Question
IMPORT LIBRARIES: from IPython.display import Image, display #display image REGEX & DATA STRUCTURE & ALGORITHM & HELPER FUNCTION: We will apply these following techniques in
IMPORT LIBRARIES:
from IPython.display import Image, display #display image
REGEX & DATA STRUCTURE & ALGORITHM & HELPER FUNCTION:
We will apply these following techniques in this assignment:
- LOOP
- List comprehension
- REGEX
- DATA STRUCTURE
- Helper function (a lot)
- Pandas
- Numpy
- Algorithm
- Hash
- If else
- Twilio (I guessed you traded a lot of Twilio stock but don't know exactly what they do)
- Code architecture
I don't expect you to care about time and space complexity for now, but it would be great for you to do so if you have some time. Our priority is to get it runs first, before caring about efficiency.
These below references are needed beside what I already taught you to do assignment.
ref:
- www.twilio.com/referral/9h3pJB #register twilio account (use my referral)
- https://www.fullstackpython.com/blog/send-sms-text-messages-python.html#:~:text=create #how to send text from python
- https://www.programiz.com/python-programming/datetime/current-datetime #how to get current date
- https://en.wikipedia.org/wiki/Luhn_algorithm #how to validate credit card number
- https://medium.com/@dwernychukjosh/sha256-encryption-with-python-bf216db497f9 #idea on how to hash sensitive infos
Imagine you are working for Best buy, your manager ask you to do an app to allow customers to register their account for the STUDENT discount. The app will take in their name, username, email, phone number, birthday, credit card number and of course a password. The app should be able to perform these following tasks:
Standard Function:
- Check if customer enter all the above infos in the correct formatting (criteria will be specified below)
- Store all those infos in a dataframe (DATABASE)
- Log-in function that can check if customer enter their username and password correctly (case sensitive)
- Temporarily suspend their account if they enter the wrong password for 3 times or more
- Allow customer to reset their passwords if they don't remember their password
Advanced Function:
- Check if it is their birthday, if yes, then text them and say "Happy Birthday to Name"
- Make an Hash function to hash all the sensitive infos (Cyber Security)
- A function alows people to do multi-factor authentication by phone (text them a code), and birth year
CRITERIA:
Username:
- Must not be the same as any other usernames that are already registered
- Must have at least 6 characters
Email:
- Email should have @
- Email should have domain
- The top-level domain should be "edu" => this is to verify if the customer is an active student to qualify for student discount.
- Must not duplicate with any existing email in the database
Phone number :
- Customer must be in the U.S meaning their phone number must in U.S format and should start with +1
- Must be in format +1-xxx-xxx-xxxx
- Must not duplicate with any existing phone number in the database
Birthday:
- Must be in format mm-dd-yyyy
- Must not be in the future compare to the current date
Credit Card:
- Must be a string of numbers depend on its length, for example if it is a MasterCard it should have 16 digits then the format is xxxxxxxxxxxxxxxx (16 x)
- Must check if it is a valid credit card number from 4 famous financial institutions (Using LUHN algorithm)
The first digit in your credit-card number signifies the system:
- 37 - American Express (15 digits)
- 4 - Visa (13 or 16 digits)
- 5 - MasterCard (16 digits)
- 6 - Discover Card (16 digits)
Password:
- Must have at least 1 number.
- Must have at least 1 uppercase and one 1 character.
- Must have at least 1 special symbol expect the question mark "?".
- Must be between 6 to 20 characters long.
- Can have space in between.
DATAFRAME (DATABASE):
Must have 8 columns named:
- Name
- Username
- Birthday
- Phone number
- Credit card number (HASHED)
- Credit institution name (ex: VISA, MASTER,...)
- Password (HASHED)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
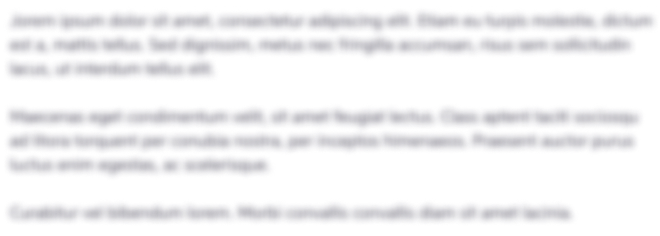
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started