Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.Random; import java.util.Scanner; import java.util.Stack; class BinarySearchTree { public static class Node { int data; Node left; Node right; public Node ( int data
import java.util.Random;
import java.util.Scanner;
import java.util.Stack;
class BinarySearchTree
public static class Node
int data;
Node left;
Node right;
public Nodeint data
this.data data;
this.left null;
this.right null;
public Node root;
public BinarySearchTree
root null;
public void insertint data
Node newNode new Nodedata;
if root null
root newNode;
return;
Node current root;
Node parent null;
while true
parent current;
if data current.data
current current.left;
if current null
parent.left newNode;
return;
else if data current.data
current current.right;
if current null
parent.right newNode;
return;
else
Duplicate values are not allowed you can modify this part as needed
return;
public void iterativeInOrderTraversalNode root
if root null
System.out.printlnTree is empty";
return;
Stack stack new Stack;
Node current root;
while current null stack.isEmpty
while current null
stack.pushcurrent;
current current.left;
current stack.pop;
System.out.printcurrentdata ;
current current.right;
public static void mainString args
BinarySearchTree bst new BinarySearchTree;
Scanner scanner new ScannerSystemin;
Prompt the user to enter the size of the BST
System.out.printEnter the size of the BST: ;
int bstSize scanner.nextInt;
Prompt the user to enter the minimum and maximum values for random numbers
System.out.printEnter the minimum value for random numbers: ;
int minValue scanner.nextInt;
System.out.printEnter the maximum value for random numbers: ;
int maxValue scanner.nextInt;
Generate random numbers and insert them into the BST
Random random new Random;
for int i ; i bstSize; i
int randomNum random.nextIntmaxValue minValue minValue;
bstinsertrandomNum;
Display the BST inorder traversal using iterative approach
System.out.printlnBinary search tree inorder traversal:";
bstiterativeInOrderTraversalbstroot;
Close the scanner
scanner.close;
This code doesn't work for bigger sizes such as Could you please fix that?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
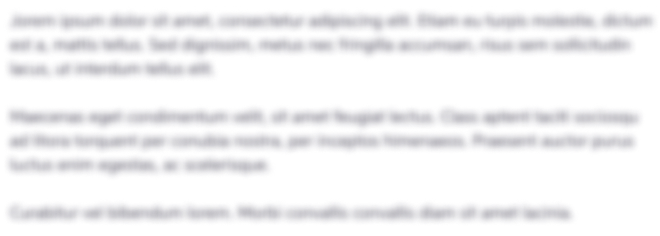
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started