Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.ArrayList; import java.util.Iterator; abstract class GenericList implements CreateIterator { private Node head; //A linked list, could change to another data structure private int length;
import java.util.ArrayList; import java.util.Iterator; abstract class GenericList implements CreateIterator{ private Node head; //A linked list, could change to another data structure private int length; public abstract void add(I data); public void setLength(boolean how) { if (how) { ++length; } else { --length; } } public int getLength() { return length; } public Node getHead(){ return head; } public void setHead(Node head) { this.head = head; } public I delete() { if (head == null) { return null; } I obj = head.data; head = head.next; return obj; } public void print() { if (head == null) { System.out.println("Empty List!"); } else { Node temp = head; while (temp != null) { System.out.println(temp.data); temp = temp.next; } } } public ArrayList dumpList(){ ArrayList list = new ArrayList(); if(head == null) { System.out.println("EmptyList: returning empty list"); return list; } else { while(head != null) { list.add(head.data); head = head.next; } return list; } } public Iterator createIterator(){ return new GLIterator(head); } class Node{ T data; Node next; } }
GenericQueue:
import java.util.Iterator; public class GenericQueue extends GenericList { GenericQueue(I data){ add(data); this.setLength(true); } public void enqueue(I data) { add(data); this.setLength(true); } public I dequeue() { if(this.getHead() != null) { this.setLength(false); } return delete(); } @Override public void add(I data) { // TODO Auto-generated method stub Node iter = new Node(); if(this.getHead() == null) { Node temp = new Node(); temp.data = data; temp.next = this.getHead(); this.setHead(temp); // head = temp; return; } else { iter = getHead(); while(iter.next != null) { iter = iter.next; } } Node newNode = new Node(); newNode.data = data; newNode.next = null; iter.next = newNode; } }
GenericStack:
public class GenericStack extends GenericList { GenericStack(I data){ add(data); this.setLength(true); } public void push(I data) { add(data); this.setLength(true); } public I pop() { if(getHead() != null) { this.setLength(false); } return delete(); } @Override public void add(I data) { // TODO Auto-generated method stub Node temp = new Node(); temp.data = data; temp.next = getHead(); setHead(temp); } }
CreateIterator:
import java.util.Iterator; public interface CreateIterator { public Iterator createIterator(); }
GLIterator:
import java.util.Iterator; public class GLIterator implements Iterator{ private GenericList.Node theList; GLIterator(GenericList.Node list){ this.theList = list; } @Override public boolean hasNext() { // TODO Auto-generated method stub if(theList == null) { return false; } else { return true; } } @Override public I next() { // TODO Auto-generated method stub I val = theList.data; theList = theList.next; return val; } }
GLProject:
import java.util.ArrayList; import java.util.Iterator; public class GLProject { public static void main(String[] args) { // TODO Auto-generated method stub System.out.println("hello generic lists"); GenericStacki = new GenericStack (20); i.push(30); i.push(40); i.push(50); i.print(); Iterator it = i.createIterator(); while(it.hasNext()) { System.out.println(it.next()); } ArrayList theList = i.dumpList(); for(Integer ival : theList) { System.out.println("from AL: " + ival); } i.print(); ArrayList theList2 = i.dumpList(); for(Integer ival : theList2) { System.out.println("from AL2: " + ival); } } }
List Test:
import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; import org.junit.jupiter.params.ParameterizedTest; import org.junit.jupiter.params.provider.ValueSource; class ListTest { GenericStacks; @BeforeEach void init() { s = new GenericStack (300); } @Test void testInitGS() { assertEquals("GenericStack", s.getClass().getName(),"init failed on GS"); } @Test void testInitNode() { assertEquals("GenericList$Node", s.getHead().getClass().getName(), "node not init"); } @Test void testNodeVal() { assertEquals(300, s.pop(), "value wrong in Node: not 200"); } @Test void testEmptyList() { s.pop(); assertNull(s.getHead()); } @ParameterizedTest @ValueSource(chars = {'a','b','a','b'}) void isLetterA(char input) { assertEquals('a', input, "not letter 'a'"); } }
Hopefully this is better
I HAVE NEVER USED JUNIT TESTING PLS HELP I DON'T KNOW WHAT IM DOING
Description: In this homework you will get some practice creating a JUnit 5 test suite for the classes in project #1. You will also add functionality for the enhanced for each loop seen in class. Implementation Details: Your task is to fully test the Generic Stack and Generic Queue classes as well as the iterator. Keep in mind, there could be errors in the code provided. You will need to create two additional Junit5 test classes in their own files in the same directory as List Test.java. Call them Queue Test.java and Iteratorlterable Test.java. You will continue to test the Generic Stack in List Test.java. You are required to use a minimum of 3 annotations and 4 assertions in your unit tests. You must test the classes and iterator in a logical, step by step, fashion. Additional to the test cases provided, A minimum of 10 test cases per class and 10 for the iterator. You will probably need more to fully test this program. Each class and the iterator should be tested in their own file. Enhanced For-each loop: Your list library is almost complete except it does not allow the user to do: list.forEach(e -> System.out.println(e)); Your going to add the functionality for an enhanced for-each loop now. You will implement the Iterable interface in the Generic List class. Remember, you can implement as many interfaces as you want in a class. Just add them as a comma separated list after the implements key word. You will need to implement one method from the Iterable interface (check documentation to see what it is); the definition will look a lot like the method you already implemented for the Createlterator interface. You will write test cases for an enhanced for each loop in the Iteratorlterable Test.java file. Yes, theses can count towards your minimum of 10 Generic List: Description: In this homework you will get some practice creating a JUnit 5 test suite for the classes in project #1. You will also add functionality for the enhanced for each loop seen in class. Implementation Details: Your task is to fully test the Generic Stack and Generic Queue classes as well as the iterator. Keep in mind, there could be errors in the code provided. You will need to create two additional Junit5 test classes in their own files in the same directory as List Test.java. Call them Queue Test.java and Iteratorlterable Test.java. You will continue to test the Generic Stack in List Test.java. You are required to use a minimum of 3 annotations and 4 assertions in your unit tests. You must test the classes and iterator in a logical, step by step, fashion. Additional to the test cases provided, A minimum of 10 test cases per class and 10 for the iterator. You will probably need more to fully test this program. Each class and the iterator should be tested in their own file. Enhanced For-each loop: Your list library is almost complete except it does not allow the user to do: list.forEach(e -> System.out.println(e)); Your going to add the functionality for an enhanced for-each loop now. You will implement the Iterable interface in the Generic List class. Remember, you can implement as many interfaces as you want in a class. Just add them as a comma separated list after the implements key word. You will need to implement one method from the Iterable interface (check documentation to see what it is); the definition will look a lot like the method you already implemented for the Createlterator interface. You will write test cases for an enhanced for each loop in the Iteratorlterable Test.java file. Yes, theses can count towards your minimum of 10 Generic ListStep by Step Solution
There are 3 Steps involved in it
Step: 1
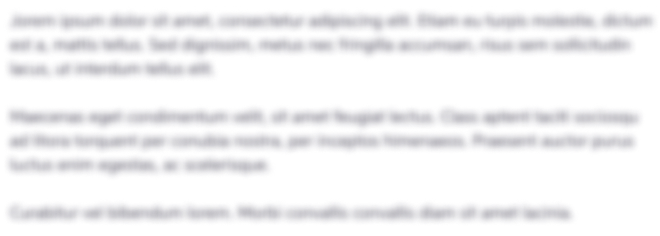
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started