Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import javafx.scene.paint.Color; public class GameBoard { public static final int WIDTH = 6; public static final int HEIGHT = 6; public static final int MAX_GAME_PIECES
import javafx.scene.paint.Color; public class GameBoard { public static final int WIDTH = 6; public static final int HEIGHT = 6; public static final int MAX_GAME_PIECES = 15; private GamePiece[] gamePieces; private int numGamePieces; private boolean completed; public GameBoard(int numPieces) { gamePieces = new GamePiece[numPieces]; numGamePieces = 0; completed = false; } public static GameBoard board1() { GameBoard b = new GameBoard(2); b.add(new VerticalGamePiece(2, Color.YELLOW, 5, 0)); b.add(new GoalPiece(1, 2)); return b; } public static GameBoard board2() { GameBoard b = new GameBoard(8); b.add(new GoalPiece(1, 2)); b.add(new HorizontalGamePiece(2, Color.LIGHTGREEN, 0, 0)); b.add(new HorizontalGamePiece(2, Color.LIGHTBLUE, 4, 4)); b.add(new HorizontalGamePiece(3, Color.GREEN, 2, 5)); b.add(new VerticalGamePiece(3, Color.YELLOW, 5, 0)); b.add(new VerticalGamePiece(3, Color.PURPLE, 0, 1)); b.add(new VerticalGamePiece(3, Color.BLUE, 3, 1)); b.add(new VerticalGamePiece(2, Color.ORANGE, 0, 4)); return b; } public static GameBoard board3() { GameBoard b = new GameBoard(9); b.add(new GoalPiece(1, 2)); b.add(new HorizontalGamePiece(2, Color.PINK, 3, 4)); b.add(new HorizontalGamePiece(2, Color.PURPLE, 0, 5)); b.add(new VerticalGamePiece(3, Color.GOLD, 0, 0)); b.add(new VerticalGamePiece(3, Color.VIOLET, 3, 0)); b.add(new VerticalGamePiece(3, Color.BLUE, 5, 2)); b.add(new VerticalGamePiece(2, Color.GREEN, 5, 0)); b.add(new VerticalGamePiece(2, Color.ORANGE, 4, 2)); b.add(new VerticalGamePiece(2, Color.LIGHTBLUE, 2, 4)); return b; } public static GameBoard board4() { GameBoard b = new GameBoard(13); b.add(new GoalPiece(2, 2)); b.add(new HorizontalGamePiece(3, Color.BLUE, 0, 0)); b.add(new HorizontalGamePiece(2, Color.PINK, 1, 1)); b.add(new HorizontalGamePiece(2, Color.LIGHTGREEN, 0, 3)); b.add(new HorizontalGamePiece(2, Color.YELLOW, 2, 5)); b.add(new HorizontalGamePiece(2, Color.BROWN, 4, 4)); b.add(new HorizontalGamePiece(2, Color.GRAY, 4, 5)); b.add(new VerticalGamePiece(3, Color.GOLD, 4, 0)); b.add(new VerticalGamePiece(2, Color.SADDLEBROWN, 3, 0)); b.add(new VerticalGamePiece(2, Color.GREEN, 5, 0)); b.add(new VerticalGamePiece(2, Color.PURPLE, 0, 1)); b.add(new VerticalGamePiece(2, Color.ORANGE, 2, 3)); b.add(new VerticalGamePiece(2, Color.LIGHTBLUE, 1, 4)); return b; } public static GameBoard board5() { GameBoard b = new GameBoard(13); b.add(new GoalPiece(3, 2)); b.add(new HorizontalGamePiece(2, Color.LIGHTGREEN, 1, 0)); b.add(new HorizontalGamePiece(3, Color.BLUE, 0, 3)); b.add(new HorizontalGamePiece(2, Color.BLACK, 4, 4)); b.add(new HorizontalGamePiece(2, Color.BROWN, 0, 5)); b.add(new HorizontalGamePiece(2, Color.YELLOW, 3, 5)); b.add(new VerticalGamePiece(3, Color.GOLD, 0, 0)); b.add(new VerticalGamePiece(2, Color.LIGHTBLUE, 1, 1)); b.add(new VerticalGamePiece(2, Color.PINK, 2, 1)); b.add(new VerticalGamePiece(2, Color.ORANGE, 4, 0)); b.add(new VerticalGamePiece(3, Color.VIOLET, 5, 1)); b.add(new VerticalGamePiece(2, Color.PURPLE, 3, 3)); b.add(new VerticalGamePiece(2, Color.GREEN, 2, 4)); return b; } public void add(GamePiece gp) { if (numGamePieces public int getNumGamePieces() { return numGamePieces; } public GamePiece[] getGamePieces() { return gamePieces; } public boolean isCompleted() { return completed; } // Return the piece at the given position public GamePiece pieceAt(int x, int y) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return null; } // Check if the board has been completed, and set the boolean accordingly public void checkCompletion(GamePiece gp) { // WRITE YOUR CODE HERE } }
import javafx.scene.paint.Color; public abstract class GamePiece { protected int width; protected int height; protected Color color; protected int topLeftX; protected int topLeftY; public GamePiece(int w, int h, Color c, int x, int y) { width = w; height = h; color = c; topLeftX = x; topLeftY = y; } public int getWidth() { return width; } public int getHeight() { return height; } public Color getColor() { return color; } public int getTopLeftX() { return topLeftX; } public int getTopLeftY() { return topLeftY; } public void moveRight() { topLeftX += 1;} public void moveLeft() { topLeftX -= 1;} public void moveDown() { topLeftY += 1;} public void moveUp() { topLeftY -= 1;} public boolean canMoveLeftIn(GameBoard b) { return false; } public boolean canMoveRightIn(GameBoard b) { return false; } public boolean canMoveDownIn(GameBoard b) { return false; } public boolean canMoveUpIn(GameBoard b) { return false; } }
import javafx.scene.paint.Color; public class GoalPiece extends HorizontalGamePiece { public GoalPiece(int x, int y) { super(2, Color.RED, x, y); } public boolean canMoveRightIn(GameBoard b) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return false; } }
import javafx.scene.paint.Color; public class HorizontalGamePiece extends GamePiece { public HorizontalGamePiece(int w, Color c, int x, int y) { super(w, 1, c, x, y); } public boolean canMoveLeftIn(GameBoard b) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return false; } public boolean canMoveRightIn(GameBoard b) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return false; } }
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; public class SliderPuzzleApp extends Application { private SliderPuzzleGame model; private SliderPuzzleView view; private GamePiece selectedPiece; private boolean justGrabbed; private int lastX; private int lastY; public void start(Stage primaryStage) { model = new SliderPuzzleGame(); view = new SliderPuzzleView(model); // Add event handlers to the inner game board buttons for (int w=1; wfor (int h=1; hnew EventHandler() { public void handle(MouseEvent mouseEvent) { handleGridSectionSelection(mouseEvent); } }); view.getGridSection(w, h).setOnMouseDragged(new EventHandler() { public void handle(MouseEvent mouseEvent) { handleGridSectionMove(mouseEvent); } }); } } // Plug in the Start button and NeaxtBoard button event handlers view.getStartButton().setOnAction(new EventHandler() { public void handle(ActionEvent actionEvent) { model.startBoard(); view.update(); } }); view.getNextBoardButton().setOnAction(new EventHandler() { public void handle(ActionEvent actionEvent) { model.moveToNextBoard(); view.update(); } }); primaryStage.setTitle("Slide Puzzle Application"); primaryStage.setResizable(false); primaryStage.setScene(new Scene(view, -10+SliderPuzzleView.GRID_UNIT_SIZE*(GameBoard.WIDTH+2),45+SliderPuzzleView.GRID_UNIT_SIZE*(GameBoard.HEIGHT+2))); primaryStage.show(); // Update the view upon startup view.update(); } private void handleGridSectionSelection(MouseEvent mouseEvent) { // FILL IN YOUR CODE } private void handleGridSectionMove(MouseEvent mouseEvent) { int currentGridX = (int)mouseEvent.getX(); int currentGridY = (int)mouseEvent.getY(); // FILL IN YOUR CODE } public static void main(String[] args) { launch(args); } }
public class SliderPuzzleGame { private static final int NUM_BOARDS = 5; private static final byte WAITING_TO_START = 0; private static final byte BOARD_IN_PROGRESS = 1; private static final byte BOARD_JUST_COMPLETED = 2; private GameBoard[] boards; private int boardNumber; private int numMovesMade; private byte gameMode; public SliderPuzzleGame() { resetBoards(); } public GameBoard getCurrentBoard() { return boards[boardNumber]; } public int getNumberOfMovesMade() { return numMovesMade; } public boolean isBoardInProgress() { return gameMode == BOARD_IN_PROGRESS; } public boolean areWeWaitingToStartABoard() { return gameMode == WAITING_TO_START; } // Set up the boards to the 5 example boards private void resetBoards() { boards = new GameBoard[NUM_BOARDS + 1]; boards[0] = new GameBoard(0); boards[1] = GameBoard.board1(); boards[2] = GameBoard.board2(); boards[3] = GameBoard.board3(); boards[4] = GameBoard.board4(); boards[5] = GameBoard.board5(); boardNumber = 1; gameMode = WAITING_TO_START; numMovesMade = 0; } // Record that a move was made public void makeAMove() { numMovesMade++; } // Allow the user to start playing the current board public void startBoard() { gameMode = BOARD_IN_PROGRESS; } // Simulate the completion of the current board public void completeBoard() { gameMode = BOARD_JUST_COMPLETED; } // Go to the next board in the game public void moveToNextBoard() { boardNumber++; gameMode = WAITING_TO_START; numMovesMade = 0; // Reset all the boards if we completed them all if (boardNumber > NUM_BOARDS) resetBoards(); } }
import javafx.event.EventHandler; import javafx.geometry.Pos; import javafx.scene.Node; import javafx.scene.control.Button; import javafx.scene.control.TextField; import javafx.scene.input.MouseEvent; import javafx.scene.layout.Pane; public class SliderPuzzleView extends Pane { public static final int GRID_UNIT_SIZE = 40; private Button[][] gridSections; private Button startButton, nextBoardButton; private TextField numMovesField; private SliderPuzzleGame model; public SliderPuzzleView(SliderPuzzleGame m) { model = m; gridSections = new Button[GameBoard.WIDTH+2][GameBoard.HEIGHT+2]; // Create the wall (i.e., non-pressable) buttons for (int i=0; inew Button(); gridSections[i][0].setDisable(true); gridSections[i][0].relocate(i * GRID_UNIT_SIZE, 0); gridSections[i][0].setPrefSize(GRID_UNIT_SIZE, GRID_UNIT_SIZE); gridSections[i][0].setStyle("-fx-base: BLACK"); gridSections[i][7] = new Button(); gridSections[i][7].setDisable(true); gridSections[i][7].relocate(i * GRID_UNIT_SIZE, 7 * GRID_UNIT_SIZE); gridSections[i][7].setPrefSize(GRID_UNIT_SIZE, GRID_UNIT_SIZE); gridSections[i][7].setStyle("-fx-base: BLACK"); } for (int i=1; inew Button(); gridSections[0][i].setDisable(true); gridSections[0][i].relocate(0, i * GRID_UNIT_SIZE); gridSections[0][i].setPrefSize(GRID_UNIT_SIZE, GRID_UNIT_SIZE); gridSections[0][i].setStyle("-fx-base: BLACK"); gridSections[7][i] = new Button(); gridSections[7][i].setDisable(true); gridSections[7][i].relocate(7 * GRID_UNIT_SIZE, i * GRID_UNIT_SIZE); gridSections[7][i].setPrefSize(GRID_UNIT_SIZE, GRID_UNIT_SIZE); if (i != 3) { gridSections[7][i].setStyle("-fx-base: BLACK"); } else { gridSections[7][i].setStyle("-fx-base: WHITE"); gridSections[7][i].setText("EXIT"); } } // Create the inner "pressable" Buttons for (int w=1; wfor (int h=1; hnew Button(); gridSections[w][h].relocate(w * GRID_UNIT_SIZE, h * GRID_UNIT_SIZE); gridSections[w][h].setPrefSize(GRID_UNIT_SIZE, GRID_UNIT_SIZE); gridSections[w][h].setStyle("-fx-base: WHITE; -fx-text-fill: RED;"); gridSections[w][h].setFocusTraversable(false); } } // Add all the buttons to the window for (int w=0; wfor (int h=0; h//if (!((h == 3) && (w == 7))) getChildren().add(gridSections[w][h]); } } // Add the Start and NextBoard buttons startButton = new Button("Start"); // ADD MORE CODE HERE nextBoardButton = new Button("Next Board"); // ADD MORE CODE HERE // Add the Num Moves Field numMovesField = new TextField(""); // ADD MORE CODE HERE getChildren().addAll(startButton, nextBoardButton, numMovesField); update(); // Update with no board } public Button getGridSection(int w, int h) { return gridSections[w][h]; } public Button getStartButton() { return startButton; } public Button getNextBoardButton() { return nextBoardButton; } public void update() { // FILL IN YOUR CODE HERE // Reset all colors to white // Update the colors of the buttons based on the GamePieces // Update the Start and NextBoard buttons // Disable all the board buttons unless we are in progress of playing a board // Update the number of moves field } }
import javafx.scene.paint.Color; public class VerticalGamePiece extends GamePiece { public VerticalGamePiece(int h, Color c, int x, int y) { super(1, h, c, x, y); } public boolean canMoveDownIn(GameBoard b) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return false; } public boolean canMoveUpIn(GameBoard b) { // REPLACE THE CODE BELOW WITH YOUR OWN CODE return false; } }
Need to be solved in Java, thank you.
D 95.105/115 Assignme x C Chegg Study Guided x Course: COMP 1105B Assignment C e:///C/Users/victor0323/Downloads/COMP1406 A6 W2017-Posted.pd COMP1406 Assignment #6 (Due: Monday, March 6th 10 am) In this assignment you will develop a simple game application that is based on the ModelWiew/Controller framework. Some of the code has been written for you in advance. You will add to the code and develop additional classes as well. Although the code is given here within the assignment, you may download it from cuLearn as well. We will be creating a slider-puzzle game where there are both horizontal sliding game pieces and vertical- sliding game pieces. The objective is to get the red horizontal-sliding goal piece out of the puzzle through the exit on the right side of the board. The user can grab and drag the game pieces to try and create a path for the red goal piece to reach the exit. Here, on the right, is an image of a game in progress. In this example, there are 2 horizontal sliding game pieces (light pink & purple), a horizontal-sliding goal piece (red), and 5 vertical sliding game pieces. The gray border represents non-movable "walls" in the game. Each time a game piece is grabbed and dragged, it counts as one "move". The idea is to get the goal piece to the exit in the least number of "moves possible. Once the goal piece is slid over to the exit, then a new board arrangement is brought up and the O fi a 20:55 1x ENG 201713/2 D 95.105/115 Assignme x C Chegg Study Guided x Course: COMP 1105B Assignment C e:///C/Users/victor0323/Downloads/COMP1406 A6 W2017-Posted.pd COMP1406 Assignment #6 (Due: Monday, March 6th 10 am) In this assignment you will develop a simple game application that is based on the ModelWiew/Controller framework. Some of the code has been written for you in advance. You will add to the code and develop additional classes as well. Although the code is given here within the assignment, you may download it from cuLearn as well. We will be creating a slider-puzzle game where there are both horizontal sliding game pieces and vertical- sliding game pieces. The objective is to get the red horizontal-sliding goal piece out of the puzzle through the exit on the right side of the board. The user can grab and drag the game pieces to try and create a path for the red goal piece to reach the exit. Here, on the right, is an image of a game in progress. In this example, there are 2 horizontal sliding game pieces (light pink & purple), a horizontal-sliding goal piece (red), and 5 vertical sliding game pieces. The gray border represents non-movable "walls" in the game. Each time a game piece is grabbed and dragged, it counts as one "move". The idea is to get the goal piece to the exit in the least number of "moves possible. Once the goal piece is slid over to the exit, then a new board arrangement is brought up and the O fi a 20:55 1x ENG 201713/2Step by Step Solution
There are 3 Steps involved in it
Step: 1
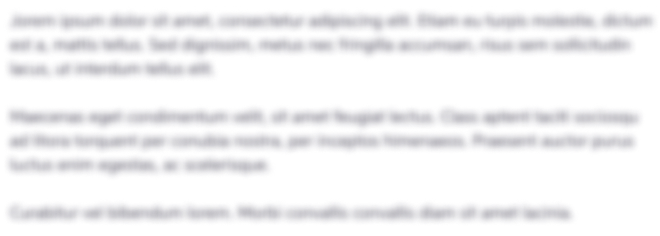
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started