Question
import java.util.Scanner; public class Lab1 { public static void main(String[] args) { Scanner scr = new Scanner(System.in); System.out.println(Please enter a string.); String str = scr.nextLine();
import java.util.Scanner;
public class Lab1 {
public static void main(String[] args) {
Scanner scr = new Scanner(System.in); System.out.println("Please enter a string."); String str = scr.nextLine(); scr.close(); boolean correct = true; //TODO - Check the number of spaces //TODO - Check the characters after the space //TODO - Check the characters before the space //TODO - Check the vowel count //TODO - Check the first character if (correct) System.out.println("This string is in the correct format."); else System.out.println("This string is NOT in the correct format!"); } //Note: you may not want to pass the whole string to some of these methods. public static int countSpaces(String str) { //TODO - Optional helper method } public static boolean containsNonNumeric(String str) { //TODO - Optional helper method } public static boolean containsNonAlphabetical(String str) { //TODO - Optional helper method } public static int countVowels(String str) { //TODO - Optional helper method }
public static boolean smallestCharFirst(String str) { //TODO - Optional helper method }
}
---------------------------------------------
please write the program above by using java language and dont forget to post a pic of the output.
Introduction This week's lab will focus on string manipulation. It is based on the strings generated in this week's homework assignment. You should review the requirements of the homework before attempting this lab. What you need to do You are to write a program that takes user input in the form of string. Then, check the string to see if it matches the output format of the homework assignment. In other words, answer the question "could this string have been created by the homework program?" Print out a message reporting whether the string matches or does not. You may use our sample program as a starting point. Review the string generation procedure from the homework assignment. You can see that the result will be a string consisting of a series of alphabetical characters, followed by a single space, followed by a series of numeric characters. The numeric characters should represent the count of vowels in the alphabetical part. Also, because of the swap we made, the first character in the alphabetical part should be the smallest. Your program must report that the format is incorrect when: 1. There is not exactly one space character, 2. any character after the space is not numeric, 3. any character before the space is not alphabetical, 4. the integer value of the numeric part does not equal the count of vowels in the alphabetic part, or 5. there is a smaller alphabetical character after the first alphabetical character. If none of these cases are met, then report that the string is in the correct format. Some helpful methods to use are: Integer.parseInt(String) //converts a string number to an integer number indexOf(int) //returns first location of specified character in string lastIndexOf(int) //returns last location of specified for character in string charAt(int) //returns the char value at the specified index substring(int, int) //splits and returns a string based on parameters passed to it Integer.parseInt() can throw an exception if a string that does not represent a number is passed to it. For example, Integer.parseInt("Forty") will throw an exception but Integer.parseInt("40") will return the integer value 40. You do not need to worry about this. Later in the semester we will learn how to handle exceptions. This also means that Integer.parseInt() can throw an exception if surrounding white space is not removed from the passed in the string. For example, Integer.parseInt(" 2") will thrown an exception. Make sure you aren't including extraneous characters in the string when you call this method. Here are some examples you can test your code on. You don't need to print out the reason for failing. It's included for your reference. Please enter a string. aabecihfe 5 This string is in the correct format. Please enter a string. cgf 0 This string is NOT in the correct format! (It contains too many spaces) Please enter a string. CORO This string is NOT in the correct format! (It contains an uppercase character) Please enter a string. cg! 0 This string is NOT in the correct format! (It contains a non-alphanumeric character) Please enter a string. cgl o This string is NOT in the correct format! (characters not in the correct place) Please enter a string. acge 1 This string is NOT in the correct format! (wrong vowel count) Please enter a string. zbae 2 This string is NOT in the correct format! (first character not the smallest) Please enter a string. This string is in the correct formatStep by Step Solution
There are 3 Steps involved in it
Step: 1
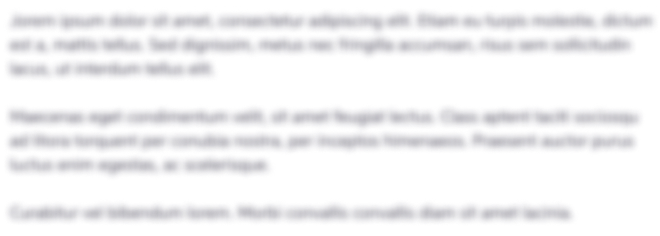
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started