Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import random from simpleai.search.models import SearchProblem from simpleai.search import breadth _ first, depth _ first, uniform _ cost , limited _ depth _ first, iterative
import random
from simpleai.search.models import SearchProblem
from simpleai.search import breadthfirst, depthfirst, uniformcost limiteddepthfirst, iterativelimiteddepthfirst, astar, greedy
class NQueensSearchProblem:
def initself N initialstateNone:
self.N N
if initialstate is None:
self.setstate
else:
self.state tuplemapint initialstate
superinittupleselfstate # Initial state is a tuple of queen positions
def actionsself state:
return i j for i in range self.N for j in range self.N
def resultself state, action:
i j action
newstate liststate
newstatei j
return tuplenewstate
def isgoalself state:
return self.countattackingpairsstate
def heuristicself state:
return self.countattackingpairsstate
def generaterandomstateself:
randomstate
for in rangeselfN:
strval random.randint self.N
randomstate strstrval
return randomstate
def setstateself:
stateanswer inputEnter for Manual Entrance, for Random State:"
if stateanswer :
self.state self.generaterandomstate
elif stateanswer :
statetemp inputenter state:
if self.isvalidstatetemp:
self.state statetemp
else:
self.state "wrong state"
else:
self.state "wrong entry"
def countattackingpairsself state:
if self.isvalidselfstate:
attackingpairs
for index, stateindexed in enumeratestate:
for index stateindexed in enumeratestate:
if index index:
coordinate index intstateindexed
coordinateindex intstateindexed
if abscoordinate coordinate abscoordinate coordinate or
coordinate coordinate or coordinate coordinate:
attackingpairs
return attackingpairs
return "Error"
def isvalidself state:
if lenstate self.N:
printThe length of the state string is not equal to N
return False
for i in rangelenstate:
try:
if not intstatei self.N:
printState string includes numbers greater than N or less than
return False
except ValueError:
return False
return True
def solvewithalgorithmself algorithm:
resultNone
algorithm None
if algorithmname bfs:
algorithm breadthfirst
elif algorithmname 'ucs':
algorithm uniformcost
elif algorithmname dfs:
algorithm depthfirst
elif algorithmname dls:
limit intinputEnter depth limit for DLS:
algorithm limiteddepthfirst
result limiteddepthfirstself depthlimitlimit
elif algorithmname 'ids':
algorithm iterativelimiteddepthfirst
elif algorithmname 'greedy':
algorithm greedy
elif algorithmname 'astar':
algorithm astar
if algorithm:
problem NQueensselfN self.state
if result is None:
result algorithmproblem graphsearchgraphsearch
printf
Algorithm: algorithmname
printfResulting State: resultstate
printfResulting Path: resultpath
printfCost: resultcost
if hasattrresult 'nodes':
printfViewer Statistics: Max Fringe Size: resultnodesmaxfringesize' Visited Nodes: resultnodesvisitednodes'
else:
printViewer statistics not available for this algorithm."
else:
printInvalid algorithm name. Please enter a valid algorithm."
if namemain:
N intinputEnter the size of the chessboard N:
algorithmname inputEnter the algorithm name BFS UCS, DFS DLS IDS, Greedy, A: lower
graphsearch inputUse graph search? yes or no: lower 'yes'
queensproblem NQueensN
queensproblem.solvewithalgorithmalgorithmname please add this code block value, mutate and crossover functions and adjust this according to the simpleai library. Add genetic search, hill climbing search and random restarts hill climbing search. P
Step by Step Solution
There are 3 Steps involved in it
Step: 1
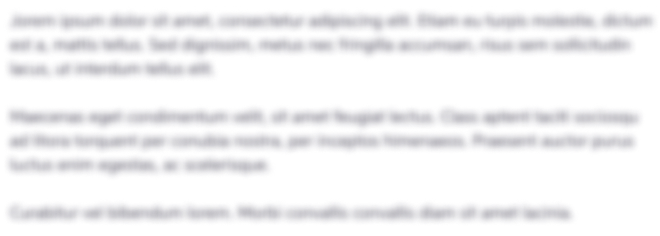
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started