Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import java.io.*; import java.util.ArrayList; import java.util.Scanner; public class SearchEngine { private int mode; private Tree nodeTree; // List -> Tree //
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import java.io.*; import java.util.ArrayList; import java.util.Scanner; public class SearchEngine { private int mode; private TreenodeTree; // List -> Tree // build everything bahaha public SearchEngine(int mode) throws IOException { if(mode == 3){ this.mode = 3; this.nodeTree = new BST<>(); buildList(); }else if (mode == 4){ this.mode = 4; this.nodeTree = new AVL<>(); buildList(); } else { System.out.println("mode can only be 3 or 4"); System.out.println("You entered: " + mode); throw new IOException(); } } public Tree getNodeTree(){ return this.nodeTree; } // assumes that the file exists already // TODO: tweak logic so that it builds the proper tree public void buildList() throws IOException { System.out.println("reading"); BufferedReader reader = new BufferedReader(new FileReader("dataset.txt")); String url; while((url = reader.readLine()) != null){ Document doc = Jsoup.connect(url).get(); String text = doc.body().text().toLowerCase(); String[] words = text.split("\\s+"); // splits by whitespace for (String word : words) { // HERE if (!word.isEmpty()) { if (nodeTree == null) { nodeTree = new Tree<>(new Node(word, url)); } else { Node node = nodeTree.search(new Node(word, null)); if (node != null) { node.insertReference(url); } else { nodeTree.insert(new Node(word, url)); } } } } } reader.close(); System.out.println("Finished reading through all URLs"); } // return a List ew ._. // TODO: Return the reference list of URLs public ArrayList search(String term) { System.out.println("Searching for " + term + " using data structure mode " + mode + "..."); // Search logic goes here ArrayList urls = new ArrayList<>(); // search logic depends on the data structure mode switch (mode) { case 1: // ArrayList mode for (Node node : nodeTree.getNode()) { if (node.getKeyword().equals(term)) { urls.addAll(node.getReferences()); } } break; case 2: // Sorted ArrayList mode for (Node node : nodeTree.getNodes()) { if (node.getKeyword().equals(term)) { urls.addAll(node.getReferences()); } else if (node.getKeyword().compareTo(term) > 0) { break; // nodes are sorted, so we can stop searching if we go past the target term } } break; case 3: // BST mode (TODO) Node bstNode = nodeTree.search(new Node(term, null)); if (bstNode != null) { urls.addAll(bstNode.getReferences()); } break; case 4: // AVL mode (TODO) Node avlNode = nodeTree.search(new Node(term, null)); if (avlNode != null) { urls.addAll(avlNode.getReferences()); } break; default: break; } return urls; } public static void main(String[] args) throws IOException{ Scanner input = new Scanner(System.in); System.out.println("Enter mode as in what data structure to use:"); System.out.println(" 1. Array List "); System.out.println(" 2. Sorted Array List"); int mode = input.nextInt(); System.out.println("Building Search Engine..."); SearchEngine engine = new SearchEngine(mode); String answer = "y"; while (answer.equals("y")) { input.nextLine(); // consume the remaining newline character System.out.print("Search (enter a term to query): "); String term = input.nextLine(); engine.search(term); System.out.print("Would you like to search another term (y/n)? "); answer = input.nextLine(); } input.close(); } }
---different class---
import java.util.ArrayList; import java.lang.Comparable; // For building the SearchEngine public class Node implements Comparable{ private String keyword; private ArrayList references; public Node(String keyword){ this.keyword = keyword; this.references = new ArrayList (); } public String getKeyword(){ return this.keyword; } public ArrayList getReferences(){ return this.references; } // // public int compareTo(Object o){ // if(o instanceof Node){ // Node other = (Node) o; // return this.keyword.compareTo(other.keyword); // } // return -1; // } public void insertReference(String website){ this.references.add(website); } public boolean equals (Object o) { if (o instanceof Node) { Node other = (Node) o; return this.keyword.equals(other.keyword); } else return false; } public String toString(){ return this.keyword + " " + this.references; } public int compareTo(Node o) { return this.keyword.compareTo(o.keyword); } }
------question------
Please fix the bold parts of these codes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
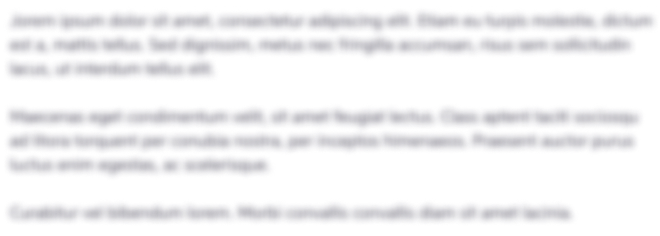
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started