Question
import java.util.Arrays; import java.util.stream.Collectors; public class PQ3 { private E[] queue; private int added = 0; private int removed = 0; /** * initializes objects
import java.util.Arrays;
import java.util.stream.Collectors;
public class PQ3
private E[] queue;
private int added = 0;
private int removed = 0;
/**
* initializes objects of type PQ3. This should instantiate the queue field.
* @param capacity the maximum size of the queue
*/
public PQ3(int capacity){
queue = newArray(capacity);
}
/**
* @return the queue
*/
public E[] getQueue(){
return queue;
}
/**
* Sets the queue so that we can decouple testing for the add and remove operations
* @param items items for the array
*/
public void setQueue(E[] items){
queue = items;
added=items.length;
removed = 0;
}
/**
* A method to help with testing
* @param items a list of the items to print
* @return returns a String that is similar to Arrays.toString but fills in "null" if there is a null.
*/
public static String formatOutput(Object[] items){
if (items == null) return "null";
return String.format("[%s] ",
String.join(", ",
Arrays.stream(items).map(o -> ((o != null) ? o.toString() : "null")).collect(Collectors.toList())));
}
/**
* @param capacity the size of the array
* @return a generic array of size capacity
*/
public E[] newArray(int capacity){
@SuppressWarnings("unchecked")
E[] arr = (E[]) new Object[capacity];
return arr;
}
/**
* If the number of items to remove is larger than the current size of the queue,
* return null. Otherwise remove and return n (inclusive) items from the queue.
* @param n the number of elements to remove
* @return the list of items removed in the order they were removed, from front
* to the back.
*/
public E[] removeNItems(int n){
@SuppressWarnings("unchecked")
E[] arr = (E[]) new Object[n];
if(n > size())
{
return null;
}
else
{
for(int i =0; i
{
arr[i] = queue[i];
queue[i] = null;
removed++;
}
}
return arr;
}
/**
* If the number of items to be added + the current elements would exceed the capacity of the queue, return false.
* Otherwise add the items to the queue from the first element to the last element and return true.
* @param items the items to be added to the queue
* @return true if added successfully, false otherwise.
*/
public boolean addNItems(E[] items){
if(items.length + size() > queue.length)
{
return false;
}
else
{
for(int i = 0; i { queue[i] = items[i]; added++; } return true; } } /** * @return the size of the queue */ public int size(){ return added - removed; } public static void main(String [] args){ // testing the constructor PQ3 System.out.print("Testing the constructor with a capacity of 10: " + formatOutput(pq3.getQueue())); System.out.println("Size of queue after operation: " + pq3.size()); // testing the newArray method System.out.println("Testing the newArray method with a capacity of 5: " + formatOutput(pq3.newArray(5))); System.out.println("Trying to add 5 items"); Integer[] addFive = {1, 2, 3, 4, 5}; System.out.println("Able to add 5 items-> " + pq3.addNItems(addFive)); System.out.println("Size of queue after operation: " + pq3.size()); System.out.println("Array after adding 5 ints: " + formatOutput(pq3.getQueue())); System.out.println("Trying to remove 4 items"); Object[] fourItemsRemoved = pq3.removeNItems(4); System.out.print("Items removed: " + formatOutput(fourItemsRemoved)); System.out.println("Size of queue after operation: " + pq3.size()); System.out.println("Array after trying to remove four items: " + formatOutput(pq3.getQueue())); System.out.println("Trying to add 8 items"); Integer[] addEight = {1, 2, 3, 4, 5, 6, 7, 8}; System.out.println("Able to add 5 items-> " + pq3.addNItems(addEight)); System.out.println("Size of queue after operation: " + pq3.size()); System.out.println("Array after adding 8 ints: " + formatOutput(pq3.getQueue())); } } Using a circular array queue! make sure the outputs matches! thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
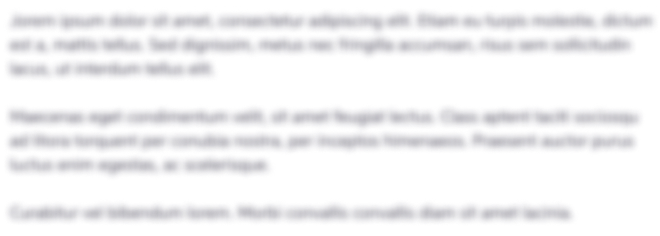
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started