Question
import java.util.Scanner; public class GamePlay { private Players[] currentPlayers = new Players[3]; public static void main(String[] args) { Scanner input = new Scanner(System.in); GamePlay game
import java.util.Scanner;
public class GamePlay {
private Players[] currentPlayers = new Players[3];
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
GamePlay game = new GamePlay();
Hosts host = new Hosts("Iron Man","");
host.randomizeNum();
Turn turn = new Turn();
for (int i = 0; i < game.currentPlayers.length; i++) {
System.out.print("Enter your name: ");
String name = input.nextLine();
System.out.print("Would you like to enter a last name? (yes/no): ");
String answer = input.nextLine();
if (answer.equals("yes")) {
System.out.print("Enter your last name: ");
String lastName = input.nextLine();
game.currentPlayers[i] = new Players(name, lastName);
} else {
game.currentPlayers[i] = new Players(name);
}
game.currentPlayers[i].setMoney(1000);
}
boolean playAgain = true;
while (playAgain) {
for (int i = 0; i < game.currentPlayers.length; i++) {
while (!turn.takeTurn(game.currentPlayers[i % game.currentPlayers.length], host)) {
}
}
System.out.println("Would you like to play again? (yes/no): ");
String answer = input.nextLine();
if (answer.equals("no")) {
playAgain = false;
} else {
host.randomizeNum();
}
}
}
}
interface Award {
int displayWinnings(Players player, boolean correctGuess);
}
class Money implements Award {
private int winningAmount = 100;
private int losingAmount = 50;
public int displayWinnings(Players player, boolean correctGuess) {
if (correctGuess) {
System.out.println(player.getName() + " won " + winningAmount + "$");
return winningAmount;
} else {
System.out.println(player.getName() + " lost " + losingAmount + "$");
return -losingAmount;
}
}
}
class Physical implements Award {
private String[] physicalPrizes = {"Television", "Laptop", "Smartphone", "Tablet", "Game Console"};
public int getRandomPrize() {
Random random = new Random();
return random.nextInt(physicalPrizes.length);
}
public int displayWinnings(Players player, boolean correctGuess) {
if (correctGuess) {
int prizeIndex = getRandomPrize();
System.out.println(player.getName() + " won " + physicalPrizes[prizeIndex]);
return 0;
} else {
int prizeIndex = getRandomPrize();
System.out.println(player.getName() + " could have won " + physicalPrizes[prizeIndex]);
return 0;
}
}
}
class Turn {
private Money money = new Money();
private Physical physical = new Physical();
public boolean takeTurn(Players player, Hosts host) {
System.out.print(player.getName() + ", please enter your guess: ");
Scanner input = new Scanner(System.in);
int guess = input.nextInt();
boolean correctGuess = host.checkGuess(guess);
int winnings = 0;
System.out.println("Enter 1 for Money or 2 for Physical Prize: ");
int choice = input.nextInt();
if (choice == 1) {
winnings = money.displayWinnings(player, correctGuess);
} else if (choice == 2) {
winnings = physical.displayWinnings(player, correctGuess);
}
player.addMoney(winnings);
System.out.println(player.getName() + " now has " + player.getMoney() + "$");
return correctGuess;
}
}
class Hosts {
private String name;
private int numToGuess;
public Hosts(String name, int numToGuess) {
this.name = name;
this.numToGuess = numToGuess;
}
public void randomizeNum() {
Random random = new Random();
numToGuess = random.nextInt(10) + 1;
}
public boolean checkGuess(int guess) {
if (guess == numToGuess) {
System.out.println("You won!");
return true;
} else {
System.out.println("You lost.");
return false;
}
}
}
class Players {
private String name;
private int money;
public Players(String name) {
this.name = name;
}
public Players(String name, String lastName) {
this.name = name + " " + lastName;
}
public void setMoney(int money) {
this.money = money;
}
public void addMoney(int money) {
this.money += money;
}
public int getMoney() {
return money;
}
public String getName() {
return name;
}
}
Delete the Numbers.java class. We do not need this since we are changing to a word game. Just remember that it is being used by Hosts.java and Turn.java. So, you'll need to provide different functionality (and that's in the next steps)
Create an exception class called MultipleLettersException that extends Exception
Override the getMessage() method to display the message "More than one letter was entered"
Create a class called Phrases.java that functions similarly to Numbers.java but with phrases, except it can throw a MultipleLettersException.
From the Hosts.java class, instead of generating a random number with Numbers, send the phrase chosen by the host to the Phrases.java class to store in a static field, called gamePhrase.
Create a String field in Phrases.java called playingPhrase. Use some of the String methods to make the playingPhrase field have an underline instead of each letter in gamePhrase. (Example: If gamePhrase is "Have a nice day", then playing Phrase should be "____ _ ____ ___")
Create a findLetters() method that accepts a String of one letter as a parameter. If the string is longer than one letter, throw a MultipleLettersException. Use String methods to find all indices of that letter within gamePhrase and change the corresponding underline in playingPhrase to the correct letter. If there are no underlines left in playingPhrase, tell the player they won and award a prize.
Make changes to the Hosts.java class
When the Host instance is created, have the "host" enter a phrase for the players to guess(THINK: Wheel of Fortune) and store this phrase in the gamePhrase field of Phrases.java.
Add functionality, so that if the players decide to "play again", the host enters a new phrase.
Make changes to Turn.java and/or GamePlay.java
Instead of using Numbers.java, now use Phrases.java. Ask the player for one letter instead of an integer. Use a try catch block with the findLetters() method from Phrases.java to evaluate the guess the player entered. Be sure to catch and handle a MultipleLettersException. (The program should NOT crash.) Also catch and handle the possibility of the user entering numbers or symbols instead of letters.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
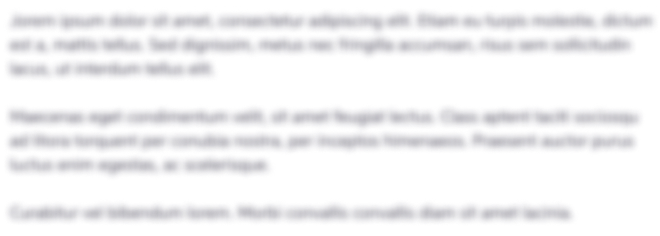
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started