Question
//IMPORTANT! Do NOT change any method headers import java.util.*; public class AllInOne { //begin class AllInOne /** * QUESTION 2: Worth 14 marks * Uses
//IMPORTANT! Do NOT change any method headers
import java.util.*; public class AllInOne { //begin class AllInOne
/** * QUESTION 2: Worth 14 marks * Uses class Rectangle * @param arr: an array of Rectangle objects * @param target: a Rectangle object * @return number of items in arr * that have the same area as that of target * return 0 if array is null */ public static int countTargetArea(Rectangle[] arr, Rectangle target) { return 0; //to be completed }
/** * QUESTION 3: Worth 14 marks * @param n: assumed to be more than 0 * @return highest digit in n * For example, * n = 18237 -> 8 * n = 911 -> 9 * n = 12321 -> 3 * NOTE: This method must be implemented recursively. * hint: n%10 gives the last digit, n/10 gives the rest of the number * and Math.max(a, b) gives higher of a and b */ public static int maxDigit(int n) { return 0; //to be completed }
/** * QUESTION 4: Worth 14 marks * @param list * @return number of times item occurs in list, 0 if it doesn't * return 0 if list is null */ public static int count(ArrayList list, int item) { return 0; //to be completed }
/** * Question 5: Worth 10 marks. D question * @param list * remove any positive items from the list. * for example, * if list passed = [5, 0, -3, 6, -7], after the execution it should be [0, -3, -7] */ public static void removePositives(ArrayList list) { //to be completed }
/** * Question 6: Worth 10 marks. HD question (try at the end) * @param start: starting index * @param nums * @param target * @return true if it is possible to (and false if not) choose a * group of some of the ints (starting at index start), * such that the group sums to the given * target with this additional constraint: If a value in the array * is chosen to be in the group, the value immediately * following it in the array must not be chosen. * (Solution must be recursive AND should not contain any loop) */ public static boolean groupNoAdjacent(int start, int[] nums, int target) { return false; //to be completed }
} //end class AllInOne (do not delete this closing bracket)
//questions 7 and 8 (custom-built linked-list) follow:
//do not modify methods add and get class MyLinkedList { private Node head;
//add an item to the end of the list public void add(int item) { Node temp = new Node(item, null); if(head == null) { head = temp; } else { Node cur = head; while(cur.getNext() != null) { cur = cur.getNext(); } cur.setNext(temp); } }
//return the value of supplied index (null if index invalid) public Integer get(int idx) { Node cur = head; for(int i=0; i < idx && cur != null; i++) { cur = cur.getNext(); } if(cur == null) return null; else return cur.getData(); }
/** * QUESTION 7: Worth 14 marks * @return number of odd items (NOT divisible by 2) in the list object. * return 0 if empty. * list should NOT BE MODIFIED * for example, * head -> 2 -> 5 -> 6 -> null: return 1 * head -> 2 -> 5 -> 6 -> -7 -> -5 -> null: return 3 * head -> 8: return 0 * head -> -5: return 1 * head -> null: return 0 */ public int countOdd() { return 0; //to be completed }
/** * QUESTION 8: Worth 10 marks. D Question * @return true if every item of the list is different from others, false otherwise. * for example, * if head -> 20 -> 80 -> 30 -> null: return true * if head -> 70 -> 10 -> 80 -> 10 -> null: return false * if head -> 50 -> null: return true * if head -> null: return true */ public boolean allDifferent() { return false; //to be completed } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
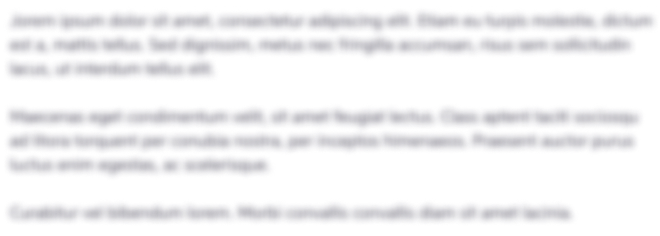
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started