Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import unittest from PythonLabCode.LabCode.Stack import Stack import PythonLabCode.LabCode.StackFullException as StackFullException import PythonLabCode.LabCode.StackEmptyException as StackEmptyException class MyTestCase ( unittest . TestCase ) : def test _
import unittest
from PythonLabCode.LabCode.Stack import Stack
import PythonLabCode.LabCode.StackFullException as StackFullException
import PythonLabCode.LabCode.StackEmptyException as StackEmptyException
class MyTestCaseunittestTestCase:
def testcreatestackself:
# ARRANGE
mystack Stack
# ACT
actual mystack.isempty
# ASSERT
self.assertTrueactual
def testisemptytrueself:
# ARRANGE
mystack Stack
# ACT
actual mystack.isempty
# ASSERT
self.assertTrueactual
def testisemptyfalseself:
# ARRANGE
mystack Stack
item "StackEmpty"
# ACT
mystack.pushitem
actual mystack.isempty
# ASSERT
self.assertFalseactual
def testisfullTrueself:
# ARRANGE
mystack Stack
item "StackItem"
# ACT
mystack.pushitem
actual mystack.isfull
# ASSERT
self.assertTrueactual
def testisfullFalseself:
# ARRANGE
mystack Stack
item "StackItem"
# ACT
mystack.pushitem
actual mystack.isfull
# ASSERT
self.assertFalseactual
def testpushstackself:
# ARRANGE
mystack Stack
item "StackItem"
expected "StackItem
# ACT
mystack.pushitem
mystack.pushitem
actual mystack.peek
# ASSERT
self.assertEqualexpected actual
def testpushfullstackself:
# ARRANGE
mystack Stack
item "StackItem"
# ACT
mystack.pushitem
# ASSERT
with self.assertRaisesStackFullException:
mystack.pushitem;
def testpopstackself:
# ARRANGE
mystack Stack
item "StackItem"
expected "StackItem
mystack.pushitem
# ACT
actual mystack.pop
# ASSERT
self.assertEqualexpected actual
def teststacksizezeroself:
# ARRANGE
mystack Stack
expected
# ACT
actual mystack.size
# ASSERT
self.assertEqualexpected actual
def teststacksizenonzeroself:
# ARRANGE
mystack Stack
item "StackItem"
expected
# ACT
mystack.pushitem
actual mystack.size
# ASSERT
self.assertEqualexpected actual
def testpopemptystackself:
# ARRANGE
mystack Stack
# ACT
# ASSERT
with self.assertRaisesStackEmptyException:
mystack.pop
def testpeekstackself:
# ARRANGE
mystack Stack
item "StackItem"
# ACT
mystack.pushitem
expected item
actual mystack.peek
# ASSERT
self.assertEqualexpected actual
def testpeekstacktwiceself:
# ARRANGE
mystack Stack
item "StackItem"
# ACT
mystack.pushitem
expected item
mystack.peek
actual mystack.peek
# ASSERT
self.assertEqualexpected actual
def testpeekemptystackself:
# ARRANGE
mystack Stack
# ACT
# ASSERT
with self.assertRaisesStackEmptyException:
mystack.peek
def testprintstackupself:
# ARRANGE
mystack Stack
item "StackItem"
expected "StackItem
StackItem
# ACT
mystack.pushitem
mystack.pushitem
actual mystack.printstackup
# ASSERT
self.assertEqualexpected actual
def testprintstackupemptystackself:
# ARRANGE
mystack Stack
# ACT
# ASSERT
with self.assertRaisesStackEmptyException:
mystack.printstackup
if namemain:
unittest.main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
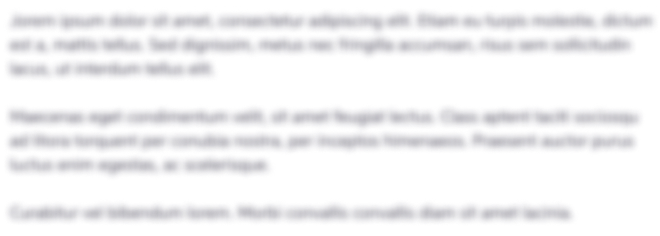
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started