implement the HeapSort algorithm based on the heap in the skeleton of the class MaxHeap. The heap must be created
using bottomup construction approach as described in lecture and exercise and inplace without using an additional data
structure Make sure to use the slightly different template of MaxHeap compared to assignment
The following methods shall be implemented:
class MaxHeap:
def initself list:
# Creates a bottomup maxheap inplace from the input list of numbers.
def containsself val:
# Tests if an item val is contained in the heap. Do not search the array sequentially, but use the heap properties.
def sortself:
# This method sorts the numbers inplace using the maxheap data structure in ascending order. a The MaxHeap constructor should create a valid maxheap using inplace bottomup construction.
b The method contains should return true, if the element val is found in the heap duplicates allowed Use the properties
of the maxheap for efficient implementation and do not search the array sequentially!
c The sort method should implement the HeapSort algorithm in ascending order, using the created maxheap by repeated
removal of the top element inplace. Dont use intermediate data structures!
You can use private help methods for your implementation. And this is the skeleton: class MaxHeap:
def initself inputarray:
@param inputarray from which the heap should be created
@raises ValueError if list is None.
Creates a bottomup max heap in place.
self.heap None
self.size
# TODO
def getheapself:
# helper function for testing, do not change
return self.heap
def getsizeself:
@return size of the max heap
return self.size
def containsself val:
@param val to check if it is contained in the max heap
@return True if val is contained in the heap else False
@raises ValueError if val is None.
Tests if an item val is contained in the heap. Does not search the entire array sequentially, but uses the
properties of a heap.
# TODO
def sortself:
This method sorts ascending the list inplace using HeapSort, eg
# TODO
def removemaxself:
Removes and returns the maximum element of the heap
@return maximum element of the heap or None if heap is empty
# TODO
Step by Step Solution
There are 3 Steps involved in it
Step: 1
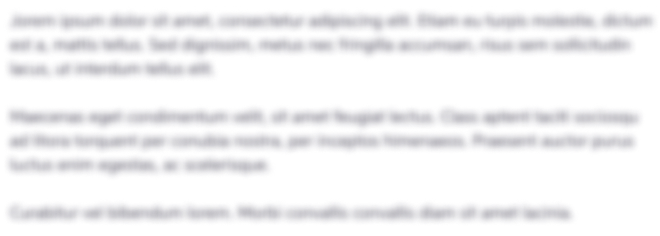
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started