Question
Implement the TODO methods in JAVA. (Task : Implement map using a sorted List) CODE: import java.util.List; import java.util.ArrayList; public class SortedListMap implements Map {
Implement the TODO methods in JAVA. (Task : Implement map using a sorted List)
CODE:
import java.util.List; import java.util.ArrayList;
public class SortedListMap
/** * Inserts an entry into the map with the given key and value. * * @param k * a key * @param v * a value */
@Override public void put(K key, V value) { //TODO } /** * Removes the value associated with the given key if any, and returns it. * * @param k * a key * @return the removed value (or null if none present) */
@Override public V remove(K key) { //TODO return null; } // Returns index of entry with key, or -1 if not found private int binarySearch(K key) { int min = 0; int max = entries.size(); while (min < max) { int mid = (max + min)/2; K midKey = entries.get(mid).key; if (midKey.equals(key)) { return mid; } else if (midKey.compareTo(key) < 0) { // key after midKey min = mid + 1; } else { // key before midKey max = mid; } } // not found return -1; } public static void main(String[] args) { System.out.println("Running the main method in SortedListMap"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
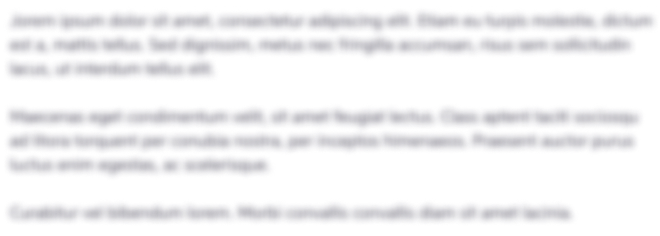
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started