Question
import java.util.Scanner; public class Calculaator { public static void main(String[] args) { int choice, sum = 0, i = 0, difference = 0; double num1,
import java.util.Scanner;
public class Calculaator { public static void main(String[] args) { int choice, sum = 0, i = 0, difference = 0; double num1, num2; Scanner input = new Scanner(System.in);
System.out.println("Welcome to Fancy Calculator"); System.out.println("Please choose your operation: "); System.out.println("1 for Addition" + ' ' + "2 for Subtraction"); System.out.println("3 for Multiplication" + ' ' + "4 for Division" + ' ' + "5 for calculating average"); choice = input.nextInt();
//Addition and Subtraction program if (choice == 1) { System.out.println("You have selected Addition"); System.out.println("Enter numbers to add (select enter after each one) to add all numbers, enter 0: "); int number; do { number = input.nextInt(); sum += number; } while (number != 0); System.out.println("Sum of all numbers is = " + sum);
} else if (choice == 2) { System.out.println("You have selected Subtraction. You will subtract 2 numbers"); System.out.println("Enter first number: "); int subnum1 = input.nextInt(); System.out.println("Enter second number: "); int subnum2 = input.nextInt();
System.out.println("Difference of " + subnum1 + " and " + subnum2 + " is = " + (subnum1 - subnum2));
} else if (choice == 3) { System.out.println("You have selected Multiplication. "); System.out.println("Enter number 1: "); double multnum1 = input.nextDouble(); System.out.println("Enter number 2: "); double multnum2 = input.nextDouble(); System.out.println(multnum1 + " times " + multnum2 + " = " + CalcMultiplication.multiplication(multnum1, multnum2));
} else if (choice == 4) { System.out.println("You have selected Division. "); System.out.println("Enter number 1: "); double divnum1 = input.nextDouble(); System.out.println("Enter number 2: "); double divnum2 = input.nextDouble(); System.out.println(divnum1 + " divided by " + divnum2 + " = " + CalcDivision.division(divnum1, divnum2)); } else if (choice == 5) { Scanner sc = new Scanner(System.in); System.out.println("You have selected calculating an average"); System.out.println("Enter number of values you want to average: "); int n = sc.nextInt(); int sum1 = 0;
int[] numbers = new int[n];
for(int k=0; k < numbers.length ; k++) { System.out.println("Enter grade " + (k + 1) + " :"); int a = sc.nextInt(); sum1 = sum1 + a; }
double average = sum1 / (double) n;
System.out.println("Average value of array elements is : " + average); sc.close();
} }
}
public class CalcMultiplication { public static double multiplication(double x, double y) { double result; result = x * y; return result; } }
public class CalcDivision { public static double division(double x, double y) { double result; if (y == 0) { System.out.println("The result is undefinded"); } else { System.out.println(x + "divided by" + y + "is" + (x/y));
} result = x / y; return result; }
}
I made this simple calculator program using two different classes. I need help making this code more object oriented by adding features such as abstraction inheritance, constructors, and encapsulation.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
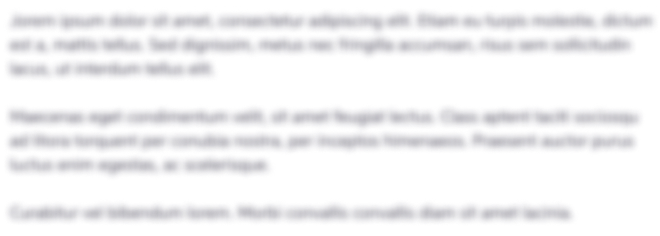
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started