Question
import java.text.*; class Coin { int year; double value; String denom; Coin(int year, double value, String denom) { this.year = year; this.value = value; this.denom
import java.text.*;
class Coin
{
int year;
double value;
String denom;
Coin(int year, double value, String denom)
{
this.year = year;
this.value = value;
this.denom = denom;
}
int getYear()
{
return year;
}
double getValue()
{
return value;
}
String getDenom()
{
return denom;
}
public String toString()
{
NumberFormat fmt = NumberFormat.getCurrencyInstance();
return denom + " " + year + " worth " + fmt.format(value);
}
}
import java.util.*;
class CoinCollection
{
ArrayList
String filename;
CoinCollection(String filename)
{
}
void add(int year, double value, String denom)
{
}
public String toString()
{
return "";
}
}
import java.util.*;
class CoinCollectionMain
{
public static void main(String[] args)
{
Scanner scan = new Scanner(System.in);
String filename = "myCoins.txt";
CoinCollection collection = new CoinCollection(filename);
int option;
do
{
System.out.println("Menu ----");
System.out.println("1-Print collection");
System.out.println("2-Add coin");
System.out.println("3-Load from text file");
System.out.println("4-Save to text file");
System.out.println("5-Load from binary file");
System.out.println("6-Save to binary file");
System.out.println("0-Exit");
System.out.print("Your option: ");
option = scan.nextInt();
scan.nextLine();
if (option==1)
;// add here one line of code to print the collection
else if (option==2)
{
System.out.print("Year: ");
int year = scan.nextInt();
System.out.print("Value: ");
double value = scan.nextDouble();
scan.nextLine();
System.out.print("Denomination: ");
String denom = scan.nextLine();
;// add here the code to add this coin to the collection
}
else if (option==3)
;// add here one line to call a method for loading from text file
else if (option==4)
;// add here one line to call a method for saving to text file
else if (option==5)
{
;// add here the lines of code to call a method for loading
// from binary file, and handle the exception if a problem arises
}
else if (option==6)
;// add here one line to call a method for saving to binary file
} while (option != 0);
}
}
Context: You are a coin collector, and you would like to keep track of the coins you have in your collection. For each coin, you have its year (as int), its value (as double), and its denomination (as string). For example, you may have a 1 cent 1947 worth $1.50, or a 5 cents 1924 worth $10. A class "Coin" is provided to you, as well as the skeleton for a class "Coin Collection" (containing an ArrayList of such Coin objects). A driver class ("Coin Collection Main") is provided, to show you the different options available to the user. You need to complete the implementation of those classes. Tasks: Add the code inside the following methods in the Coin Collection class: o constructor (creating an empty ArrayList and saving the filename sent by parameter) o add (creating the Coin object with the information provided, and adding such object to the ArrayList) o toString (preparing a String to print the whole collection) Add the lines of code to implement the options 1 and 2 in the main method. Create a method to load the collection from a text file (myCoins.txt). The text file should be structured such that you have one coin per line. You have to figure out where such method should go. This method should remove all items from the ArrayList, and put instead the coins previously saved in the text file. If there is a problem opening the file, the current ArrayList should not be changed i.e., its items should not be removed from it). If you have a problem parsing one of the lines in the file, you should stop loading the file from that point on, keep all the other coins that you successfully loaded into the ArrayList, and indicate to the user how many elements you managed to load. This method should not throw any exception. Finally, call this method under option 3 in the main method. Create a method to save the collection into a text file (myCoins.txt), which can be read with your method above. Make sure that exceptions are handled inside that method (i.e., not thrown). You should not save again the coins which were already in the text file: if the user loads the text file first, containing 5 coins, and then add 2 more coins, this method should only append the 2 extra coins to the text file. After this, if one more coin is added and then saved, then only that extra coin should be added to the text file. Hint: find a way to remember how many coins are in the text file, and loop through the remaining coins in the ArrayList. Finally, call this method under option 4 in the main method. Create a method to save the collection into a binary file instead, as object serialization. Hardcode the file name: MyCoinCollection.dat. You have to figure out where such method should go. You can save the whole collection this time, not just the extra items that were added. You should also save the filename, as well as any information you are keeping related to the number of coins in the text file. Note that you will have to make modifications to some existing classes in order to do this. Also, as a hint, the ArrayList does implement the Serializable interface. Your method here should not throw any exception. Finally, call this method under option 6 in the main method. Create a corresponding method to load the collection from a binary file (object serialization again). Make your method return the collection object that was loaded. If there is a problem opening the file, or reading the objects (i.e., lassNotFoundException), do not return a collection. Instead, throw your own exception named "CoinCollection NotloadedException" (containing a different message depending on the exact nature of the problem). If there is a problem closing the file, simply print an error message and do return the collection. Finally, add the proper code in the main method under option 5. In particular, you should catch the new exception if thrown. In the case that an exception is thrown, print the error message (as coded into the exception itself), and ask the user whether s/he wants to keep the old collection currently in memory or create a new (empty) collection. Implement both options properly. In general, ensure that all exceptions handled do indicate the problem faced to the user. import java.text.*; class Coin o ovou A WNP int year; double value; String denom; Coin(int year, double value, String denom) 11 this.year = year; this.value = value; this.denom = denom; 13 14 15 16 int getYear() 17 { 18 return year; 19 20 double getValue() return value; 25 26 String getDenom() 27 9 return denom; 29 30 31 32 public String toString() { NumberFormat fmt = NumberFormat.getCurrency Instance( return denom + " " + year + " worth " + fmt.format(value); 34 35 36 } 37 import java.util.*; class CoinCollection 00vu W NP ArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
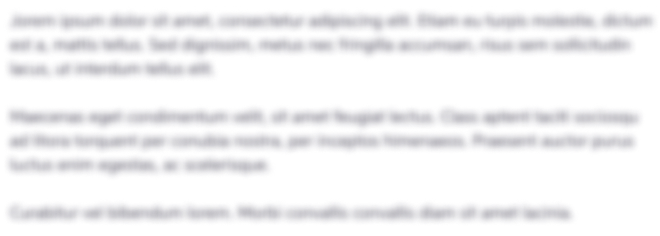
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started