Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import string import textwrap import re from collections import Counter from itertools import combinations import numpy as np english _ freq = { ' a
import string
import textwrap
import re
from collections import Counter
from itertools import combinations
import numpy as np
englishfreq
a:
b:
c:
d:
e:
f:
g:
h:
i:
j:
k:
l:
m:
n:
o:
p:
q:
r:
s:
t:
u:
v:
w:
x:
y:
z:
def onlylettersX caseNone:
Returns the string obtained from X by removing everything but the letters.
If case"upper" or case"lower", then the letters are all
converted to the same case.
X joinc for c in X if c in string.asciiletters
if lenX:
return None
if case is None:
return X
elif case "lower":
return Xlower
elif case "upper":
return Xupper
def stringforcodeblockX linewidth:
return
jointextwrapwrapX widthlinewidth
def addspacesX width linewidth:
oneline jointextwrapwrapX widthwidth
manylines stringforcodeblockoneline, linewidthlinewidth
return manylines
def shiftcharch shiftamt:
Shifts a specific character by shiftamt.
Example:
shiftcharY returns B
if ch in string.asciilowercase:
base a
elif ch in string.asciiuppercase:
base A
# It's not clear what shifting should mean in other cases
# so if the character is not upper or lowercase, we leave it unchanged
else:
return ch
return chrordchordbaseshiftamtordbase
def shiftstringX shiftamt:
Shifts all characters in X by the same amount.
return joinshiftcharch shiftamt for ch in X
def mutindcod d:
For letter frequency dictionaries d and d return the Mutual Index of Coincidence.
See Equation on page in Hoffstein, Pipher, Silverman.
s
for k in dkeys:
s dgetkdgetk
return s
def indcoX:
X onlylettersX case"upper"
ctr countsubstringsX
n sumctrvalues
return nnsumff for f in ctrvalues
def weavestringlist:
output joinjointup for tup in zipstringlist
# The rest is just to deal with the case of unequal string lengths
# We assume the only possibility is that the early strings are one character longer
lastlength lenstringlist
extra s for s in stringlist if lens lastlength
return output joinextra
def countsubstringsXn:
Returns a Python Counter object of all ngrams in X
if not X:
return
X onlylettersX
shifts Xi: for i in rangen
grams joinchrs for chrs in zipshifts
return Countergrams
def getfreqX case"lower":
Returns the proportion that each letter occurs in X
if case "lower":
letters string.asciilowercase
elif case "upper":
letters string.asciiuppercase
else:
raise ValueErrorcase should be 'upper' or 'lower'."
X onlylettersX casecase
n lenX
ctr countsubstringsX
output
for char in letters:
outputchar ctrcharn
return output
def kasiskidiffsY case"upper":
Y onlylettersY casecase
ctr countsubstringsY
trireps k for kv in ctritems if v
diffs
for tri in trireps:
starts mstart for m in refinditerftri Y
diffs.extendabsxy for xy in combinationsstarts
return nparraysorteddiffs
When we encrypt using the Vigenre cipher with a key length of for example we shift all the characters at integer positions modulo by the same amount, all characters at integer positions modulo by the same amount, and so on Assume X is the string we want to encrypt using the Vigenre cipher with a key length of Notice you can get all the characters in X at integer positions modulo for example using X::You can think of this as shorthand for X:lenX: which means, start at position go until reaching lenX go up by Test this out by setting X string.asciiuppercase and then printing X and X::
We need a way of reassembling" these strings. We can do that using the imported weave function. Evaluate the following code to get a sense for how it works. Here X should be the same as above. If you know list comprehension, try to recreate this list using list comprehension.
weaveX:: X:: X:: X:: X::
Step by Step Solution
There are 3 Steps involved in it
Step: 1
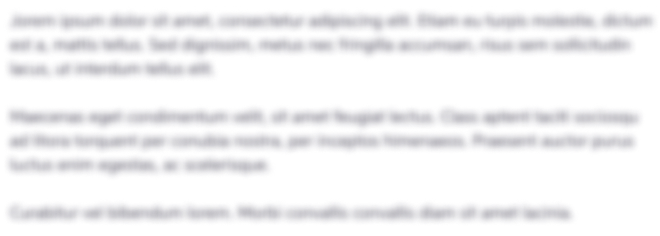
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started