Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.Date; public class Account { private int id; private double balance; private double annualInterestRate; private Date DateCreated; / / No - arg constructor public
import java.util.Date;
public class Account
private int id;
private double balance;
private double annualInterestRate;
private Date DateCreated;
Noarg constructor
public Account
id ;
balance ;
annualInterestRate ;
DateCreated new Date;
Constructor with specified id and initial balance
public Accountint id double initialBalance
this.id id;
balance initialBalance;
annualInterestRate ;
DateCreated new Date;
Accessor and mutator methods for id
public int getId
return id;
public void setIdint id
this.id id;
Accessor and mutator methods for balance
public double getBalance
return balance;
public void setBalancedouble balance
this.balance balance;
Accessor and mutator methods for annualInterestRate
public double getAnnualInterestRate
return annualInterestRate;
public void setAnnualInterestRatedouble annualInterestRate
this.annualInterestRate annualInterestRate;
Accessor method for dateCreated
public Date getDateCreated
return DateCreated;
Method to calculate monthly interest rate
public double getMonthlyInterestRate
return annualInterestRate ;
Method to withdraw a specified amount
public void withdrawdouble amount
if amount && amount balance
balance amount;
else
System.out.printlnInvalid withdrawal amount.";
Method to deposit a specified amount
public void depositdouble amount
if amount
balance amount;
else
System.out.printlnInvalid deposit amount.";
public static void mainString args
Creating an Account object with specified values
Account harry new Account;
harry.setId;
harry.setBalance;
harry.setAnnualInterestRate;
Withdraw $
harry.withdraw;
Deposit $
harry.deposit;
Displaying account information
System.out.printlnId harry.getId;
System.out.printlnBalance $ harry.getBalance;
System.out.printlnMonthly Interest $harrygetBalance harry.getMonthlyInterestRate;
System.out.printlnDate Created harry.getDateCreated;
flowchart of this
Step by Step Solution
There are 3 Steps involved in it
Step: 1
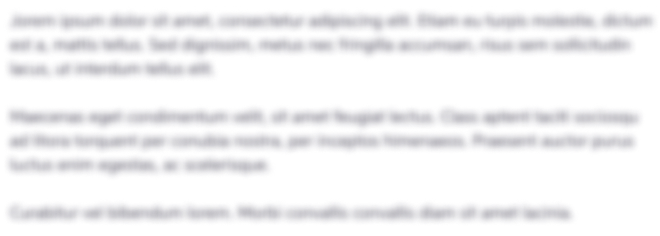
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started